Intermediate React
Advanced Conditional Rendering
Conditional rendering is a technique that allows showing or hiding elements in the user interface based on certain conditions. In this chapter, we will explore advanced techniques for implementing conditional rendering in React, optimizing component display in complex applications.
react conditional rendering methods
Basic Concepts of Conditional Rendering
Conditional rendering in React is based on logical expressions to decide whether a component should be displayed or not. React offers several ways to implement this logic, from simple conditional operators to the use of component-returning functions.
Basic Conditional Rendering Example
A simple method of conditional rendering is the &&
operator, which evaluates whether to display a component:
javascript
Advanced Conditional Rendering with Functions
In large applications, conditional rendering can become complex. A technique to simplify this is to encapsulate the rendering logic in functions. This improves code clarity and facilitates reuse.
Example with Rendering Functions
In this example, we use a function to decide which message to show based on the user's state:
javascript
Rendering Based on Switch or Map for Complex Views
To handle multiple conditions, it is common to use structures like switch
or map
. This allows managing complex conditions in an organized and readable manner.
Rendering with Switch Example
In this example, a message component uses switch
to decide the content according to the user's role:
javascript
Rendering Based on Dynamic Components
Sometimes it is useful to dynamically decide which component to render based on a property or state. React allows this by using objects that map conditions to specific components.
Example with Dynamic Components
Here we use a mapping object to choose and render a specific component based on the state:
javascript
Best Practices in Advanced Conditional Rendering
- Encapsulate Conditions in Functions: Move conditional logic to independent functions to improve clarity and reuse.
- Use Maps for Dynamic Components: Mapping objects facilitate the selection and rendering of complex components based on specific states.
- Optimize Rendering Logic: Avoid deeply nested conditions in JSX to improve code readability.
Conclusion
Advanced conditional rendering allows developers to effectively manage element display in complex React applications. In this chapter, we explored techniques and patterns for implementing more complex and organized rendering logic.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
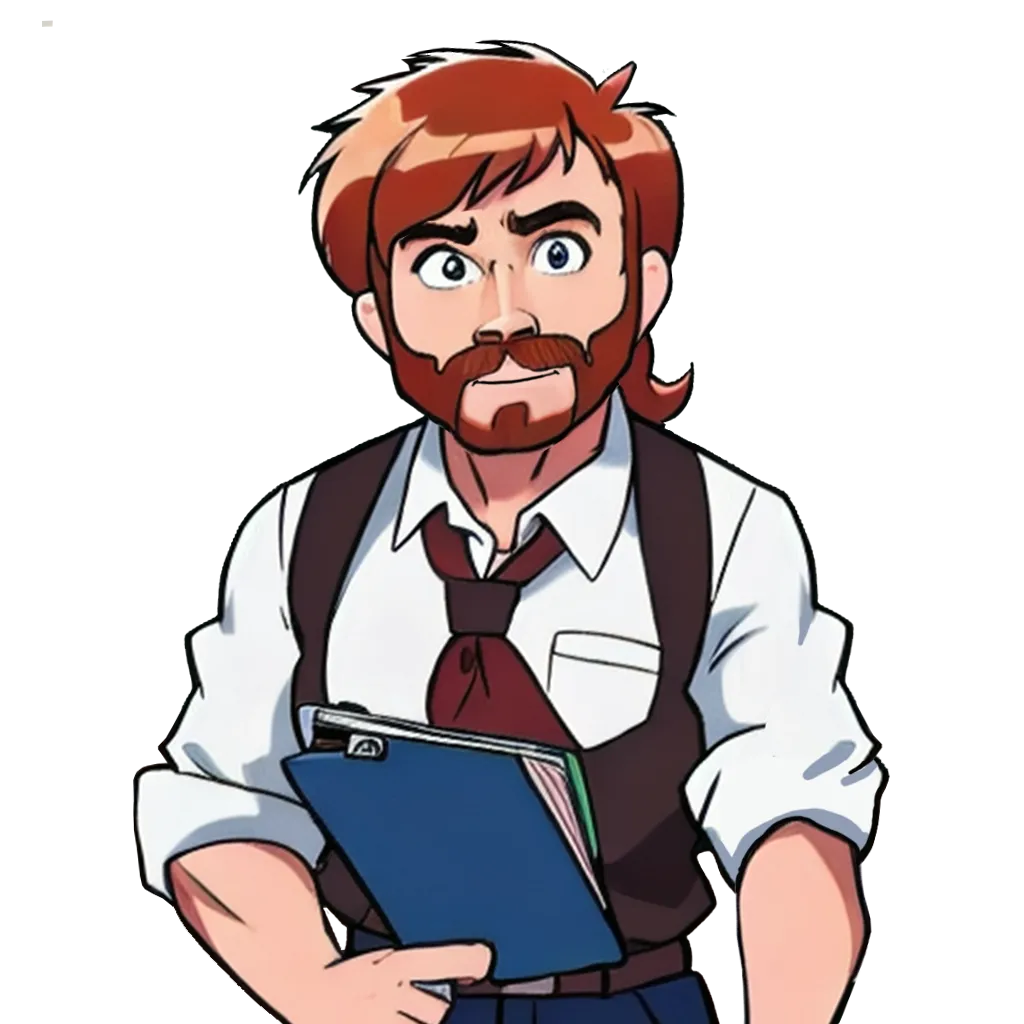