Intermediate JavaScript
Best Practices and Code Styles
Writing clear, efficient, and maintainable code is fundamental in software development. In this chapter, we will explore best practices and code styles that improve the quality and readability of JavaScript code.
Use Descriptive Variable and Function Names
Variable and function names should be descriptive and reflect their purpose in the code.
javascript
Keep Functions Small and with a Single Responsibility
It is advisable that each function performs a single task. This facilitates debugging and code reuse.
javascript
Use const
and let
Instead of var
const
and let
offer better scope control and avoid errors associated with var
, such as hoisting and redeclaration.
javascript
Object and Array Destructuring
Destructuring allows extracting values from objects and arrays in a concise and clear way.
javascript
Avoid the Use of Magic Numbers and Strings
Magic numbers or strings (unexplained values) can make the code confusing. Use descriptive constants for these values.
javascript
Use Template Literals to Concatenate Strings
Template Literals facilitate concatenation and offer greater readability.
javascript
Efficient Error Handling
Use try/catch
blocks to handle errors and provide helpful messages. This prevents errors from stopping program execution.
javascript
Document the Code
Comments and documentation help understand the logic and purpose of the code.
javascript
Maintain Consistent Formatting
Use a consistent code format and tools like Prettier or ESLint to standardize the style.
- Prettier: Automatically formats the code to follow a consistent style.
- ESLint: Detects errors and suggests style improvements.
Conclusion
Best practices and code styles are essential to keep code readable, maintainable, and error-free. By applying these principles, you will improve the quality and sustainability of your JavaScript projects.
With this chapter, we conclude the Intermediate JavaScript course. Congratulations on completing the course! Keep practicing and exploring to continue improving your JavaScript skills.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
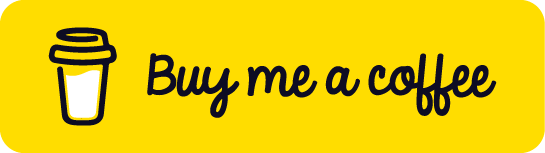
Chat with Chuck
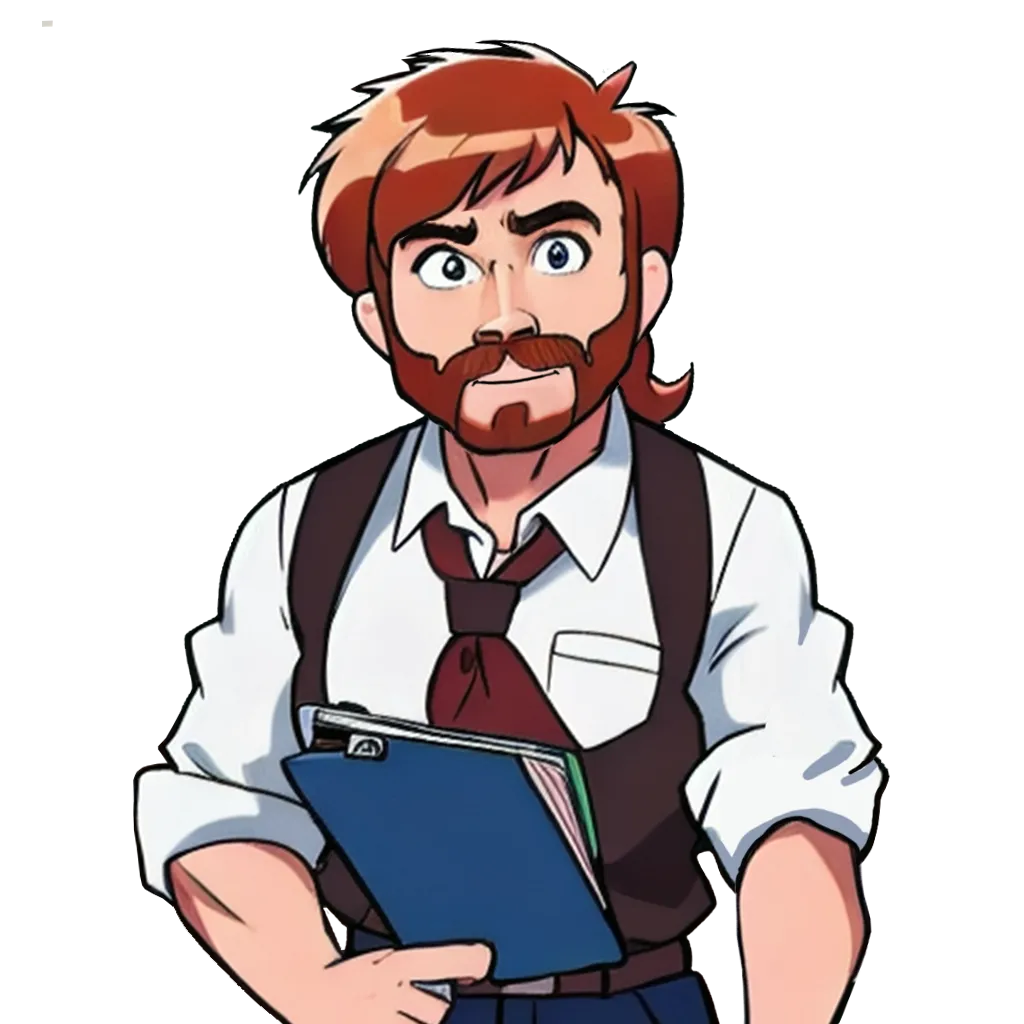
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps