Intermediate JavaScript
Classes and Object-Oriented Programming in Depth
JavaScript allows working with classes to implement the object-oriented programming (OOP) paradigm. In this chapter, we will delve into the use of classes, properties, methods, and design patterns in JavaScript.
concepts in OOP
Classes in JavaScript
Classes in JavaScript are a clearer way to define objects and methods.
The keyword class
allows us to define a class structure, and the constructor
method initializes its properties.
javascript
Static Methods
Static methods belong to the class itself, not to its instances, and are defined with the static
keyword.
javascript
Private Properties and Methods
In JavaScript, properties and methods can be declared private using the #
prefix, limiting access only within the class.
javascript
Class Inheritance
Inheritance allows a class to extend another, reusing properties and methods. The extends
keyword creates a child class based on a parent class.
javascript
Method Overloading
JavaScript allows redefining methods in a child class, overriding the method implementation in the parent class.
javascript
Polymorphism
Polymorphism allows treating different objects that share a common method similarly, allowing each object to execute its own version of the method.
javascript
Design Patterns in Classes
Singleton
The Singleton pattern ensures that only one instance of a class exists.
javascript
Factory
The Factory pattern provides a way to create instances of classes without specifying the exact class to create.
javascript
Conclusion
Classes and object-oriented programming in JavaScript allow for a clearer and more organized code structure, facilitating the reuse and implementation of advanced patterns. With private properties, inheritance, and design patterns, you can build robust and scalable applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
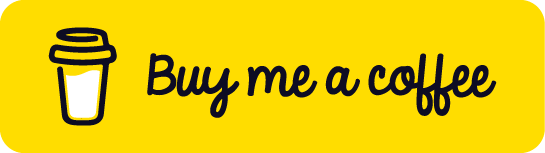
Chat with Chuck
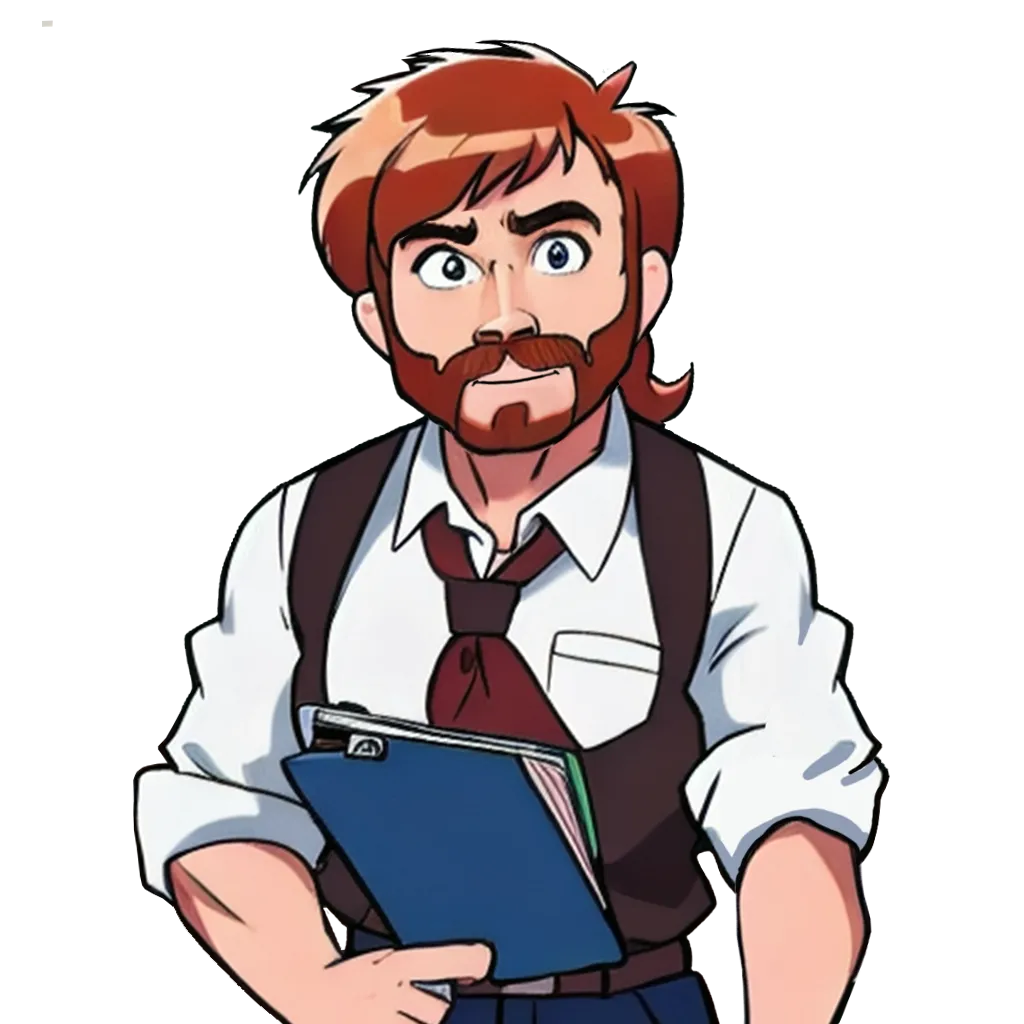
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps