Intermediate React
Performance Optimization in React
In this chapter, we will address performance optimization techniques in React. The efficiency of a React application is crucial, especially when dealing with complex projects and large amounts of data. The techniques presented here include the use of React.memo, useMemo, and useCallback, among other tools.
Identifying Performance Issues
The first step to optimizing a React application is identifying problematic areas. React provides several development tools, such as the React Profiler, which helps detect components that unnecessarily re-render.
This image shows the React Profiler interface
Using React Profiler
To use the React Profiler, add the Profiler
component around the components you want to analyze. The Profiler's callback function captures information about renders, allowing you to analyze details about their performance.
javascript
React.memo to Prevent Unnecessary Re-Renders
React.memo
is a helpful tool for memoizing functional components and preventing them from re-rendering if their props have not changed. This is especially beneficial in components that receive large amounts of data.
Example of Using React.memo
javascript
Using useMemo to Memoize Expensive Calculations
The useMemo
hook allows you to memoize computed values that can be expensive in terms of performance. This is useful when the calculation of a value depends on props or states that do not change frequently.
Example with useMemo
javascript
Optimizing Callbacks with useCallback
When passing a function as a prop to child components, a new reference is created each time the parent component renders, leading to unnecessary re-renders in the child component. useCallback
memoizes the reference of a function, avoiding these re-renders if not necessary.
Example with useCallback
javascript
Other Optimization Strategies
In addition to the previous hooks and techniques, there are several additional strategies that can enhance the performance of a React application:
- Lazy Loading: Deferred loading of components, useful for improving initial load times.
- Code Splitting: Separating code into smaller parts and loading only what is necessary on each view.
- Avoiding Deep Rendering: Keep component structures as flat as possible.
Conclusion
Optimizing the performance of a React application is essential for a good user experience, especially in complex applications. In this chapter, we explored tools and techniques such as React Profiler, React.memo, useMemo, and useCallback to help you identify and resolve performance issues.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
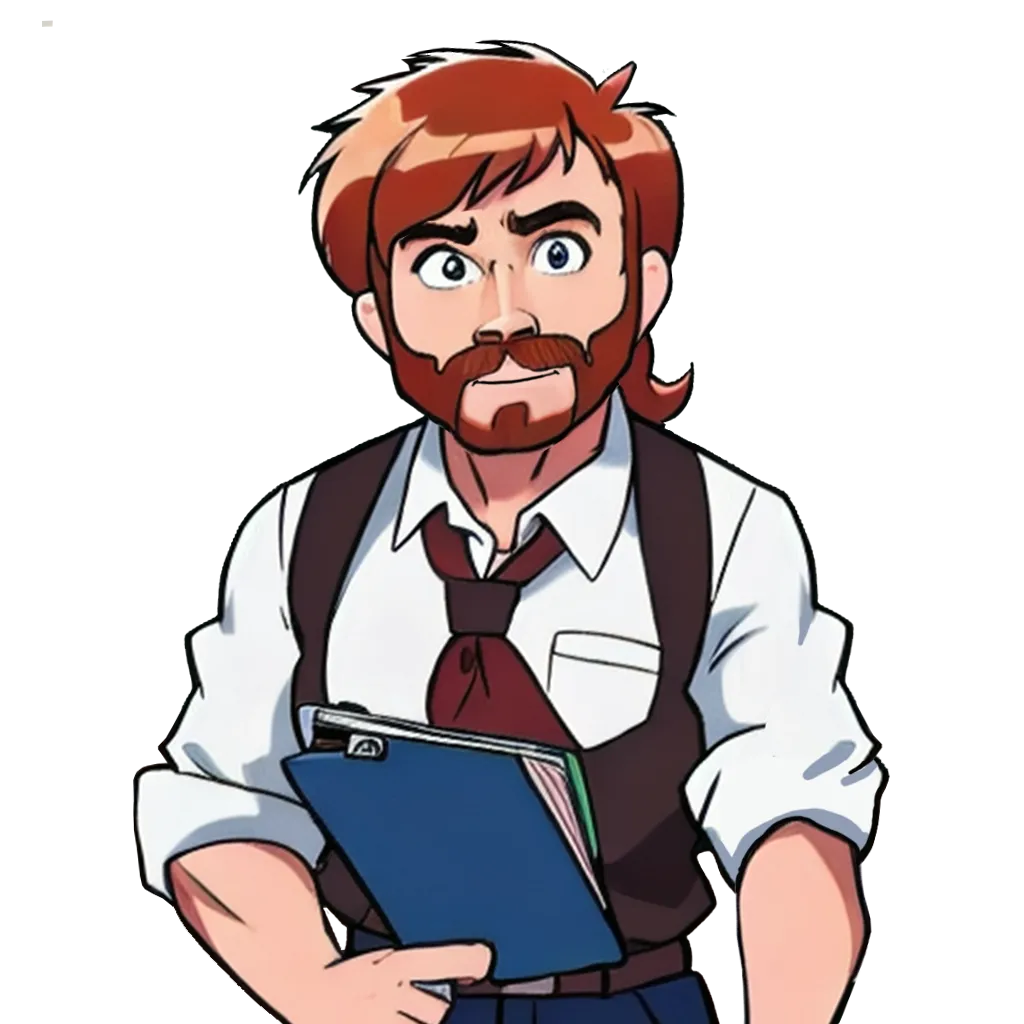