Intermediate React
Accessibility Management in Interactive Components
Accessibility is a fundamental aspect in web application development, and React provides tools to facilitate the creation of accessible interfaces. In this chapter, we will explore how to implement accessibility in interactive React components to make applications inclusive for all users.
Basic Principles of Web Accessibility
Accessibility ensures that people with different abilities can interact with web applications. The basic principles include:
- Use of ARIA attributes: To describe the role and state of interactive elements.
- Focus management: Facilitate keyboard navigation.
- Alternative texts: Provide alternative texts for screen readers.
- Color contrast: Ensure the readability of texts and visual elements.
components on a web page
Use of ARIA Attributes in React Components
ARIA attributes help describe the role and state of elements that are not easily understandable by assistive technologies. Below, we show how to apply ARIA attributes in React.
Example with ARIA Attributes in Buttons
Here, we use aria-label
and aria-disabled
to describe the purpose and state of a button:
javascript
Focus Control for Keyboard Navigation
Focus is crucial for users who navigate using the keyboard. We can use tabIndex
and focus
in React to control and enhance the keyboard navigation experience.
Focus Management Example
This example demonstrates how to control focus on an input field when the component mounts:
javascript
Creating Accessible Forms
Forms are key elements in web accessibility. React facilitates handling accessible forms by providing tools to correctly associate labels and states.
Accessible Form Example
This example shows how to create a form with accessible labels and error messages:
javascript
Ensuring Adequate Contrast and Accessible Colors
Color contrast is vital for readability, especially for users with visual impairments. Tools like color-contrast
can help choose accessible color combinations.
Example of a Button with Adequate Contrast
In this example, we use high contrast colors to improve the readability of a button:
javascript
Best Practices in Accessibility for React Components
- Use ARIA Attributes Only When Necessary: Add ARIA attributes only when native elements do not provide the desired functionality.
- Avoid Multiple Focuses: Control focus to avoid confusion, ensuring each component has only one focused element.
- Implement Accessible Contrasts: Use contrast tools to ensure used colors meet accessibility standards.
Conclusion
Accessibility is a crucial component in developing inclusive React applications. In this chapter, we explored how to implement accessibility practices through the use of ARIA attributes, focus control, design of accessible forms, and color contrast management.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
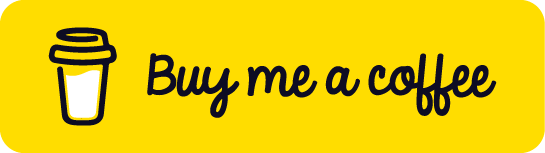
Chat with Chuck
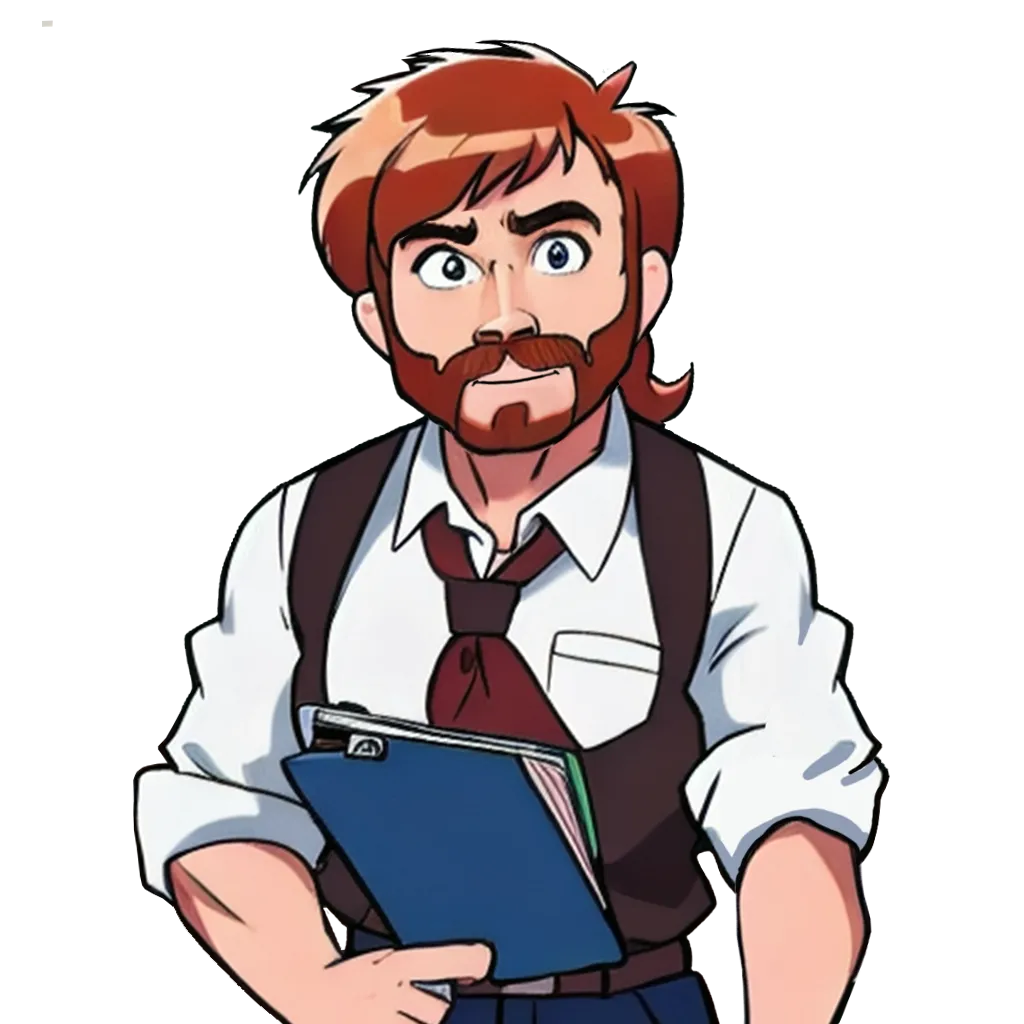
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React