Intermediate JavaScript
Reactive Programming with RxJS
Reactive programming is a paradigm that allows for handling data flows and events asynchronously and declaratively. In JavaScript, the RxJS library facilitates the implementation of this approach through observables and operators.
rxjs logo
What is RxJS?
RxJS (Reactive Extensions for JavaScript) is a library that allows you to work with reactive programming in JavaScript, providing a collection of operators to transform and manage data flows. With RxJS, you can manage events, network requests, and other data flows more controlled and declaratively.
Observables
Observables are the foundation of RxJS. An observable represents a data source that can emit values over time.
javascript
Transformation Operators
RxJS operators allow transforming the data emitted by an observable. One of the most common operators is map
.
javascript
Filtering Operators
Filtering operators, such as filter
, allow emitting only the values that meet a specific condition.
javascript
Combination of Observables
RxJS allows combining multiple observables using operators like merge
and concat
.
javascript
Error Handling in Observables
The catchError
operator allows handling errors in observables and providing a recovery strategy.
javascript
Creating Custom Observables
RxJS allows creating custom observables to represent any flow of data or events.
javascript
Conclusion
Reactive programming and the use of RxJS allow handling data flows flexibly and controlled, ideal for real-time applications and complex event management. By mastering observables and operators, you can create more interactive and efficient applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
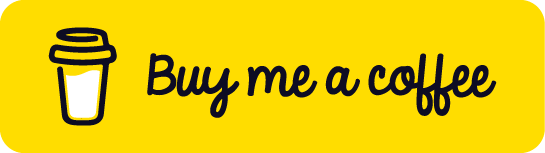
Chat with Chuck
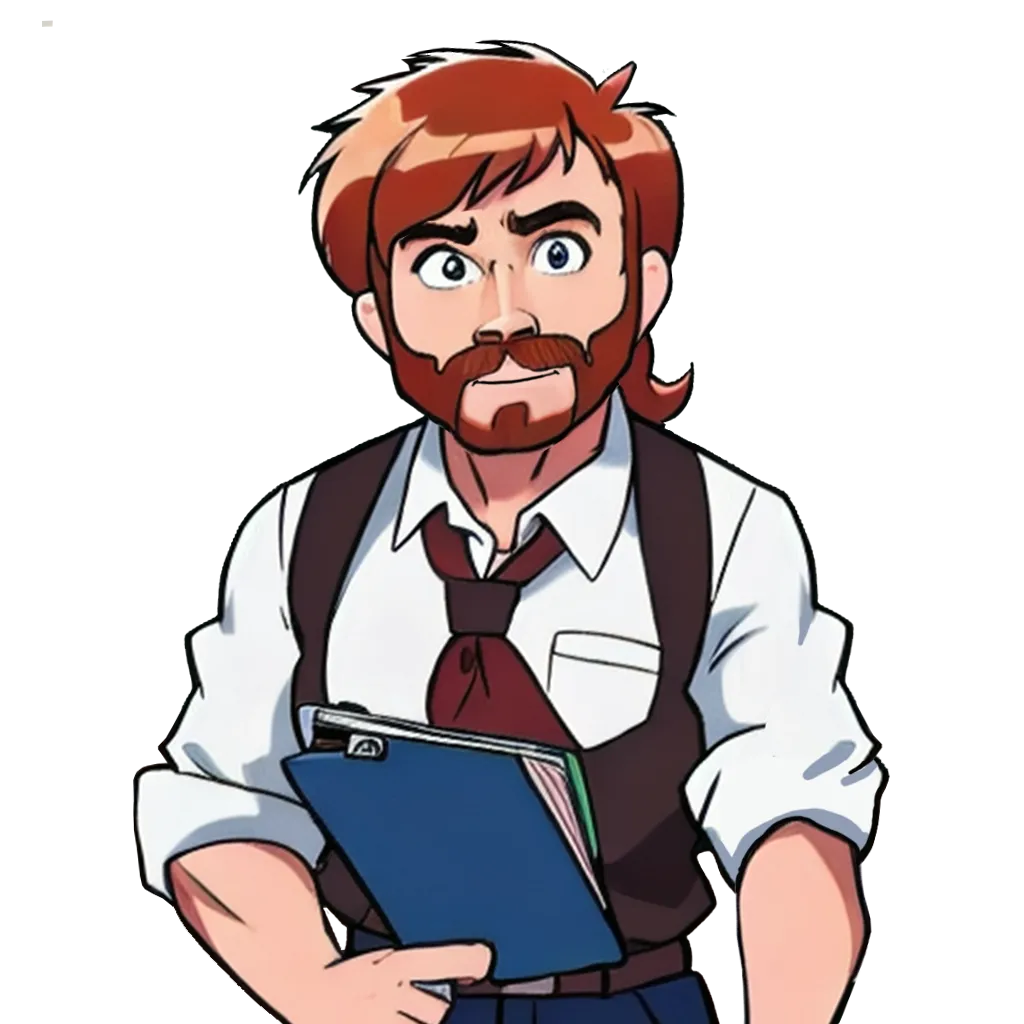
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps