Intermediate JavaScript
Functional Programming in JavaScript
Functional programming is a programming paradigm that focuses on the use of pure functions, immutability, and function composition. In JavaScript, it is possible to apply many concepts of functional programming to improve code clarity and maintainability.
This image shows a diagram of functional programming
Pure Functions
A pure function is a function that always returns the same result for the same arguments and has no side effects. Pure functions are predictable and easy to test.
javascript
Immutability
Immutability implies not changing the original data. Instead of modifying an object or array, we create a new one. This is fundamental in functional programming as it prevents errors associated with shared state.
javascript
Function Composition
Function composition is the process of combining small functions to build more complex functions. By composing functions, we can create clearer and more reusable data flows.
javascript
Using map
, filter
, and reduce
The methods map
, filter
, and reduce
are pillars of functional programming in JavaScript, allowing you to transform, filter, and accumulate data declaratively.
map
map
applies a function to each element of an array, returning a new array.
javascript
filter
filter
returns a new array containing only the elements that satisfy a condition.
javascript
reduce
reduce
allows combining all elements of an array into a single value, such as a sum or product.
javascript
Avoiding Side Effects
In functional programming, we avoid side effects, which means not modifying values outside the function's scope. This keeps the code predictable and makes debugging easier.
javascript
Currying
Currying is the process of transforming a function that takes multiple arguments into a series of functions that take a single argument. This facilitates function reuse and composition.
javascript
Conclusion
Functional programming in JavaScript allows you to write clearer, modular, and side-effect-free code. By applying principles like pure functions, immutability, and composition, it is possible to build more maintainable and robust applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
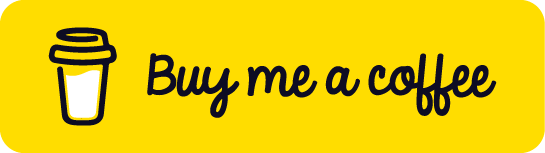
Chat with Chuck
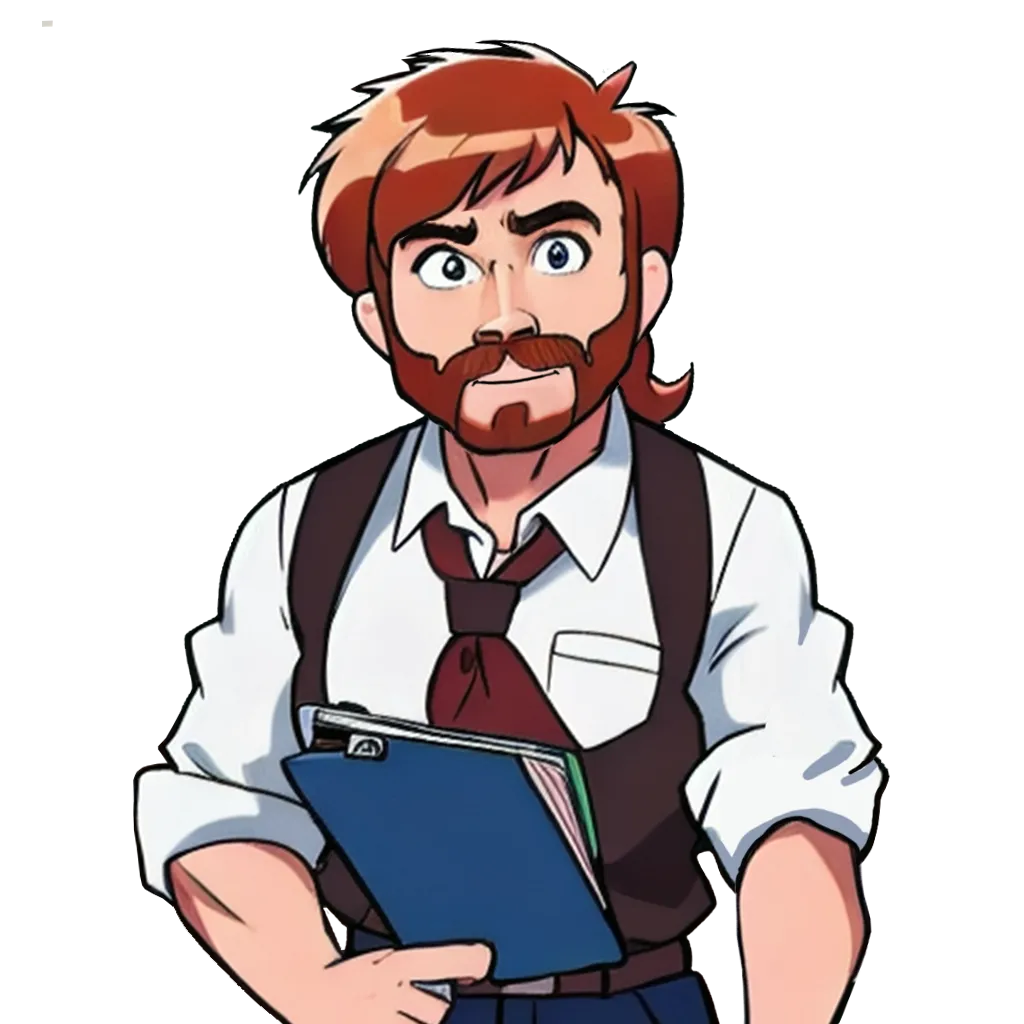
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps