Intermediate JavaScript
Conclusions and Next Steps
desktop-image:
chucks-js-banner-2.png
Congratulations on completing the Intermediate JavaScript course! Throughout this course, we have explored various advanced topics that strengthen your understanding and skills in JavaScript. In this final chapter, we summarize the key concepts and suggest some steps to continue your learning.
Summary of Key Concepts
-
Destructuring and Spread Operator: You learned to efficiently manipulate and assign values from objects and arrays using destructuring and the spread operator.
-
Advanced Array Manipulation: We explored advanced functions like
map
,filter
,reduce
, and other methods to work with arrays declaratively and functionally. -
Closures and Scope: You understood how closures and the variable scope work in JavaScript, which is essential for data management and encapsulation.
-
Functional Programming: We introduced concepts of functional programming, including pure functions and immutability, which are useful for writing predictable and side-effect-free code.
-
Advanced DOM Manipulation: You learned techniques to efficiently manipulate the DOM using events, delegation, and performance improvements.
-
Advanced Promises and Async/Await: You mastered the use of Promises and the
async/await
syntax to work with asynchronous operations in a structured and efficient manner. -
Error Handling: We explored how to handle errors in JavaScript using
try/catch
and control flow structures. -
Modules and Code Organization: We delved into the import and export of modules to organize and reuse code in larger-scale projects.
-
Classes and Inheritance: You learned about classes, inheritance, and object-oriented design patterns that improve code structure and modularity.
-
Web APIs and Reactive Programming: We explored advanced Web APIs and the use of RxJS to implement reactive programming, ideal for complex data flows and events.
-
Advanced Testing and Debugging: You learned to use Mocha, Chai, and Jest for advanced testing, as well as debugging techniques to efficiently identify and resolve errors.
-
Best Practices and Code Styles: We concluded with best practices for writing clear, maintainable, and efficient code, improving project readability and quality.
Next Steps
Delve into Advanced JavaScript
There are several advanced topics you can explore to continue developing your JavaScript skills:
- Design Patterns: Learning more design patterns can help you write more flexible and modular code.
- Advanced Functional Programming: Exploring advanced functional programming techniques will enable you to write cleaner and more maintainable code.
- State Management in Web Applications: Learn about tools like Redux and Context API for managing state in larger applications.
Explore Frameworks and Libraries
To expand your web development skills, consider learning popular frameworks:
- React: A JavaScript library for building user interfaces.
- Vue.js: A progressive framework for building interactive user applications.
- Angular: A complete application development framework including state solutions, routing, and more.
Continuous Improvement with Projects
The best way to consolidate your knowledge is by applying it in projects. Some projects you might consider include:
- To-Do List Application: A classic project that allows practicing with the DOM and event handling.
- SPA with Routing and State Management: Building a Single Page Application (SPA) with routing and state management is ideal for developing your frontend skills.
- Interactive Games: Building simple games in JavaScript is a great way to improve your programming logic and DOM manipulation.
Final Conclusion
This Intermediate JavaScript course has provided you with a solid foundation and advanced skills to tackle complex challenges in web development. Remember that learning is continuous, and each project and challenge along the way will help you become a stronger and more versatile developer.
Thank you for participating and best of luck with your future JavaScript projects!
You can take the Quiz for this topic:
You can also deepen in the following topics:
- Async/Await in JavaScript
- JavaScript ES6+
- Testing JavaScript and DOM with Jest
- Functional Programming in JavaScript
- Design Patterns in JavaScript
- Security in JavaScript
- Game Development with JavaScript
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
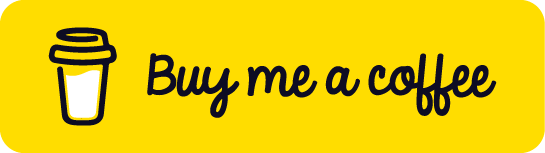
Chat with Chuck
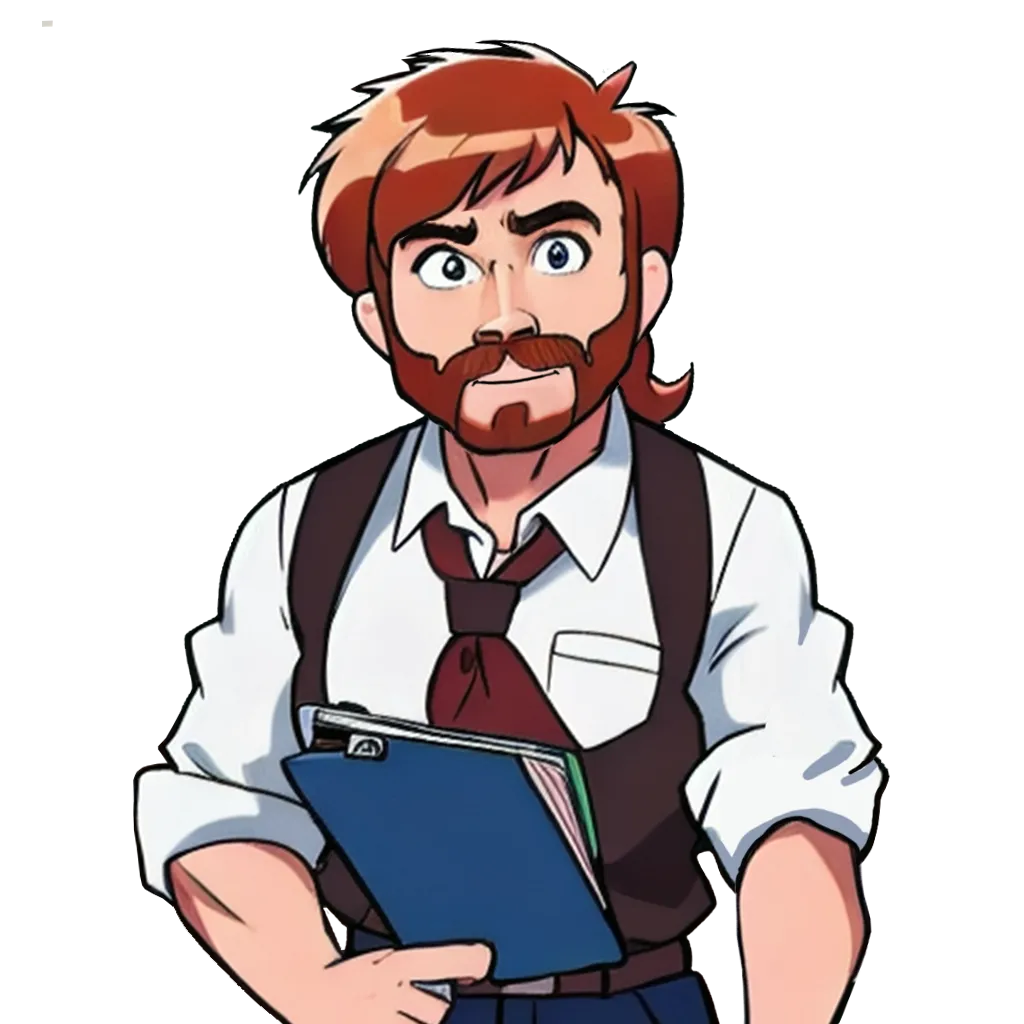
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps