Intermediate React
Advanced State Management with Context API and useReducer
In this chapter, we will delve into state management in React using Context API and the useReducer hook. Both concepts are fundamental for managing complex and shared states in medium and large applications, where traditional approaches like useState
may be insufficient.
Introduction to Context API
context api
The Context API allows sharing information between components without needing to manually pass props through every level of the component hierarchy. This approach is ideal for handling global data, such as color themes, language settings, or even a user's authentication state.
Creating a Context
To create a context, we use React's createContext
method. This method creates a Context object, which is composed of two parts: a Provider and a Consumer. Here is a basic example of how to define and use a context.
javascript
Consuming a Context
To consume the context in a component, we use the useContext
hook, which allows us to directly access the context value without needing to use an explicit Consumer.
javascript
Using useReducer for Complex State Management
The useReducer
hook is particularly useful when a component's state is complex or when state updates depend on specific actions. Unlike useState
, useReducer
requires a reducer, which is a pure function that takes the current state and an action, and returns a new state.
Basic Example with useReducer
In the following example, we will use useReducer
to manage a counter with increment and decrement actions:
javascript
Combining Context API and useReducer
Combining Context API and useReducer is an advanced technique for managing global states in large applications. By combining these two approaches, we achieve centralized state and actions management while sharing the global state across components without the need to pass props.
Example: Managing Authentication State
Let's imagine an example where we need to manage the user's authentication state in our application. We will use useReducer
to define login and logout actions and Context API
to provide this state globally.
javascript
Consuming Authentication State
Now that we have the authentication context, we can consume it in our components as follows:
javascript
Conclusion
In this chapter, we explored how to use the Context API and the useReducer hook to manage global and complex states in a React application. These concepts are essential for building scalable and well-structured applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
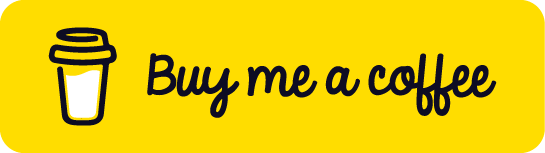
Chat with Chuck
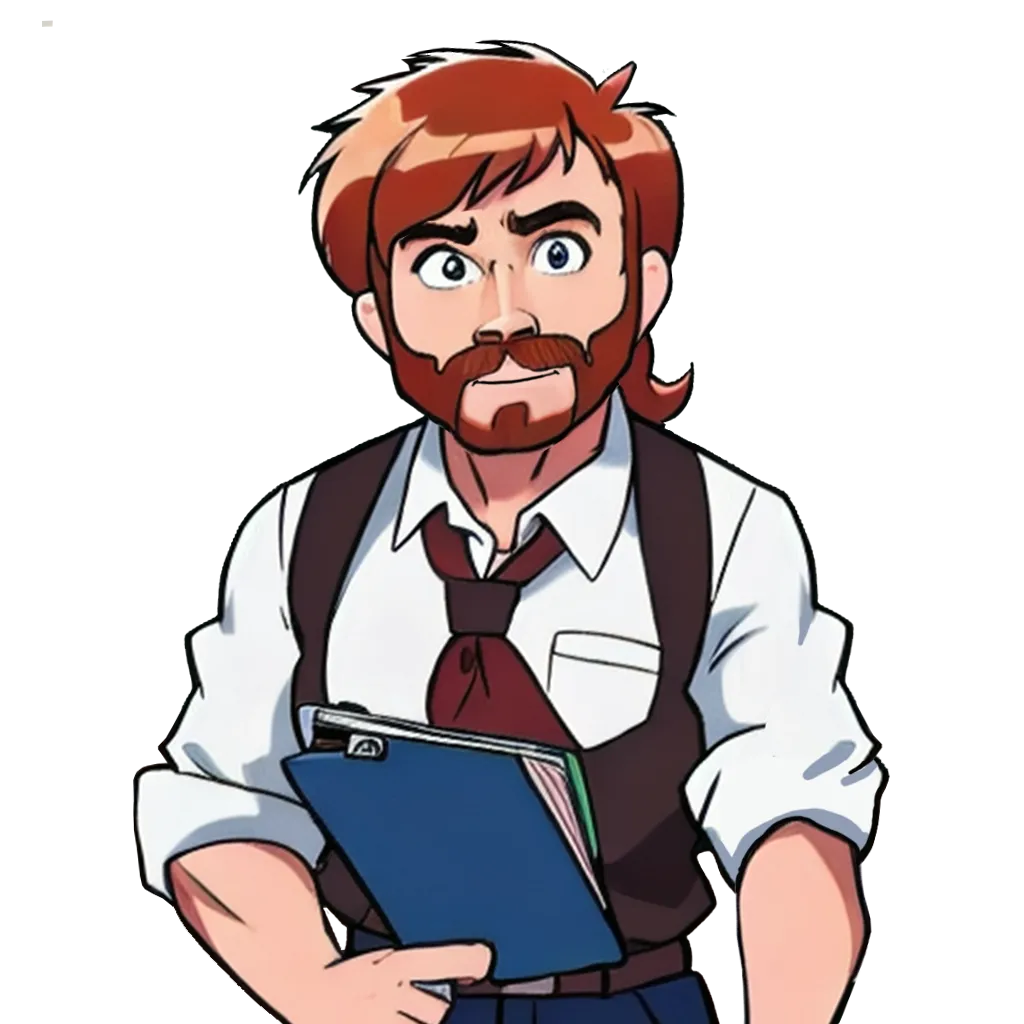
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React