Intermediate JavaScript
Advanced Array Manipulation
Javascript Arrays
Arrays are fundamental data structures in JavaScript, and offer a wide range of methods for advanced manipulation. In this chapter, we will explore how to use array methods to efficiently transform, filter, and reduce data.
Using map
for Transformations
The map
method allows you to transform each element of an array into a new array. It's useful when you need to apply a function to each element.
javascript
Filtering Elements with filter
The filter
method creates a new array that contains only the elements that meet a specific condition.
javascript
Reducing Arrays with reduce
The reduce
method accumulates all elements of an array into a single value, which is useful for computations like sums or products.
javascript
Finding Elements with find
The find
method returns the first element in an array that meets a condition, without affecting the others.
javascript
Checking Conditions with some
and every
The some
method checks if at least one element in the array meets a condition, while every
checks if all elements meet a condition.
javascript
Sorting Elements with sort
The sort
method allows you to sort the elements of an array. It's important to understand how to use it correctly to avoid issues.
javascript
Precautions When Using sort
The sort
method modifies the original array. If you need to keep the original array, be sure to make a copy before sorting.
javascript
Combining Methods for Complex Transformations
By combining multiple array methods, we can perform complex operations very efficiently.
javascript
Conclusion
array cheatsheet
Advanced array manipulation is fundamental to working efficiently with data in JavaScript. By mastering methods like map
, filter
, reduce
, and others, you can create complex data transformations with ease.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
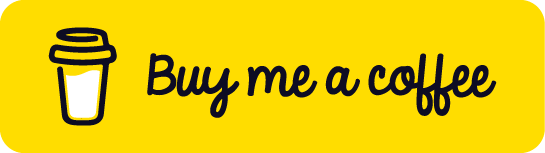
Chat with Chuck
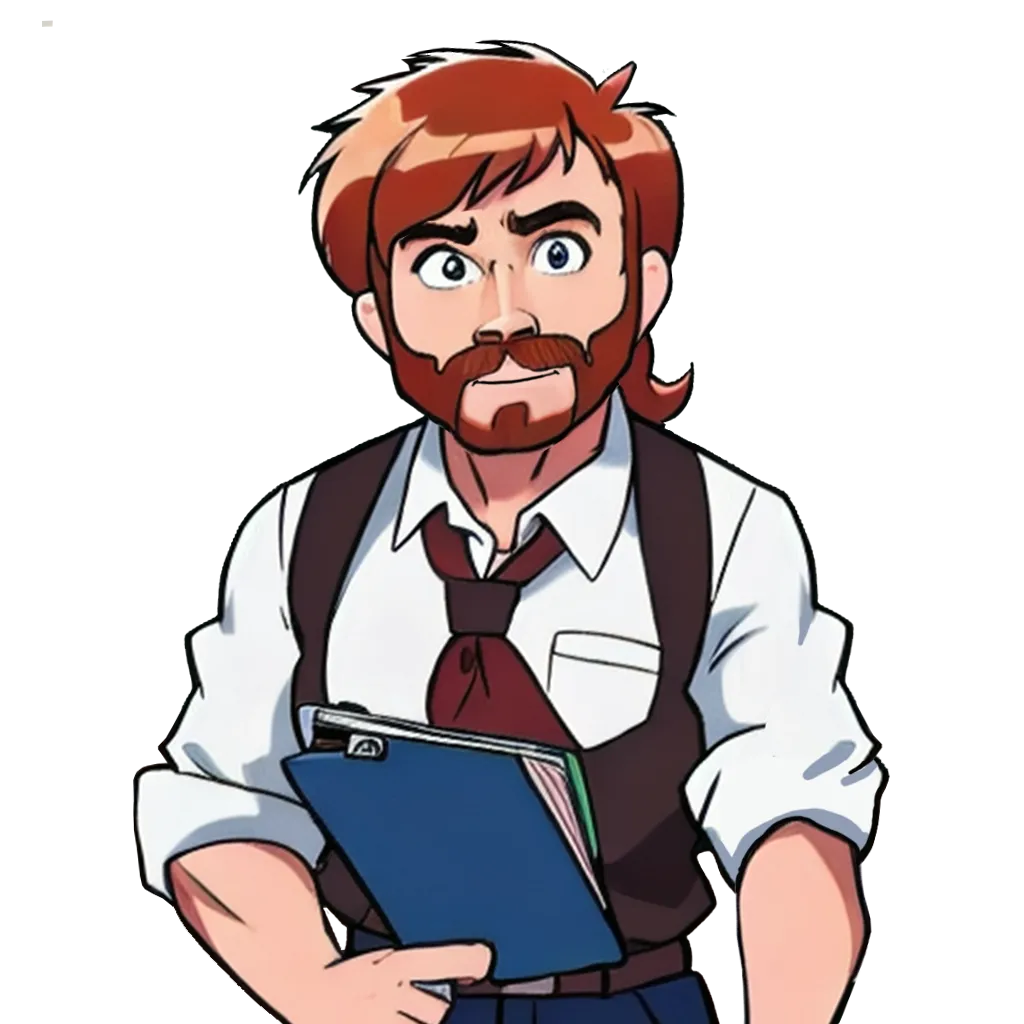
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps