Intermediate JavaScript
Advanced Testing with Mocha, Chai, and Jest
Advanced testing in JavaScript is essential to ensure that the code works as expected and is reliable. Mocha, Chai, and Jest are three popular tools in the JavaScript ecosystem that allow you to write, structure, and execute tests efficiently. In this chapter, we will explore how to use them to implement advanced tests.
this image shows different types of testing frameworks
Mocha: A Flexible Testing Framework
Mocha is a testing framework that allows you to write and run tests in JavaScript, providing a clear and flexible structure.
Installing Mocha
To install Mocha in your project, use npm:
bash
Writing Tests with Mocha
Tests in Mocha are organized into describe
and it
blocks, which help structure test cases.
javascript
Chai: Assertion Library
Chai is a library that provides more expressive assertions for our tests, compatible with Mocha and other frameworks.
Installing Chai
To install Chai in the project:
bash
Using expect
in Chai
Chai offers various assertion styles, such as expect
, which provides a readable and expressive syntax.
javascript
Jest: An All-in-One Framework
Jest is a complete testing framework that includes its own test runner and assertion library, popular in React development.
Installing Jest
Install Jest in your project:
bash
Writing Tests with Jest
In Jest, you can write tests without needing additional configurations, using the test
and expect
methods.
javascript
Asynchronous Testing
Both Mocha and Jest allow working with asynchronous tests to handle operations like network requests or timers.
Asynchronous Tests in Mocha
In Mocha, you can work with asynchronous functions using async/await
.
javascript
Asynchronous Tests in Jest
Jest also supports asynchronous testing using async/await
.
javascript
Spies and Mocks with Jest
Jest makes it easy to create mocked functions and spies for testing dependent functions.
javascript
Conclusion
Mocha, Chai, and Jest offer powerful tools for advanced testing in JavaScript. From Mocha's flexible structure and Chai's expressive assertions, to Jest's all-in-one mocks and tests, these tools will help you write reliable and maintainable code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
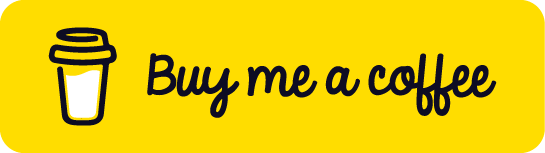
Chat with Chuck
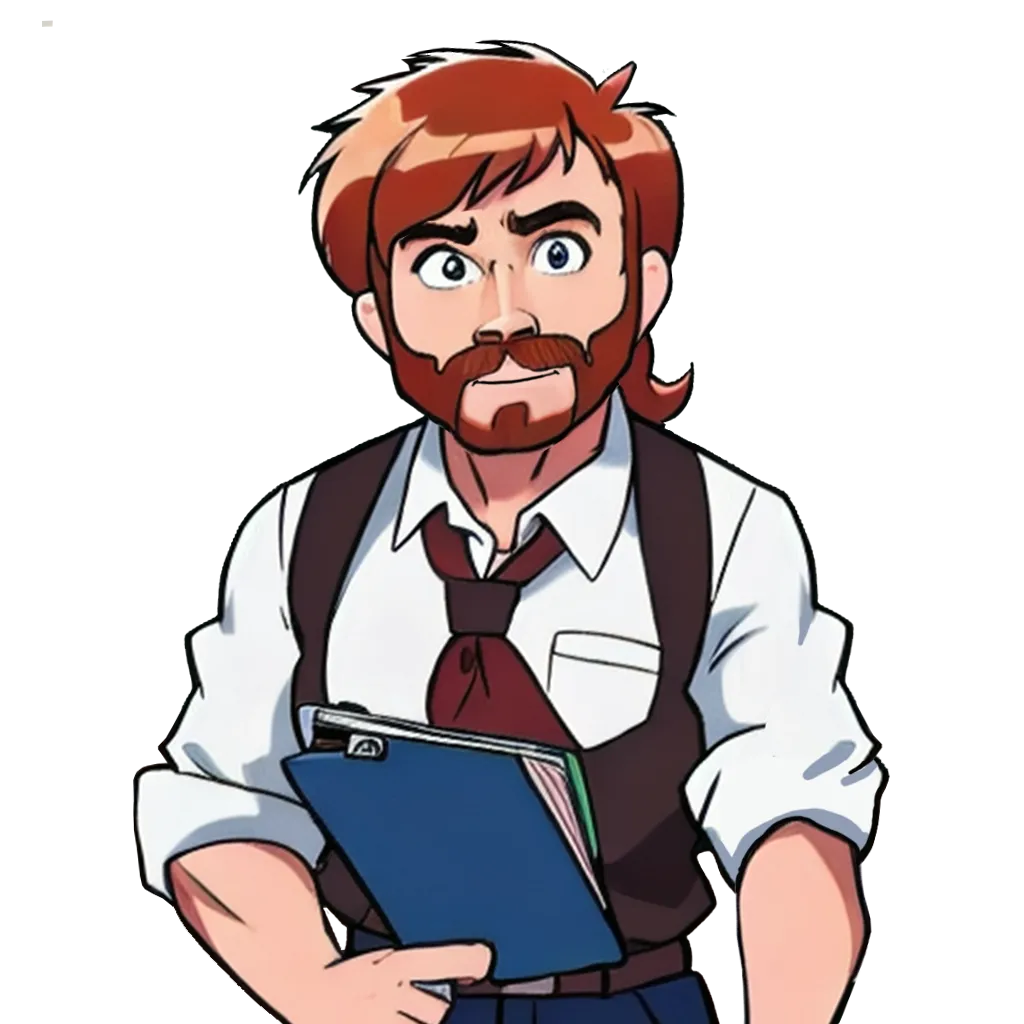
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps