Intermediate JavaScript
Advanced Debugging and Development Tools
Debugging is an essential skill for identifying and resolving code issues. In this chapter, we'll explore advanced debugging techniques and how to use development tools to debug and improve code quality.
Using console
for Advanced Debugging
While console.log
is the most common debugging tool, there are other console
methods that can provide more detailed information.
console.error
: To display error messages in red in the console.console.warn
: For warnings, ideal for highlighting minor issues.console.table
: To display tabular data in a structured way.
javascript
Using Breakpoints in the Browser
Breakpoints allow you to pause code execution at a specific line, helping you analyze the state of variables at that moment.
Types of Breakpoints
- Line breakpoint: Pauses at a specific line of code.
- Conditional breakpoint: Pauses when a specific condition is met.
- DOM Breakpoint: Pauses when a specific element changes in the DOM.
- XHR Breakpoint: Pauses when a specific HTTP request is made.
How to Use a Breakpoint
- Open the development tools in your browser (usually F12 or right-click -> Inspect).
- Go to the "Sources" tab.
- Click on the line number where you want to pause.
javascript
Call Stack and Stack Trace
The Call Stack shows the trace of function calls that have led to the current line, which is useful for tracking code flow.
javascript
Using Watch and Scope in Development Tools
The Watch
section allows you to observe specific variables and see how they change during program execution, while Scope
shows the scope and values of variables in each context.
- Watch: Add custom expressions to observe variable changes.
- Scope: Explore the current context and available variables.
javascript
Debugging with debugger
Using the debugger
keyword in your code allows you to set a breakpoint programmatically.
javascript
Profiler: Analyze Performance
The Profiler allows you to measure code performance and detect bottlenecks. It generates an execution profile that shows the time each function takes.
How to Use the Profiler
- Open the development tools and go to the "Performance" tab.
- Start recording and run the code you want to analyze.
- Stop recording and review the results to identify time-consuming functions.
Debugging Tools in Node.js
Node.js also has built-in debugging tools.
Using node inspect
You can debug a Node.js script using node inspect
to enter interactive debugging mode.
bash
Integration with Visual Studio Code
Visual Studio Code allows easy debugging of JavaScript, both in the browser and Node.js, with breakpoints, watch
, and profiling tools.
Debugging Setup in VSCode
- Open your JavaScript file in Visual Studio Code.
- Go to the "Run and Debug" tab and select "JavaScript Debug Terminal."
- Set breakpoints and observe step-by-step execution.
javascript
Debugging Promises and Asynchronous Code
Debugging asynchronous code can be challenging. Development tools allow you to view pending promises and the state of each one.
javascript
Conclusion
Advanced debugging in JavaScript requires mastering various techniques and tools to efficiently identify and solve issues.
By learning to use console
, breakpoints, the Profiler, and Node.js debugging tools, you can improve code quality and performance.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
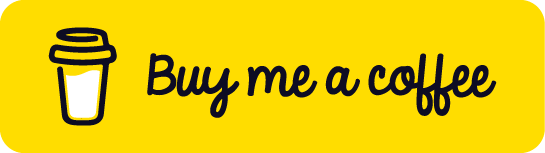
Chat with Chuck
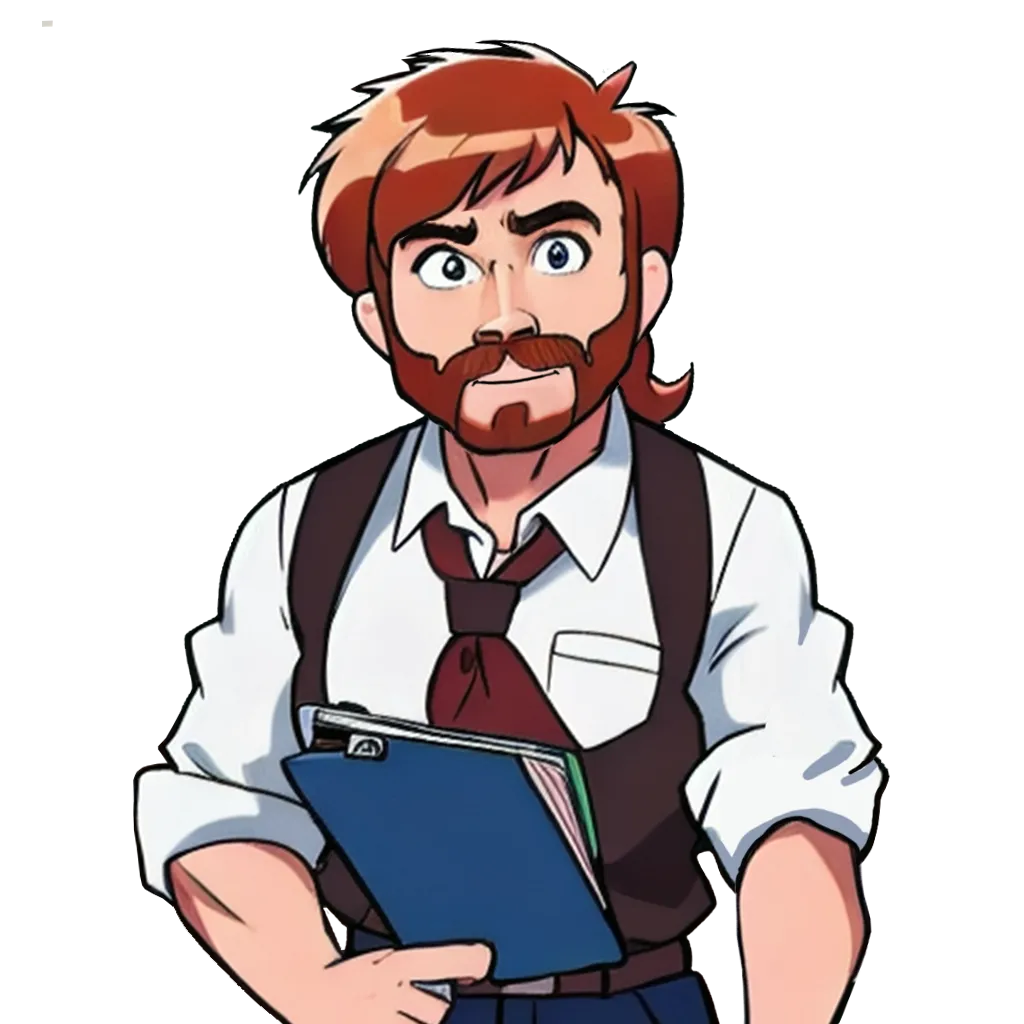
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps