Intermediate React
Advanced Custom Hooks Patterns
In React, custom hooks allow you to encapsulate state logic and reuse it across different components. As applications grow, advanced custom hook patterns become useful for simplifying the code and improving maintainability. In this chapter, we will explore how to create custom hooks and review advanced patterns to optimize logic reuse.
custom hooks
Creating a Custom Hook
A custom hook is a function that uses React hooks (useState
, useEffect
, etc.) to encapsulate logic and return reusable data or functions. Custom hooks facilitate the separation of state logic into more manageable modules.
Example of a Basic Custom Hook
In this example, we create a useCounter
hook that manages a counter with functions to increment and decrement:
javascript
Handling Asynchronous Effects with Custom Hooks
It is common to handle asynchronous effects, such as API requests, within custom hooks. Encapsulating asynchronous logic in a custom hook allows easy control over loading and error states.
Example: API Call Hook
In this example, we create a useFetch
hook that makes a request to a given URL and returns the data, loading state, and any errors:
javascript
Using References and Persistent States in Hooks
Custom hooks can handle references and persistent states with useRef
and useReducer
, allowing additional control over state logic. This is useful in cases where the state requires complex manipulation or tracking across multiple renders.
Example: Reducer-Based Counter Hook
Here we create a custom hook that uses useReducer
to handle a counter with more complex actions:
javascript
Composing Custom Hooks
Composing hooks allows for combining multiple custom hooks into a single one, which is useful when logic requires multiple states or effects. This approach helps keep the code modular and reusable.
Example of Hook Composition
In this example, we create a useUserStatus
hook that combines useFetch
and useCounter
to obtain user data and track the number of visits:
javascript
Best Practices for Using Custom Hooks
- Encapsulate Complex Logic: Use custom hooks to encapsulate repetitive or complex logic and make the code cleaner.
- Avoid Side Effects: Custom hooks should avoid side effects that could interfere with other components.
- Compose Hooks for Modularity: Combine hooks when shared logic is needed, improving the application's modularity.
Conclusion
Advanced custom hook patterns allow encapsulating and reusing logic in complex React applications. In this chapter, we explored how to create basic and advanced custom hooks, including handling asynchronous effects, persistent references, and hook composition.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
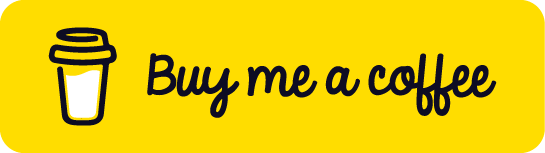
Chat with Chuck
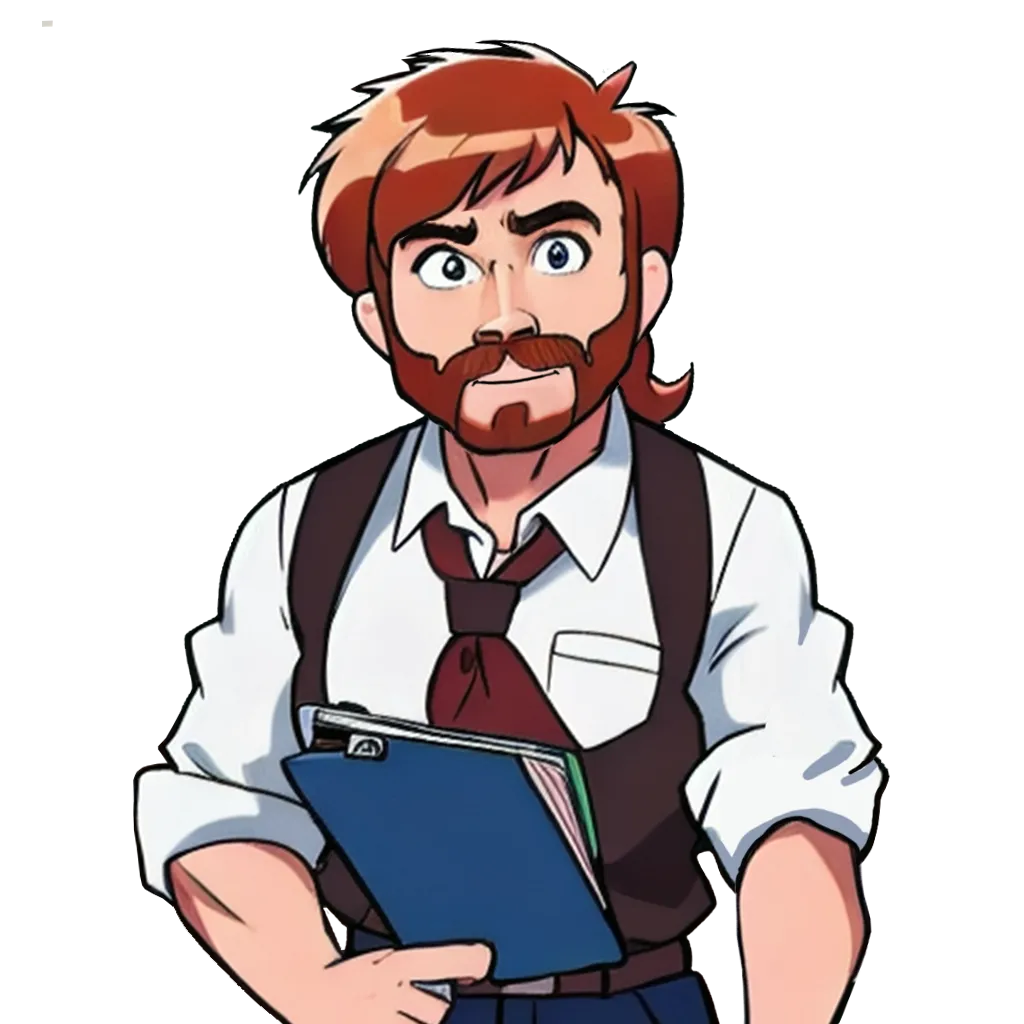
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React