Intermediate JavaScript
Scope, Context, and `this` in Depth
Scope, context, and the this
keyword are fundamental concepts in JavaScript, and understanding how they work is essential for writing efficient, error-free code. In this chapter, we'll explore these topics in depth.
Scope in JavaScript
Scope determines the accessibility of variables. In JavaScript, there are three main types of scope: global, function, and block.
javascript
Block Scope
Block scope allows variables to be declared that are only available within a block of code, such as a loop or condition.
javascript
Context and the this
Keyword
Context refers to how a function is called and the value of this
within that function. this
can change depending on how the function is invoked.
javascript
Changing Context with call
, apply
, and bind
JavaScript allows you to change a function's context using call
, apply
, and bind
. These techniques are useful for reusing functions in different contexts.
call
call
invokes a function and allows you to specify the value of this
.
javascript
apply
apply
is similar to call
, but it passes the arguments as an array.
javascript
bind
bind
creates a new function with the specified context, without executing it immediately.
javascript
this
in Arrow Functions
Arrow functions do not have their own this
value. Instead, this
in an arrow function is inherited from the context in which it is defined.
javascript
Combined Examples of Scope and Context
The combination of scope and context is key in JavaScript, especially when handling nested functions and callbacks.
javascript
Conclusion
Understanding scope and context in JavaScript is essential to avoid common errors and manipulate this
effectively. With practices like call
, apply
, and bind
, you can control the context of your functions and build more flexible code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
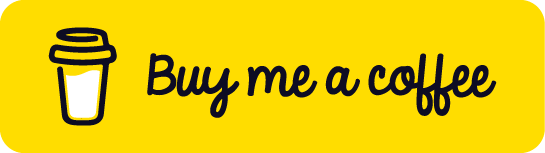
Chat with Chuck
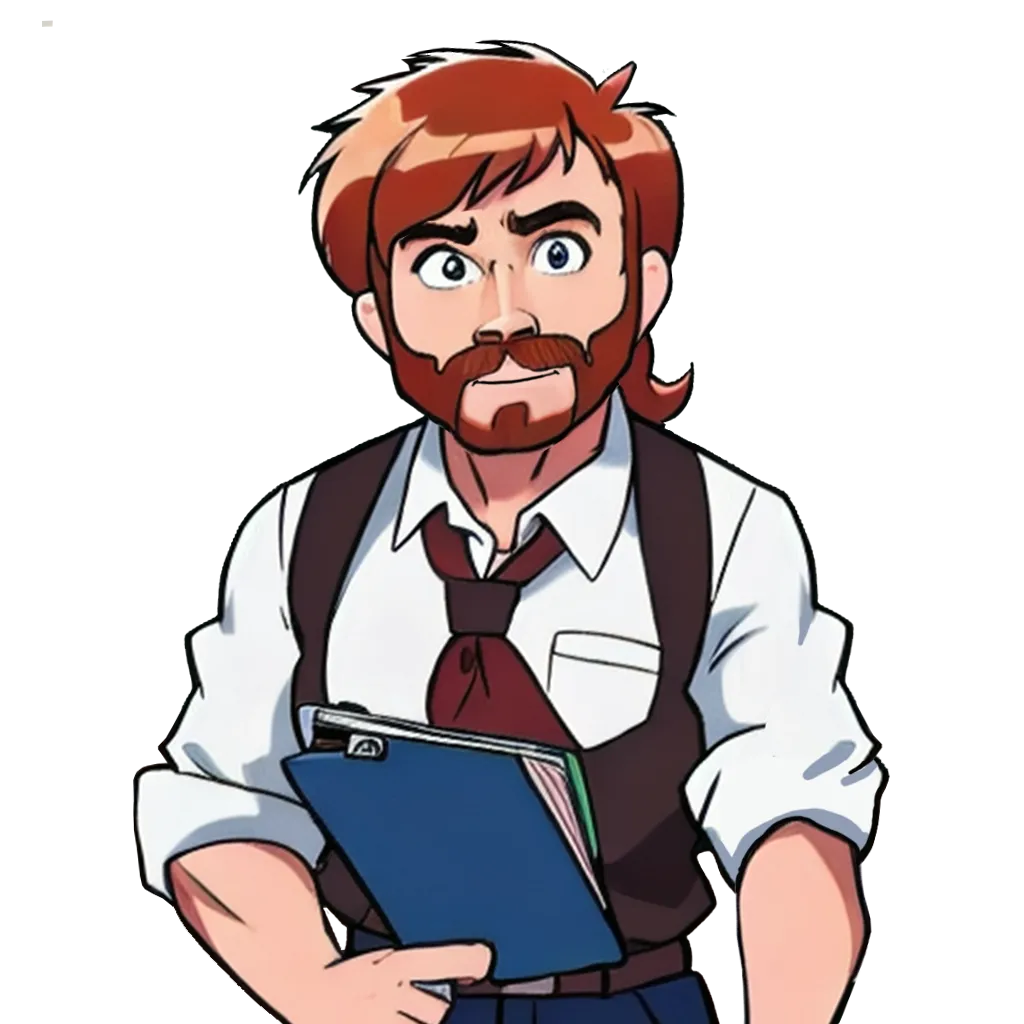
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps