Intermediate JavaScript
Introduction to WebAssembly
WebAssembly is a technology that allows low-level code to run in the browser, offering performance close to that of native code. It is ideal for tasks that require high efficiency, such as image processing, file compression, and gaming applications. In this chapter, we'll explore the basics of WebAssembly and its integration with JavaScript.
integration with webassembly
What is WebAssembly?
WebAssembly (Wasm) is a binary code format that can run in most modern browsers. It is designed to be fast and efficient, allowing developers to write code in other languages (such as C, C++, or Rust) and run it in the browser alongside JavaScript.
Benefits of WebAssembly
Some key benefits of WebAssembly include:
- Performance: Allows faster execution of code than JavaScript in intensive tasks.
- Portability: Wasm is operating system independent and works in any modern browser.
- Interoperability: WebAssembly and JavaScript can communicate, allowing both to be combined in applications.
Example of a Simple WebAssembly Module
To work with WebAssembly, we generally start with a file written in a compatible language (e.g., C). This code is then compiled to WebAssembly.
Code in C
c
Compile to WebAssembly
To compile the example.c
file to WebAssembly, we can use tools like Emscripten
, which converts C/C++ code to WebAssembly.
bash
Load WebAssembly in JavaScript
Once we have the .wasm
file, we can load it in the browser and use it alongside JavaScript.
javascript
Communication between JavaScript and WebAssembly
JavaScript can pass data to WebAssembly and receive results back, allowing the logic of both languages to be combined.
Example of a Function that Receives Arguments
javascript
Tools for Working with WebAssembly
There are several tools to facilitate development in WebAssembly:
- Emscripten: Compiles C/C++ to WebAssembly.
- AssemblyScript: Allows writing WebAssembly directly in TypeScript.
- Rust: Offers excellent support for WebAssembly.
Use Cases for WebAssembly
WebAssembly is ideal for applications that require performance, such as:
- Games in the browser.
- Image editing or multimedia processing.
- Scientific calculations and complex simulations.
Conclusion
WebAssembly opens a new dimension for web development, allowing low-level code to run alongside JavaScript. By learning to integrate and use WebAssembly, you can create faster and more powerful applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
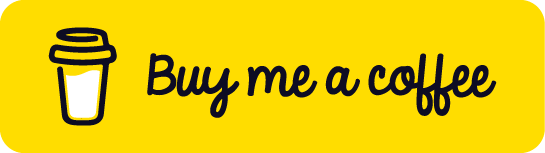
Chat with Chuck
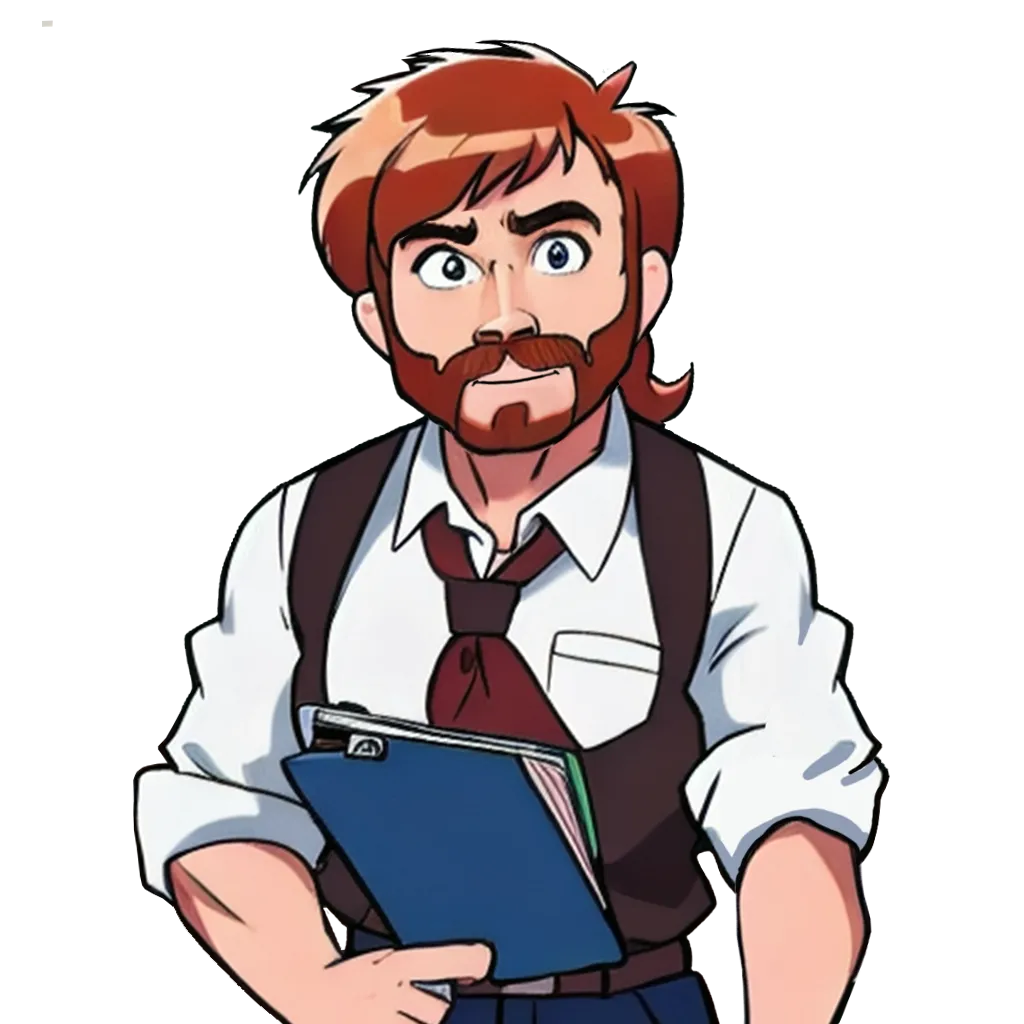
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps