Next.js
Error Handling and States in the Page Router
In this chapter, we will learn how to handle errors and states in a Next.js 13 application using the Page Router. Error management is crucial to ensure that applications work correctly in unexpected situations, such as when data requests fail or when users access non-existing routes.
Handling 404 Errors: Page Not Found
Next.js allows for easy customization of the 404 error page. If a user tries to access a non-existing route, Next.js displays a 404 page. You can create a custom page for this type of error.
Create a 404 Page
To handle 404 errors, simply create a 404.js
file in the pages/
folder. This file will represent the error page when users access a non-existing route.
javascript
With this setup, you can provide a more user-friendly experience when encountering a 404 error.
Handling 500 Errors: Server Error
500 errors indicate server issues. We can customize the 500 error page similarly to how we customize the 404 page, by creating a 500.js
file in the pages/
folder.
Create a 500 Page
javascript
Having a custom 500 error page is helpful for handling serious server errors in a more controlled way.
Handling Errors in Data Fetching
When working with SSR or SSG, data fetching requests might fail. It's important to handle these errors and display appropriate messages to users.
Handling Errors with getServerSideProps
In the following example, we show how to handle an error in a request within getServerSideProps
.
javascript
This pattern is useful when we need to ensure that the requested data is available and valid before rendering the page.
Handling Client-Side Errors
To handle errors on the client side, we can use try-catch
blocks and handle errors in React components. This is useful when fetching data on the client using Client-Side Rendering (CSR).
Client-Side Error Handling Example
javascript
This approach ensures that users receive clear feedback when something goes wrong, enhancing user experience.
Managing Loading States
In addition to error handling, it's important to manage loading states when data takes time to arrive. Displaying loading indicators improves user experience by providing information about the status of requests.
Loading State Example
javascript
Managing loading states is essential for users to understand that the application is working to retrieve the requested data.
Conclusion
Handling errors and states in the Page Router of Next.js 13 is fundamental to ensuring a smooth and professional user experience. Through custom error pages, handling failed requests, and clear loading states, we can significantly improve the reliability and responsiveness of our applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
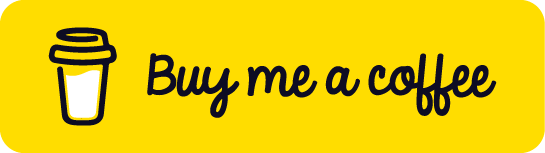
Chat with Chuck
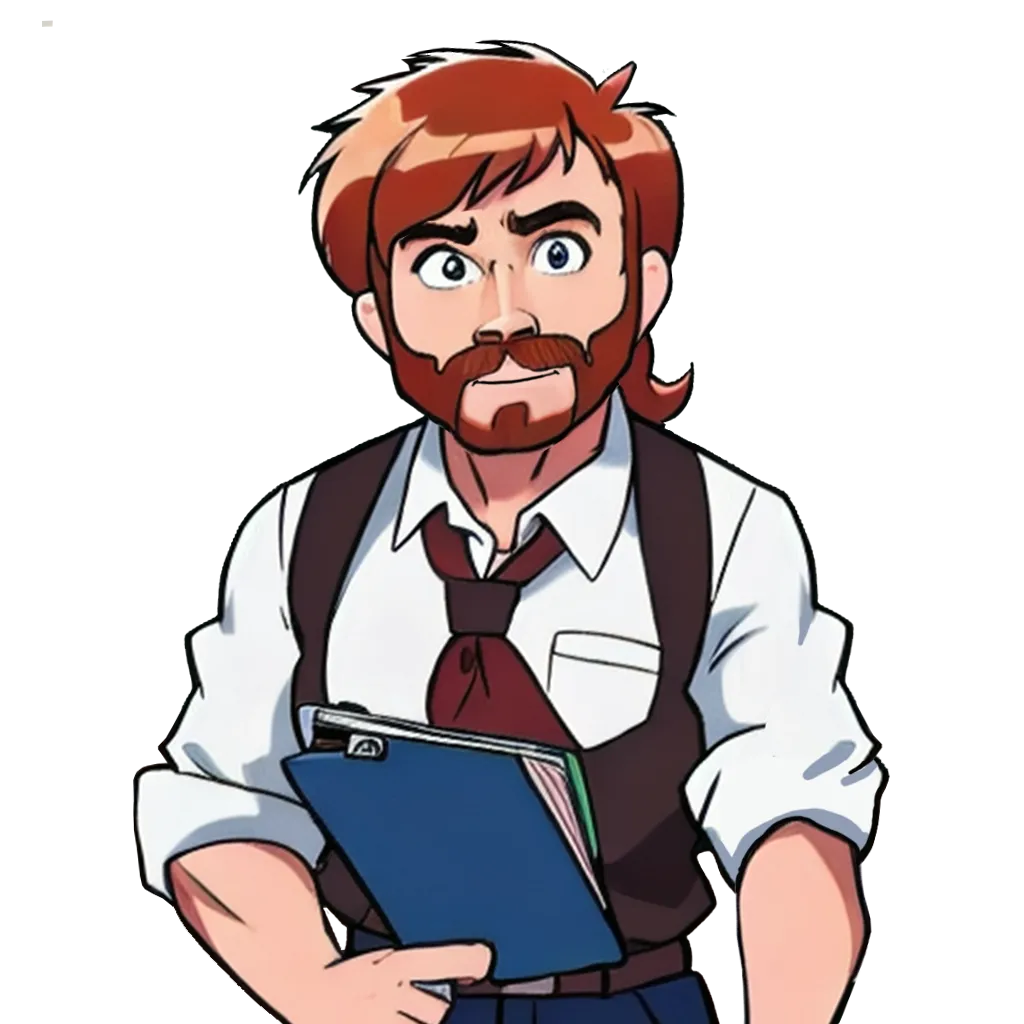
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13