Intermediate JavaScript
Functions and Closures in JavaScript
Functions in JavaScript are blocks of code that can be executed independently, and closures are a powerful feature of the functions that allow them to retain their execution context. In this chapter, we will explore advanced functions and the concept of closures.
Higher-Order Functions
A higher-order function is one that can take other functions as arguments or return a function. This allows building more flexible code patterns.
javascript
Closures
This image shows a diagram of how Closures work
A closure is a function that remembers the environment in which it was created, even after that environment has ended. This means that a closure's variables remain available even outside of their original scope.
javascript
Application of Closures in Programming
Closures are useful for creating private variables and functions that retain a state between calls.
javascript
Functions as Parameters
Passing functions as arguments is common in JavaScript, especially in methods like forEach
, map
, and filter
.
javascript
Functions that Return Functions
This pattern is common when you want to create more specific functions from general functions.
javascript
Scope and Context in Closures
It is important to understand how closures retain the execution context and can access variables defined at the time of their creation.
javascript
Practical Examples of Closures
Closures are frequently used to encapsulate logic and protect data, especially in the creation of custom functions or modules.
javascript
Conclusion
Functions and closures are advanced concepts in JavaScript that allow for better modularization and protection of code. By understanding how closures and higher-order functions work, more robust and maintainable applications can be built.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
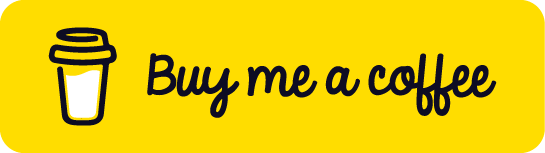
Chat with Chuck
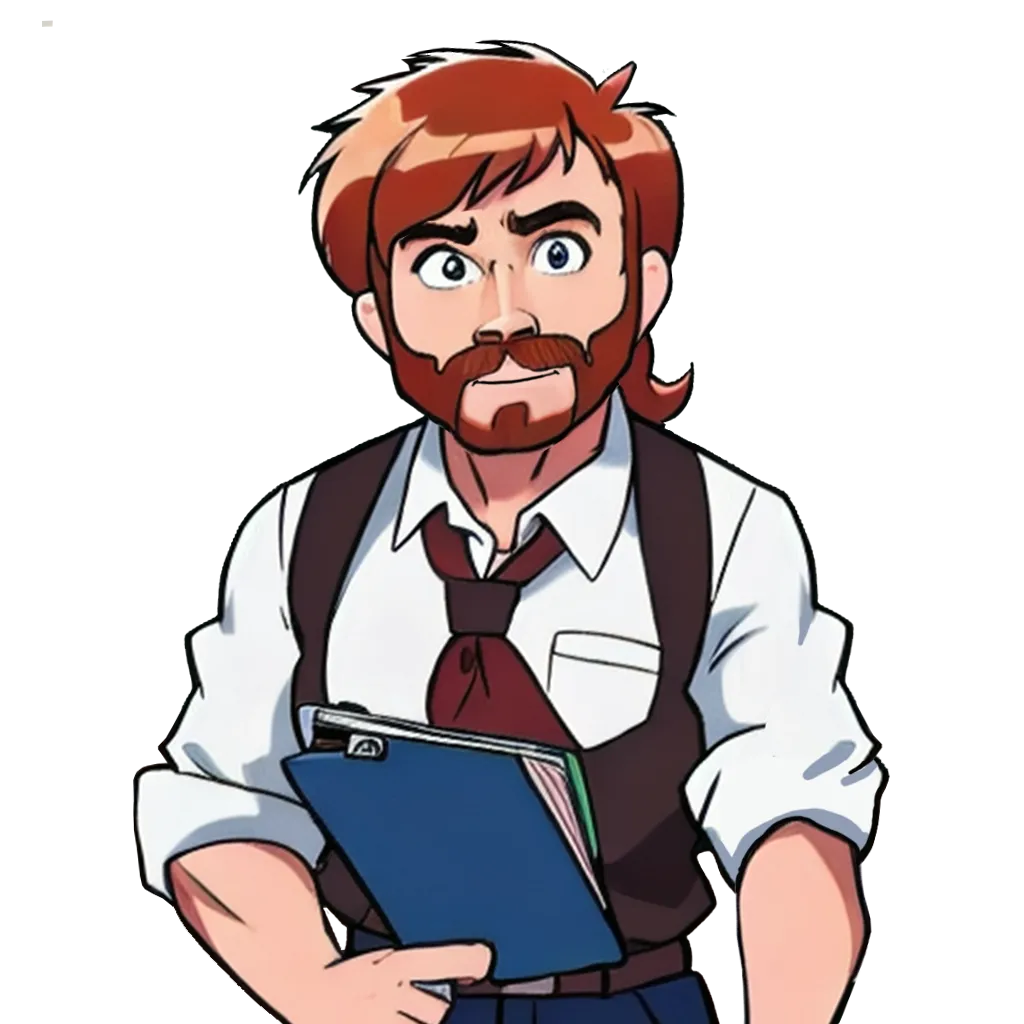
- Advanced Destructuring and Spread Operator
- Advanced Array Manipulation
- Functions and Closures in JavaScript
- Functional Programming in JavaScript
- Advanced DOM Handling
- Scope, Context, and `this` in Depth
- Advanced Promises and Async/Await
- Error Handling in JavaScript
- Modules in JavaScript
- Prototype Manipulation and Inheritance
- Classes and Object-Oriented Programming in Depth
- Design Patterns in JavaScript
- Asincronía Avanzada y Web APIs
- Reactive Programming with RxJS
- Expresiones Regulares Avanzadas en JavaScript
- Optimización del Rendimiento en JavaScript
- Introduction to WebAssembly
- Advanced Testing with Mocha, Chai, and Jest
- Advanced Debugging and Development Tools
- Best Practices and Code Styles
- Conclusions and Next Steps