Intermediate JavaScript
Advanced DOM Handling
The Document Object Model (DOM) is a programming interface that allows dynamic manipulation of a web page's elements. In this chapter, we will explore advanced techniques for working with the DOM efficiently and cleanly.
this image shows a DOM Diagram
Efficient Element Selection
The DOM offers several ways to select elements. It is essential to use efficient selectors, especially in applications with many elements.
javascript
Creation and Deletion of Elements
For manipulating the DOM, it is common to create and delete elements in real-time, especially in interactive applications.
javascript
To remove an element, we can use the removeChild
method or remove
directly on the element.
javascript
Event Delegation
Event delegation is a technique that allows assigning a single event handler to a container instead of each individual element, improving performance.
javascript
Advanced Style Manipulation
It is possible to change the styles of elements directly from JavaScript or manipulate classes to achieve more manageable style changes.
javascript
Form Handling and Validation
JavaScript allows handling forms and performing validations in the DOM before sending data to the server.
javascript
Performance Optimization in DOM Manipulation
To improve performance, it is advisable to reduce the number of accesses and manipulations to the DOM.
Document Fragments
A DocumentFragment
allows making changes to the DOM efficiently, applying multiple changes before inserting them into the document.
javascript
Intersection Observer
The IntersectionObserver
allows observing elements when they enter or leave the view, which is useful for implementing effects like lazy loading or animations.
javascript
Conclusion
Advanced DOM handling in JavaScript allows building more dynamic and efficient user interfaces. By understanding how to select, create, and manipulate elements efficiently, you can improve the performance and usability of your applications.
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps
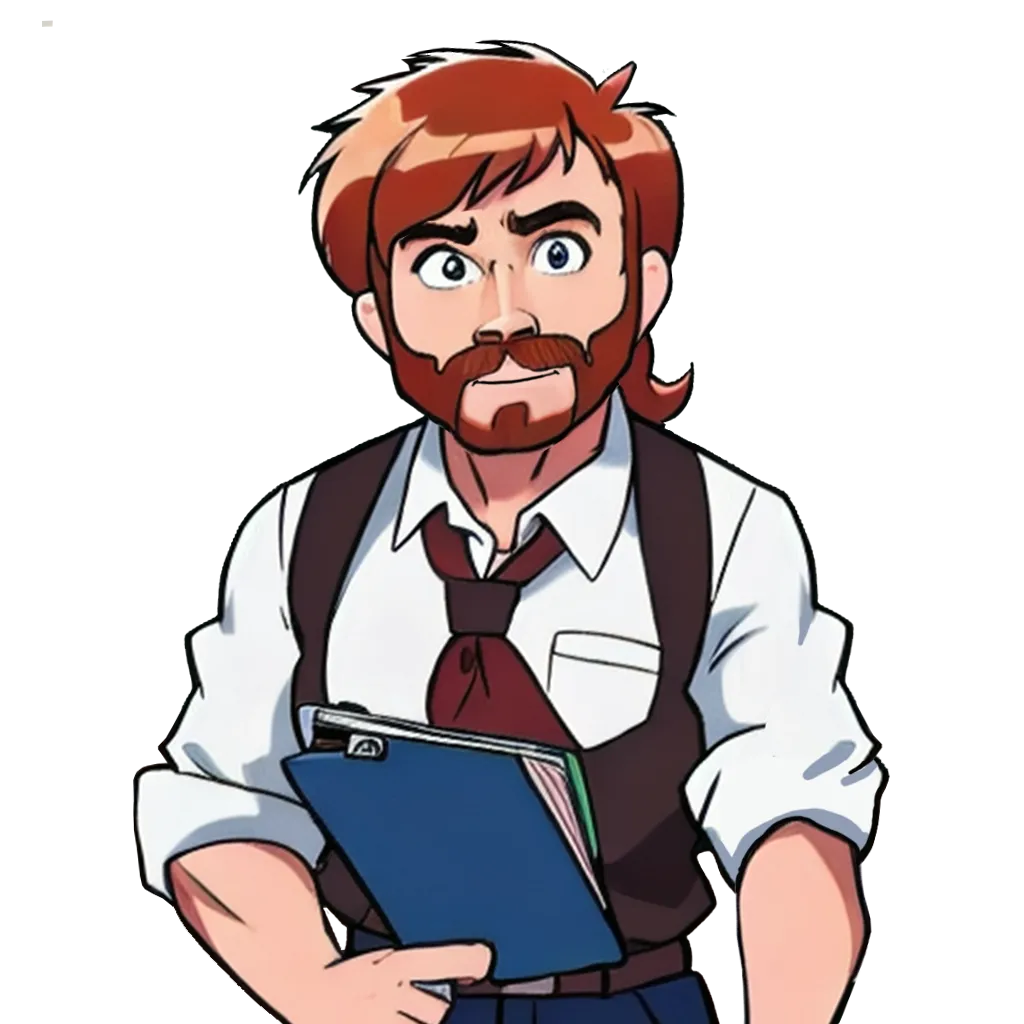