Intermediate React
Refs and Direct DOM Manipulation
In React, direct manipulation of the DOM is less common due to the virtual DOM representation system. However, in certain cases, it is necessary to interact directly with DOM elements. In this chapter, we will learn how to use Refs to access and manipulate DOM elements and explore some practical cases.
Dom manipulation with Refs
What Are Refs in React?
Refs are a function provided by React to reference elements or components created in the DOM. They allow us to directly manipulate the DOM of these elements without compromising React's declarative structure, being useful for cases like animations, form control, and managing external elements.
Creating and Basic Use of a Ref
Refs are created using the useRef
hook in functional components. The created reference is maintained throughout the component's entire lifecycle without being reinitialized at every render.
Basic Usage Example with useRef
In this example, we use a Ref to automatically focus an input field when the component mounts:
javascript
DOM Elements Manipulation with Refs
Refs are useful for directly controlling DOM elements when necessary, such as playing or pausing a video or manipulating style properties of a specific element.
Example: Controlling a Video Element
Here, we use a Ref to pause or play a video through control buttons:
javascript
Refs and Controlled Components
Although Refs are useful for directly accessing the DOM, in forms it is preferable to use controlled components with states. However, in situations where direct access is necessary, Refs can facilitate the process.
Example: Form Validation with Refs
This example shows how to use Refs to access multiple form fields and validate them upon submission:
javascript
Advanced Use of Refs: Forwarding Refs
Forwarding Refs allows passing a ref to a child component, granting direct access to a DOM element within the child component. This is useful when a reference is needed in reusable components or libraries.
Forwarding Refs Example
Here we have a CustomInput
component that accepts a Ref and forwards it to the internal input:
javascript
Using the Component with Forwarding Refs
To use the CustomInput
component, a Ref is assigned from the parent component:
javascript
Best Practices When Using Refs
- Use Refs Sparingly: Refs are a powerful tool, but excessive use is not recommended, as they can complicate the application's state management.
- Prefer Controlled Components for Forms: In forms, controlled components with states are more declarative and easier to maintain.
- Forwarding Refs for Reusable Components: Implement
forwardRef
in reusable components to facilitate DOM access from parent components.
Conclusion
Refs and direct DOM manipulation are advanced tools that allow accessing and manipulating specific elements in a React application. In this chapter, we explored the basic use of Refs, DOM elements manipulation, and forwarding Refs for reusable components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
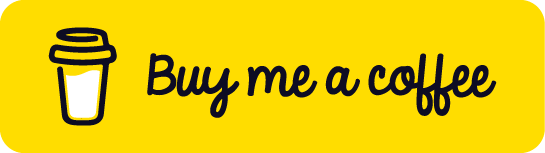
Chat with Chuck
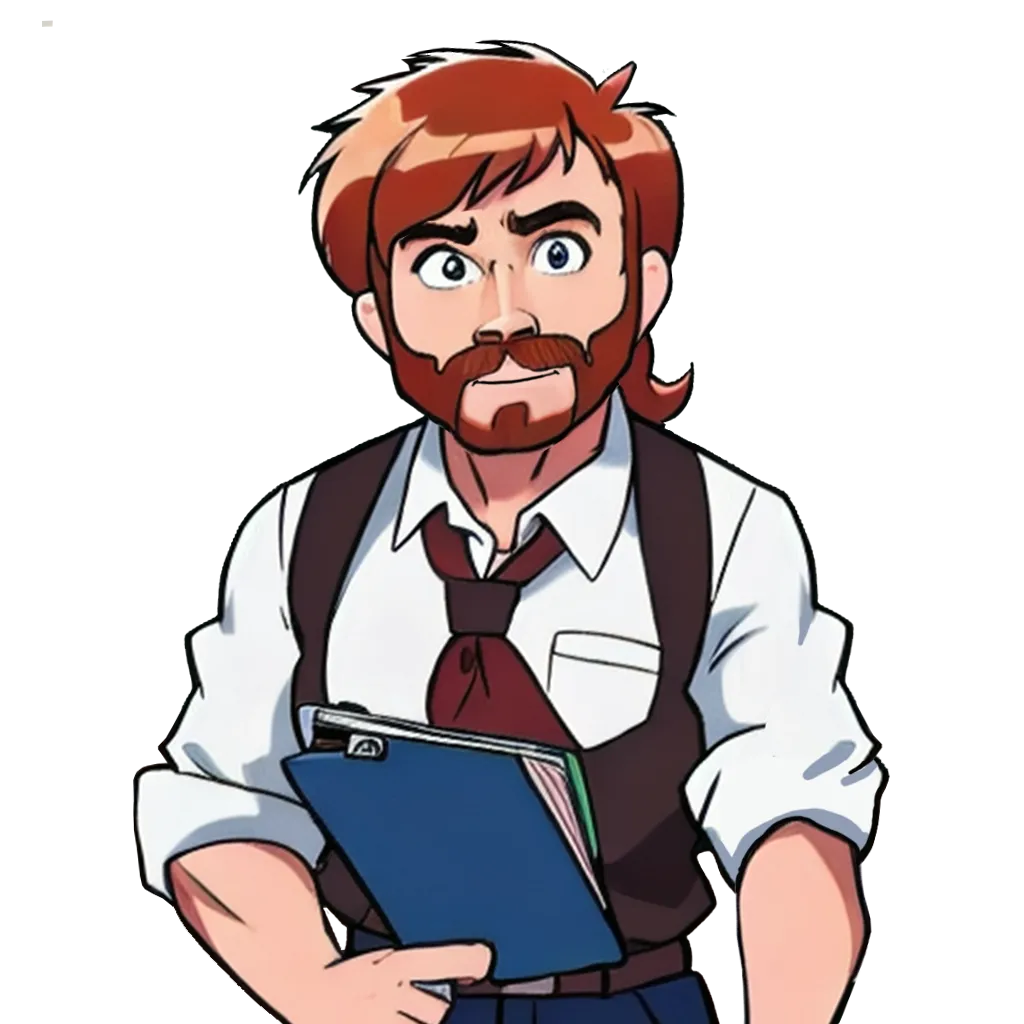
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React