Intermediate React
Lazy Loading and Code Splitting
As React applications grow in complexity, the initial load time can significantly increase. This can affect the user experience, especially on devices with slow connections. To mitigate this issue, React offers tools like Lazy Loading and Code Splitting that allow for efficient code loading.
lazy loading and code splitting in react
Introduction to Lazy Loading
Lazy Loading is a technique that allows components and other resources to be loaded only when needed. This reduces the initial load time and improves the user experience by reducing the size of the initial bundle.
Implementing Lazy Loading in React
React provides the React.lazy
method to implement Lazy Loading in components. React.lazy
is used along with the Suspense
component, which acts as a wrapper that shows a loading message while the component loads asynchronously.
javascript
Code Splitting to Divide Code
Code Splitting is the process of dividing code into different parts or chunks, which are loaded only when needed. React, along with tools like Webpack, facilitates the implementation of Code Splitting dynamically. This is particularly useful for applications with multiple routes.
Code Splitting Example in React
By splitting the code of individual routes, only the necessary code for the current route is loaded, improving the initial load time.
javascript
Lazy Loading and Code Splitting in Nested Components
In complex applications, it is common to have nested components that depend on specific data or user actions. Lazy Loading and Code Splitting can be implemented in these components to avoid unnecessary loads and improve performance.
Lazy Loading Example in Nested Components
javascript
Best Practices for Lazy Loading and Code Splitting
- Split only large components: Apply Lazy Loading and Code Splitting on large components or pages that are not immediately rendered.
- Use Suspense to improve user experience: Suspense allows custom loading messages to be displayed, providing a better visual experience.
- Evaluate the size of each chunk: Use analysis tools like Webpack Bundle Analyzer to evaluate chunk sizes and ensure Code Splitting is optimized.
Conclusion
Lazy Loading and Code Splitting are effective techniques for improving load times and user experience in React applications. In this chapter, we explored how to implement these techniques to optimize the loading of components and routes in React applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
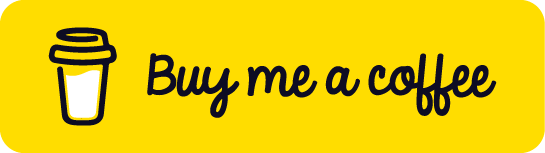
Chat with Chuck
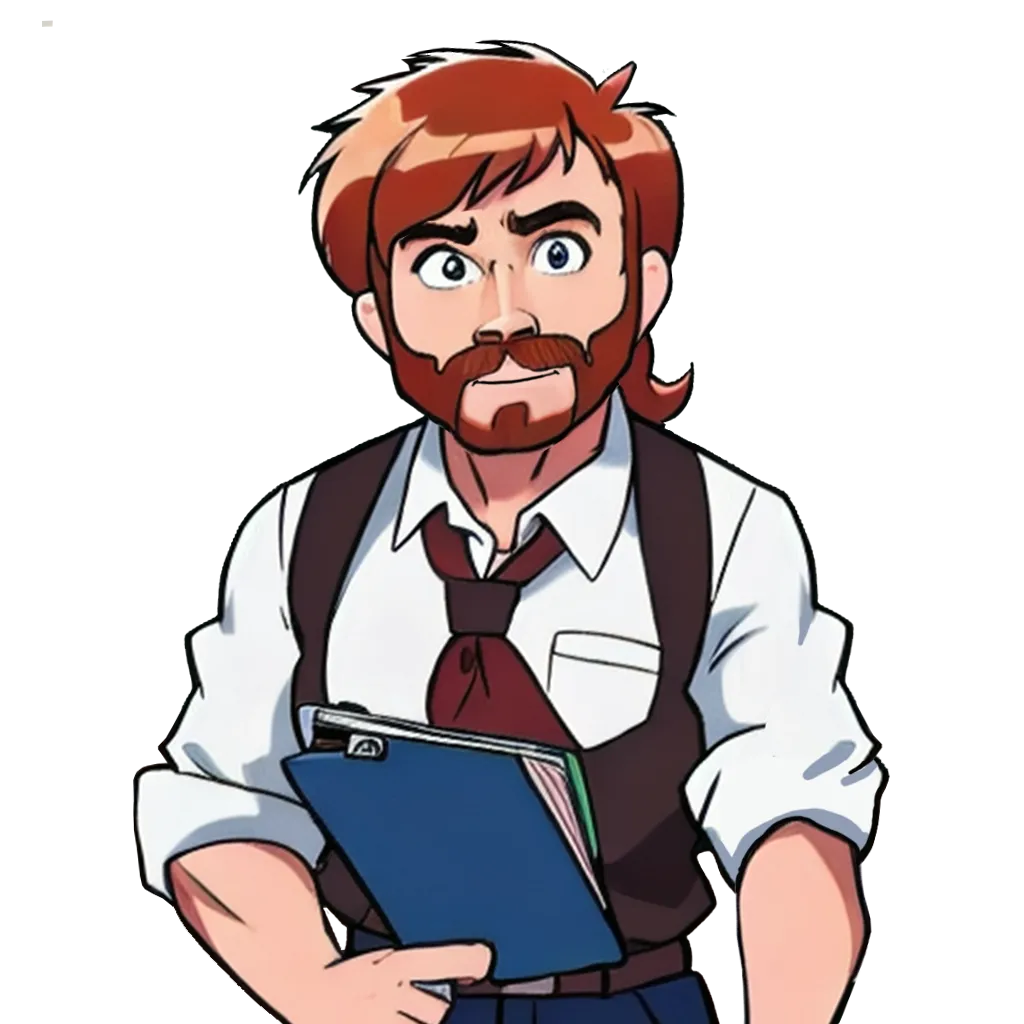
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React