Intermediate JavaScript
Advanced Destructuring and Spread Operator
In JavaScript, destructuring and the spread operator (...
) allow for more flexible and clearer data manipulation. These tools are very useful when working with complex data structures, such as objects and arrays, and make the code more readable and efficient. In this chapter, we will explore how to use them in an advanced way.
Object Destructuring
destructuring in javascript
Object destructuring allows you to extract values from an object and assign them to variables more concisely. This is especially useful when working with large or nested objects.
javascript
Default Values in Object Destructuring
We can assign default values to the variables if the properties do not exist in the object.
javascript
Array Destructuring
Array destructuring works similarly and allows extracting values from arrays into individual variables.
javascript
Skipping Elements in Array Destructuring
It is possible to skip elements during destructuring if we do not want to assign them.
javascript
Spread Operator for Objects
The spread operator allows combining objects and copying properties from one object to another.
javascript
Overwriting Properties with Spread
The spread operator also allows overwriting properties when combining objects.
javascript
Spread Operator in Arrays
The spread operator also works with arrays and is useful for copying or combining arrays.
javascript
Removing Elements Using Spread
It is possible to create a copy of an array with certain elements excluded using the spread operator.
javascript
Combined Applications of Destructuring and Spread
In complex situations, destructuring and the spread operator can be combined to make the code more compact and flexible. For example, when processing data from APIs or managing states in frameworks like React.
javascript
Conclusion
Destructuring and the spread operator are powerful tools in JavaScript that allow for clearer and more concise code. As you work with complex data, you'll find these techniques improve code efficiency and readability.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
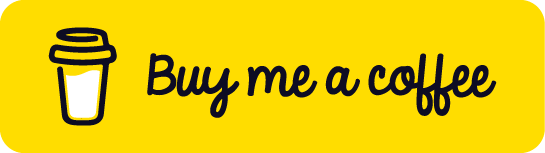
Chat with Chuck
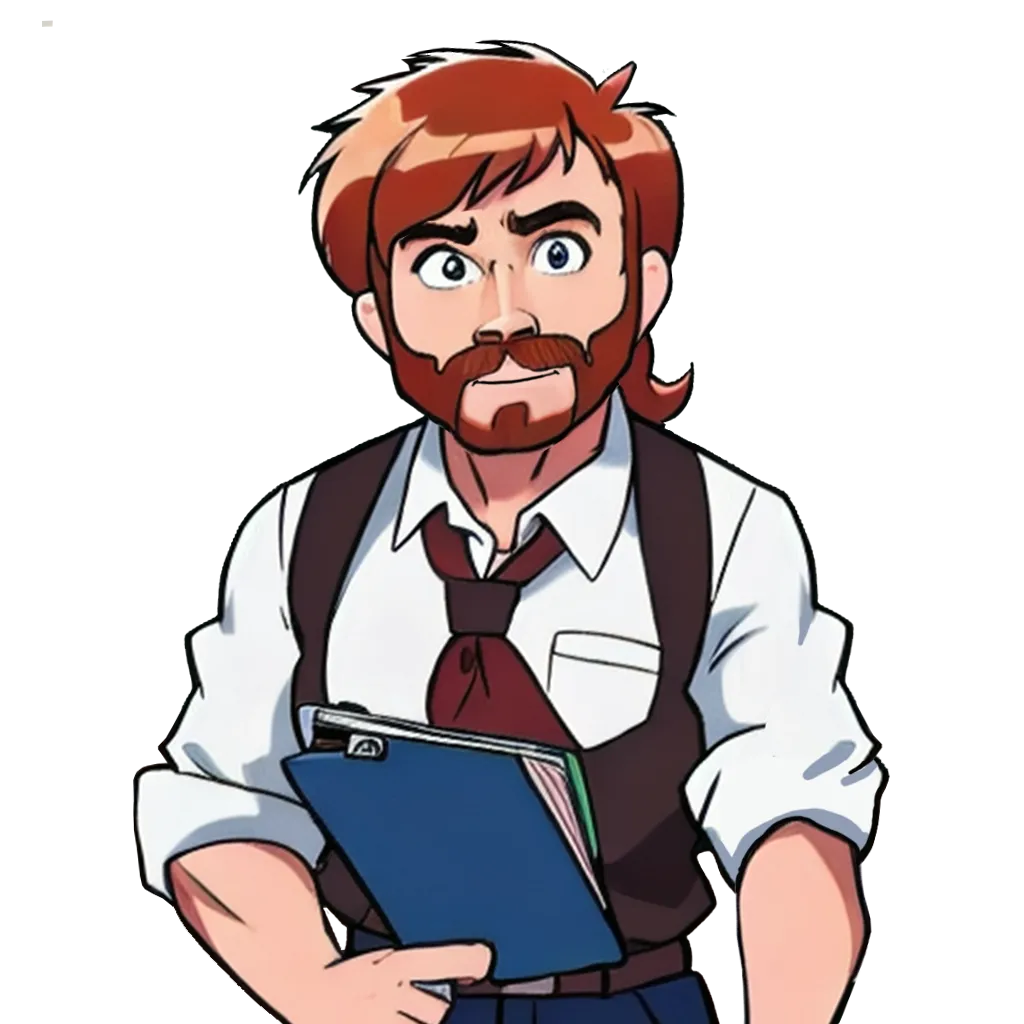
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps