Intermediate React
Render Props and Higher-Order Components (HOC)
In this chapter, we will explore two advanced patterns in React: Render Props and Higher-Order Components (HOC). Both are useful for sharing logic between components and improving code reusability in a React application.
hoc y render props
Introduction to Render Props
The Render Props pattern involves passing a function as a prop to a component, instead of passing a static value or a specific function. This allows a component to control the content it renders and share logic between components more flexibly.
Basic Example of Render Props
In this example, we have a MouseTracker
component that uses Render Props to share the mouse position with other components:
javascript
Using the Component with Render Props
Below, we show how to use MouseTracker
with Render Props:
javascript
Introduction to Higher-Order Components (HOC)
A Higher-Order Component is a function that takes a component and returns a new component with additional functionality. HOCs are useful for applying common logic to multiple components without repeating the code.
Basic Example of Higher-Order Component
In this example, we create a HOC withUser
that provides user data to a component:
javascript
Using the Higher-Order Component
Below, we show how to use withUser
in a component:
javascript
Comparison between Render Props and Higher-Order Components
Both patterns, Render Props and Higher-Order Components, allow sharing logic and functionality between components. However, there are key differences:
- Render Props is ideal when the component needs to directly control the content it renders.
- Higher-Order Components are useful when you want to extend a component’s functionality without modifying its internal implementation.
Best Practices for Render Props and HOC
- Avoid deep nesting: When using Render Props or HOCs excessively, you may end up with a hard-to-maintain component structure. Try to limit their use to avoid "nested higher-order components" that are difficult to read.
- Descriptive naming for HOCs: Ensure that the name of the HOC clearly describes its purpose to facilitate code readability.
- Consider using Hooks as an alternative: Hooks can offer a cleaner way of sharing logic in functional components, without the limitations of HOCs.
Conclusion
Render Props and Higher-Order Components are advanced patterns that offer flexibility and reusability to code in React. In this chapter, we explored how to implement them and when to use them to share logic between components.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
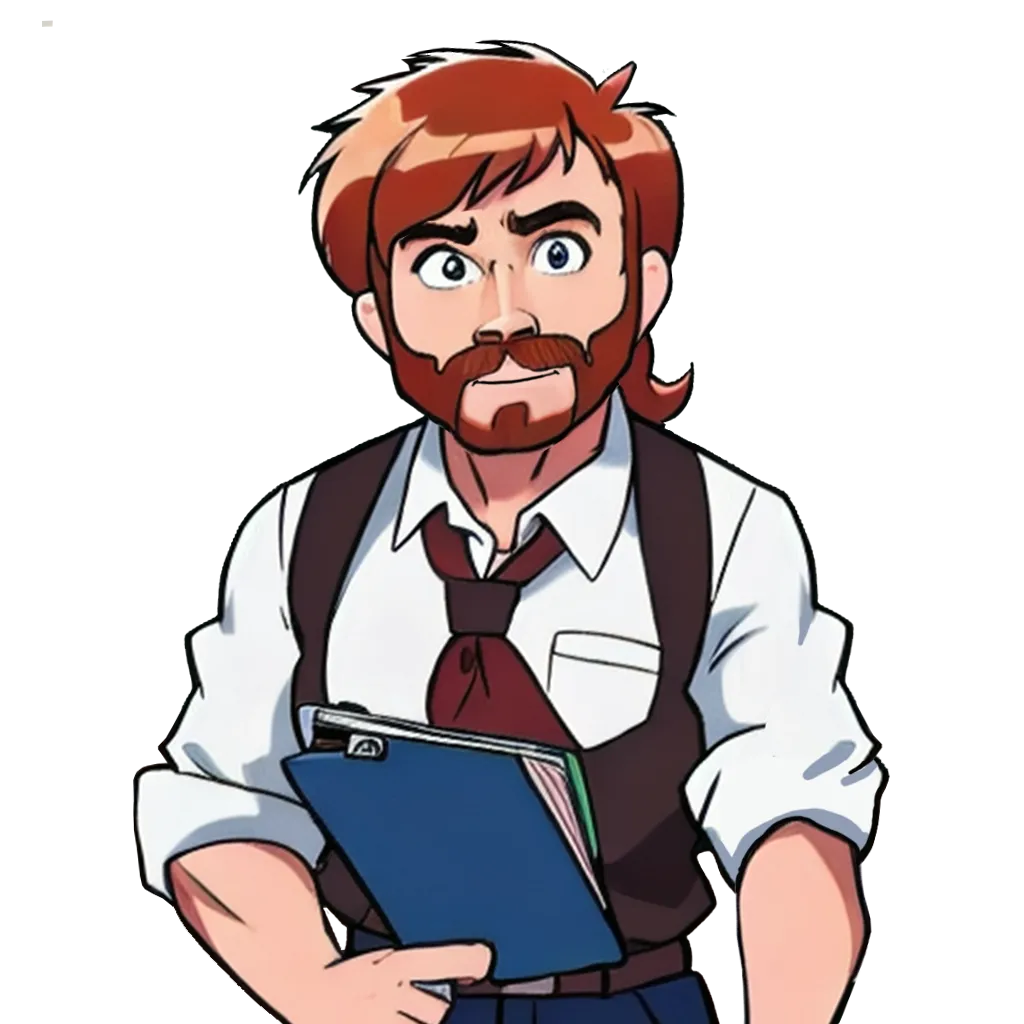