Intermediate JavaScript
Error Handling in JavaScript
Error handling is an essential part of programming to ensure that our applications behave robustly in unexpected situations. In this chapter, we will explore techniques to efficiently handle errors in JavaScript.
Using try/catch
try/catch diagram
The try/catch
block allows capturing errors in the code without interrupting the general flow of the program.
javascript
Throwing Errors with throw
We can use throw
to generate custom errors when specific conditions occur in our code.
javascript
finally
for Additional Executions
The finally
block always executes, regardless of whether an error occurs or not. This is useful for freeing resources or performing final tasks.
javascript
Error Handling in Asynchronous Functions
In asynchronous functions, errors can be handled with try/catch
around await
blocks to capture Promise errors.
javascript
Error Handling in Promises with catch
When working with Promises, catch
is essential to handle errors without breaking the execution chain.
javascript
Custom Errors and Inheritance from Error
We can create custom errors by inheriting from the Error
class, providing specific messages or additional structures.
javascript
Error Logging and Monitoring Tools
For production applications, it is essential to log errors and use monitoring tools like Sentry or LogRocket.
javascript
Conclusion
Error handling in JavaScript is fundamental to creating robust applications and avoiding unexpected failures.
With the use of try/catch
, custom errors, and monitoring tools, you can effectively identify and handle errors.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
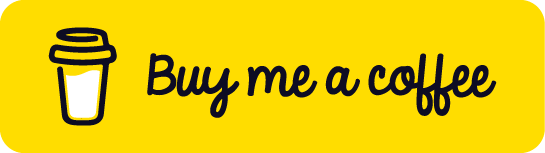
Chat with Chuck
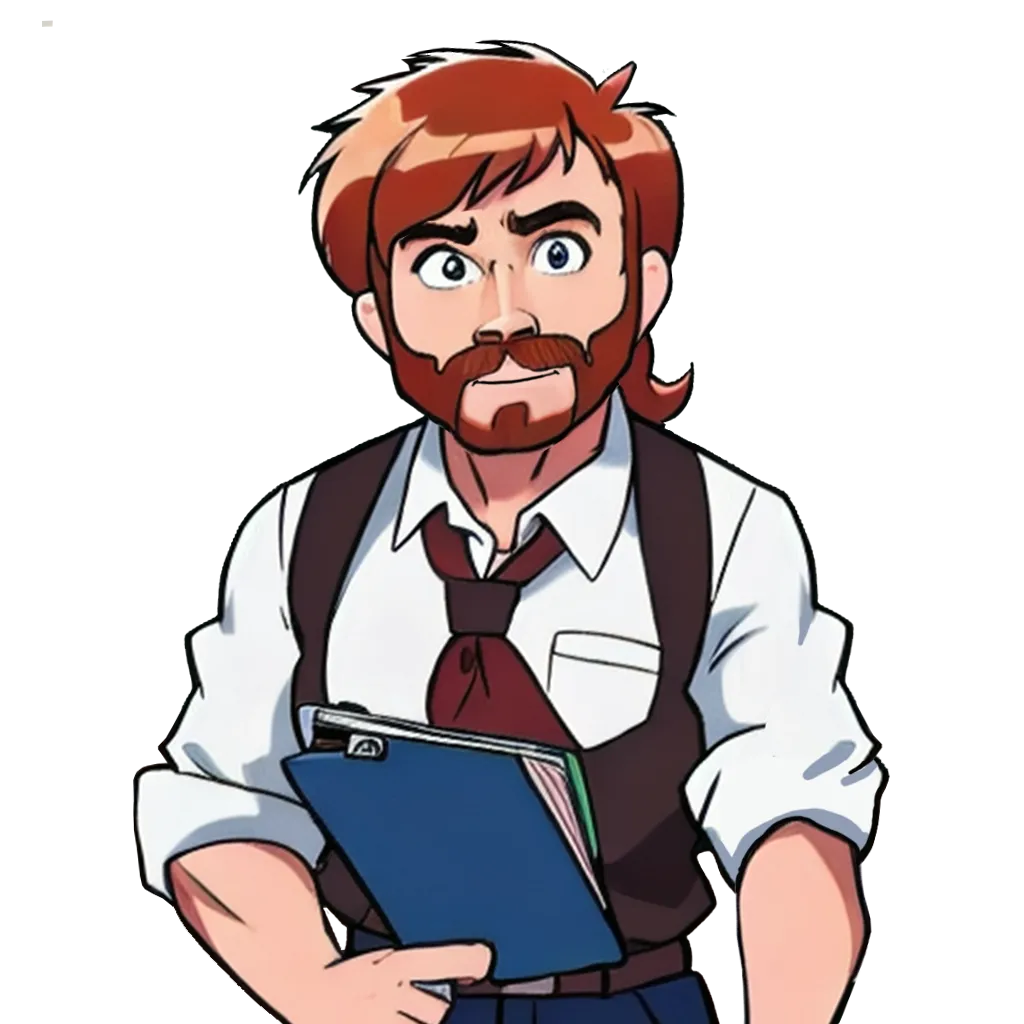
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps