Intermediate React
Testing Components with Mocking and Integration Tests
Testing in React ensures that components work as expected in different scenarios. In this chapter, we will explore advanced testing techniques, including mocking and integration tests. These strategies are essential to verify the interaction between components and simulate external behaviors in complex applications.
React testing
Introduction to Mocking in Tests
Mocking allows simulating functions, modules, or external services in tests, avoiding dependencies and achieving a controlled environment for tests. In React, mocking is commonly used to isolate components and simulate data.
Mocking Example with Jest
In this example, we use Jest to mock an API function that returns user data:
javascript
Component Testing with Data Mocking
When testing components, we can simulate data to validate how the component behaves with different inputs. This is useful in components that rely on APIs or external functions.
Mocking Props Example in a Component
In this example, we test a component that displays a user list by simulating the data:
javascript
Integration Tests Between Components
Integration tests verify the interaction between multiple components, ensuring that the components work together correctly. In React, integration tests are useful to validate user flows and more complex scenarios.
Integration Test Example with Events
In this example, we test the integration of a button and a text field:
javascript
Using Mock Service Worker (MSW) to Simulate APIs in Tests
Mock Service Worker (MSW) allows simulating APIs in tests, which is useful for applications that depend on external data. MSW intercepts requests and returns simulated responses.
Mock API Example with MSW
Here, we configure MSW to simulate a user API:
javascript
Best Practices for Mocking and Integration Tests
- Isolate Components with Mocking: Simulate functions and data to avoid dependencies in unit tests.
- Use Integration Tests for Critical Flows: Verify interactions between components that are key to the user experience.
- Simulate APIs with MSW for Flexibility: MSW allows simulating different API responses, facilitating complex integration tests.
Conclusion
The use of mocking and integration tests in React allows verifying the behavior of components and their interaction in different scenarios. In this chapter, we explored how to test with Jest, API simulation with Mock Service Worker, and techniques for integration tests.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
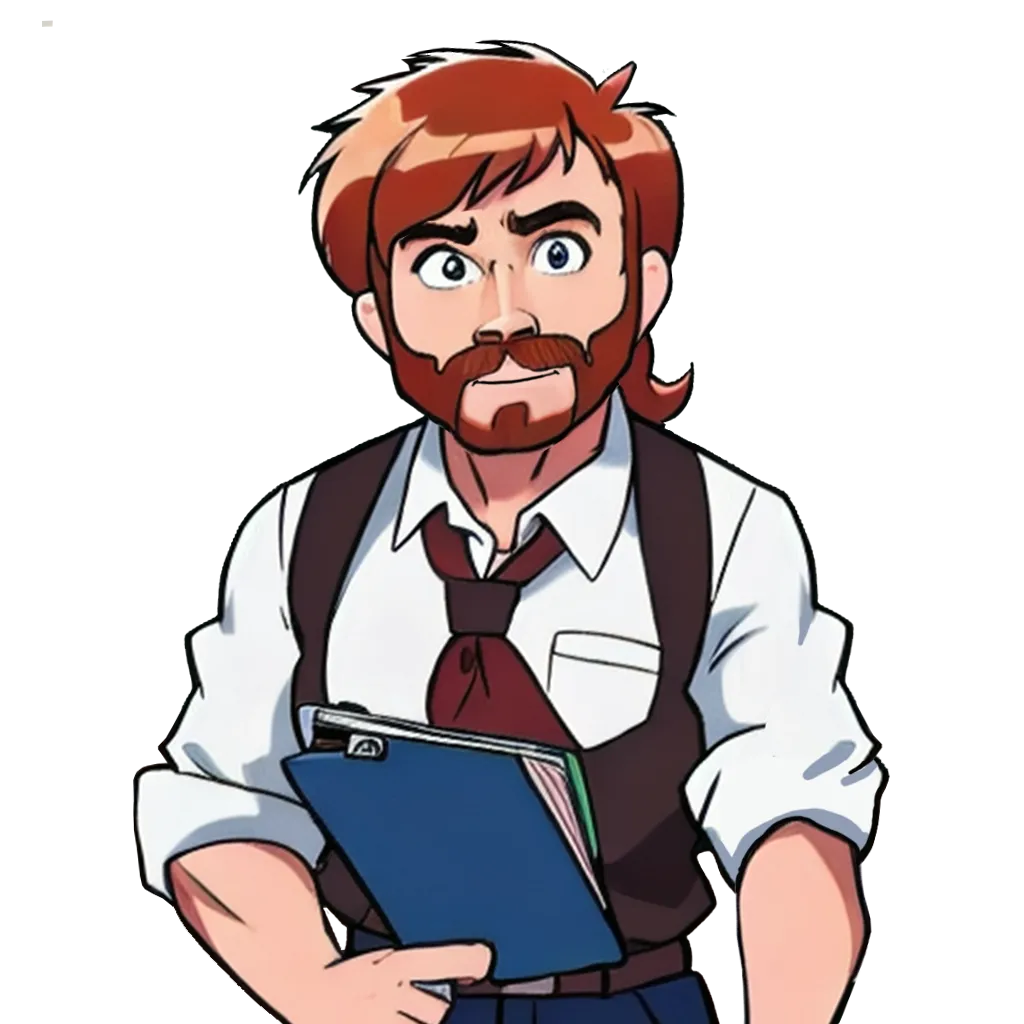