Intermediate JavaScript
Modules in JavaScript
Modules in JavaScript allow you to divide code into reusable files and components, improving the organization and maintainability of applications. In this chapter, we will explore how to use modules to effectively structure your code.
this image shows a modules cheatsheet
Importing and Exporting Modules
JavaScript allows exporting and then importing functions, objects, or variables between files.
The export
keyword lets you share elements, while import
allows you to use them in other files.
javascript
javascript
Default Export
Default export allows exporting a single primary value or function from a module without explicitly naming it.
javascript
javascript
Renaming Imports and Exports
We can rename imports and exports to avoid name conflicts or improve readability.
javascript
javascript
Importing the Entire Module
It is also possible to import all elements of a module under a single name using * as
.
javascript
javascript
Using Modules in Browsers
To use modules in the browser, it's important to declare the type module
in the HTML script
tag.
html
Benefits of Using Modules
Using modules in JavaScript offers several benefits, such as:
- Better organization and separation of code into different files.
- Code reuse across multiple projects or applications.
- Isolation of variables, avoiding name conflicts.
- Loading only the necessary modules, improving performance.
Conclusion
Modules in JavaScript allow you to divide the code into reusable units, improving the organization and scalability of applications. By learning to export and import modules, you can build more modular and maintainable applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
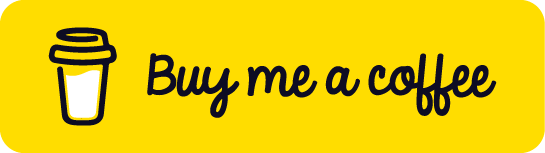
Chat with Chuck
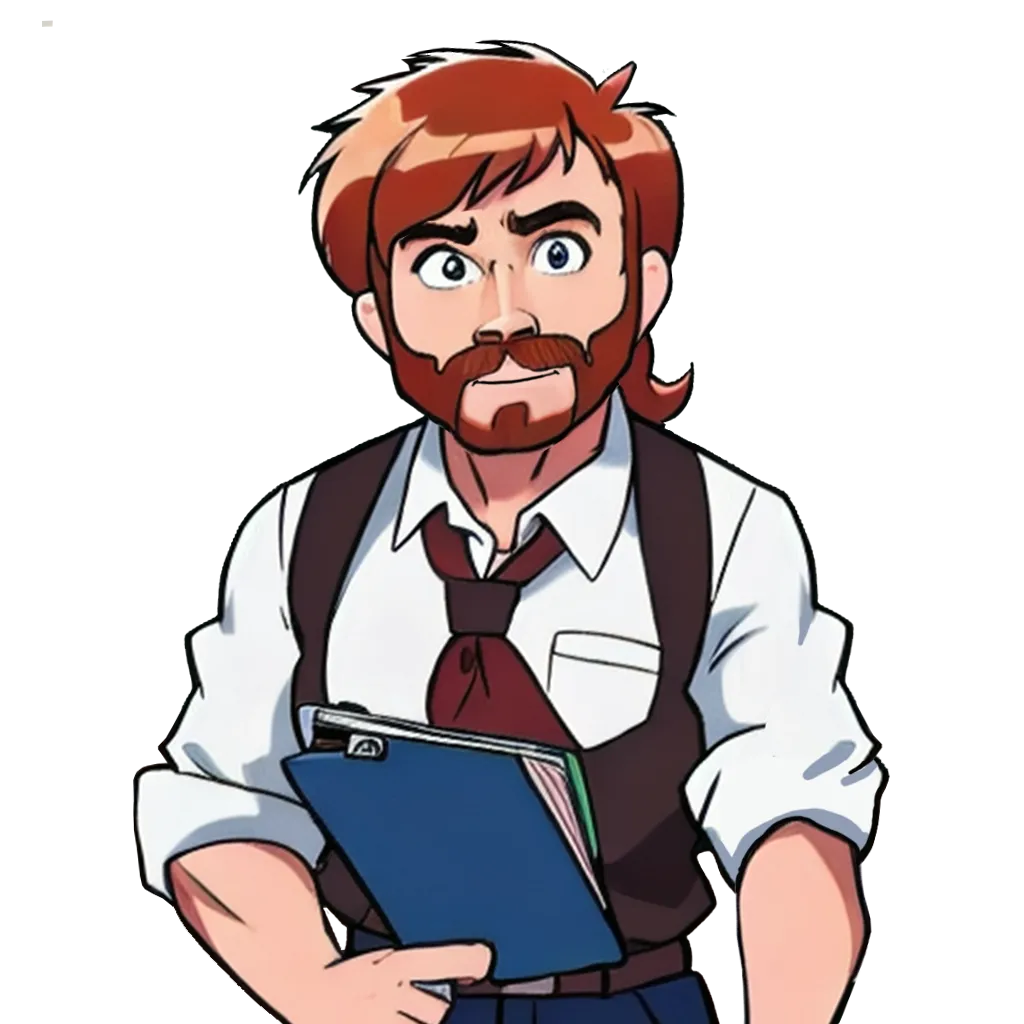
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps