Intermediate JavaScript
Prototype Manipulation and Inheritance
JavaScript is a prototype-based language, which allows objects to inherit properties and methods from other objects. In this chapter, we will explore the prototype system in JavaScript and how it is used to implement inheritance.
Prototype in JavaScript
Prototypes in JavaScript
Each object in JavaScript has a prototype, which is another object from which it inherits properties and methods. This allows objects to share functionality without duplicating code.
javascript
Constructor and prototype
Constructors are functions that allow creating multiple instances of an object with similar properties. Using the prototype
property, we can define methods that will be shared among all instances.
javascript
Inheritance in JavaScript
We can create a chain of prototypes to implement inheritance, allowing an object to inherit properties from another.
javascript
Classes and Inheritance Syntax
With ES6, JavaScript introduced a clearer syntax for working with inheritance through the class
keyword.
javascript
Method Overriding
When a child class needs different behavior, it can override an inherited method.
javascript
instanceof
and isPrototypeOf
JavaScript offers instanceof
and isPrototypeOf
to check inheritance relationships.
javascript
Conclusion
Prototype manipulation and inheritance in JavaScript are key concepts for building modular and reusable applications. Understanding how the prototype system works allows you to write more flexible code and leverage JavaScript's inheritance model.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
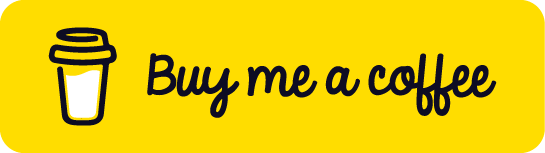
Chat with Chuck
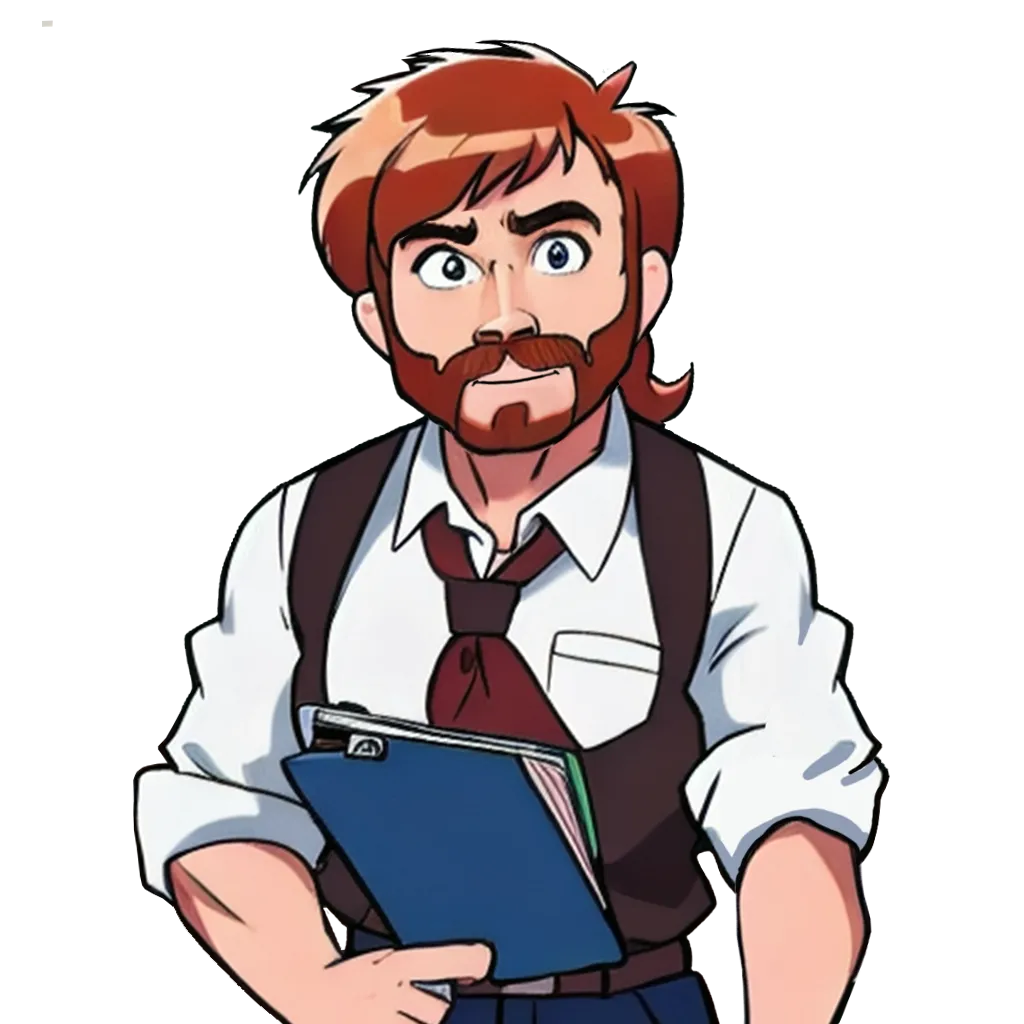
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps