Intermediate JavaScript
Design Patterns in JavaScript
Design patterns are proven solutions to common problems in software development. In JavaScript, these patterns help us structure code in a modular, reusable, and maintainable way. In this chapter, we will explore some of the most common design patterns in JavaScript.
Javascript design pattern
Module Pattern
The module pattern allows for encapsulating code in a function or an object, hiding internal details and exposing only what is necessary.
javascript
Singleton Pattern
The Singleton pattern ensures that only one instance of a class or an object exists throughout the application.
javascript
Observer Pattern
The observer pattern allows an object (the subject) to maintain a list of dependents (observers) and notify them of changes.
javascript
Factory Pattern
The Factory pattern is useful for creating instances of classes without exposing the creation logic to the client.
javascript
Decorator Pattern
The decorator pattern allows for adding additional functionality to an object dynamically, without modifying its original structure.
javascript
Strategy Pattern
The strategy pattern allows defining a family of algorithms and making the algorithms interchangeable.
javascript
Conclusion
Design patterns in JavaScript offer elegant solutions to common problems and promote a modular and reusable architecture. By applying patterns like Singleton, Observer, and Decorator, you can improve the scalability and flexibility of your code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
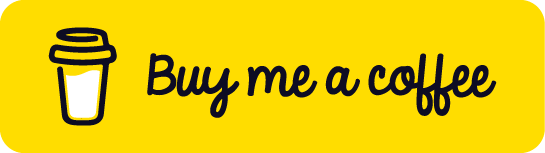
Chat with Chuck
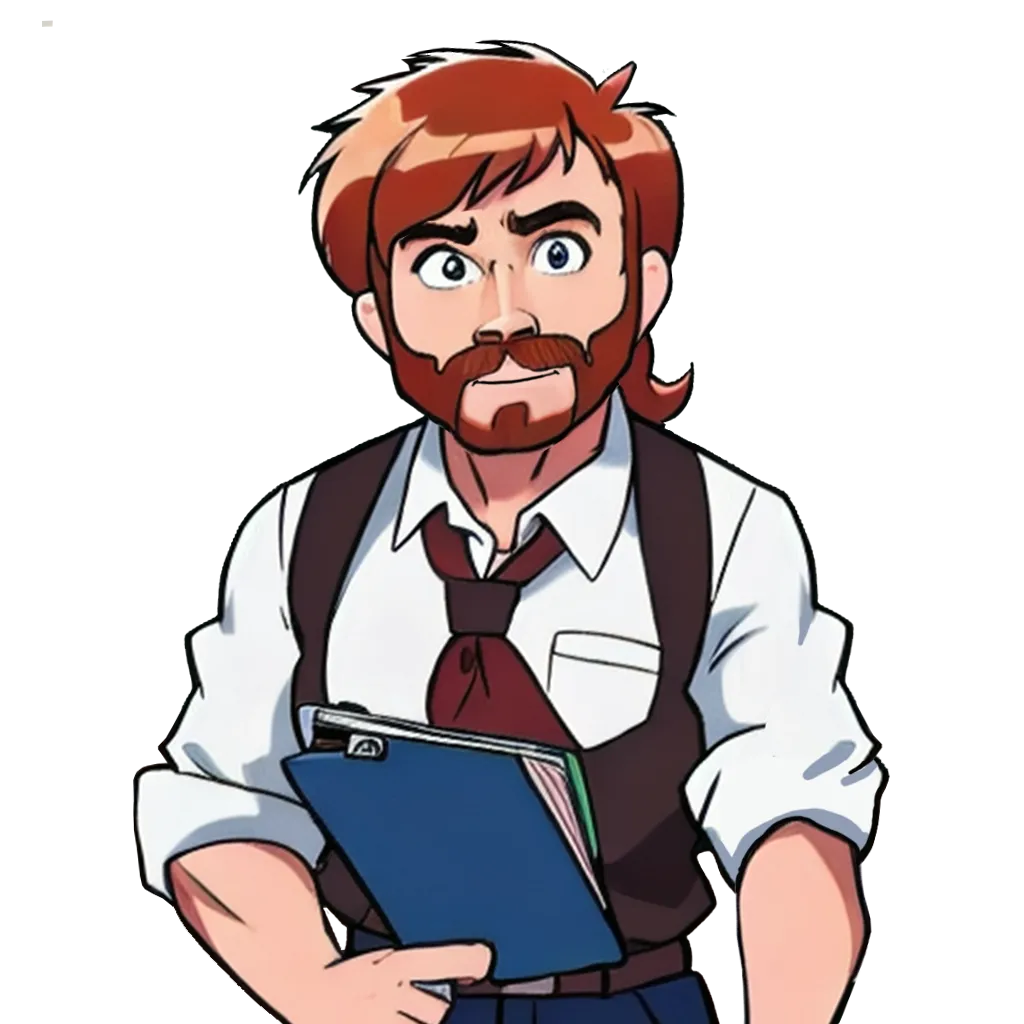
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps