Intermediate React
Best Practices in React Component Architecture
The architecture of components in React is fundamental for developing scalable, maintainable, and high-performance applications. Good organization and structuring of components enable developers to keep the code clean and flexible as the application grows. In this chapter, we will explore the best practices for organizing components, managing state, and designing a solid architecture in React.
Component Design Principles in React
To create an efficient component architecture, it is important to follow some principles:
- Separation of concerns: Each component should have a single responsibility.
- Reusable components: Design components that can be reused in different parts of the application.
- Controlled data propagation: Keep data logic at the highest possible level and propagate only the necessary data.
Structuring Components: Smart and Dumb Components
Smart Components are responsible for handling the business logic and application state, while Dumb Components are presentational and only take care of the interface. This pattern allows presentation components to be reusable and easy to test.
Example of Smart and Dumb Components
In this example, the UserContainer
component manages user data, while UserProfile
displays the information:
javascript
Organization of Files and Folders
An organized folder structure facilitates the maintenance and scalability of React projects. A common approach is to organize files by type (components, hooks, contexts) or by specific features.
Example of Feature-based Organization
plaintext
In this structure, each feature or component has its own folder, keeping test files and related components together.
Self-contained Components and Modularization
A self-contained component includes its own styles, logic, and tests. This allows for greater modularization and reuse of components in different parts of the application without external dependencies.
Example of a Self-contained Component
javascript
Using Custom Hooks for Shared Logic
Custom hooks encapsulate logic that can be reused in various components. This helps keep the code clean and modular, especially in large applications.
Example of a Custom Hook for Handling API Data
javascript
Best Practices for Component Architecture
- Split into Small Components: Avoid monolithic components, as small components are easier to test and maintain.
- Use Context API for Global State: Implement Context API to handle shared data throughout the application, reducing excessive use of props.
- Encapsulate Logic in Custom Hooks: Use custom hooks to separate business logic from presentation components, keeping the code modular.
Conclusion
Component architecture is essential to maintain the efficiency and scalability of a React application. In this chapter, we explored architectural practices that include separation of concerns, file organization, and the use of custom hooks.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
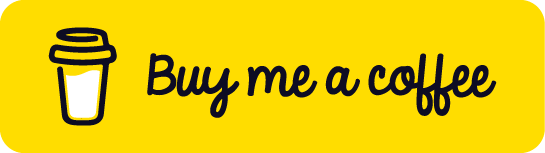
Chat with Chuck
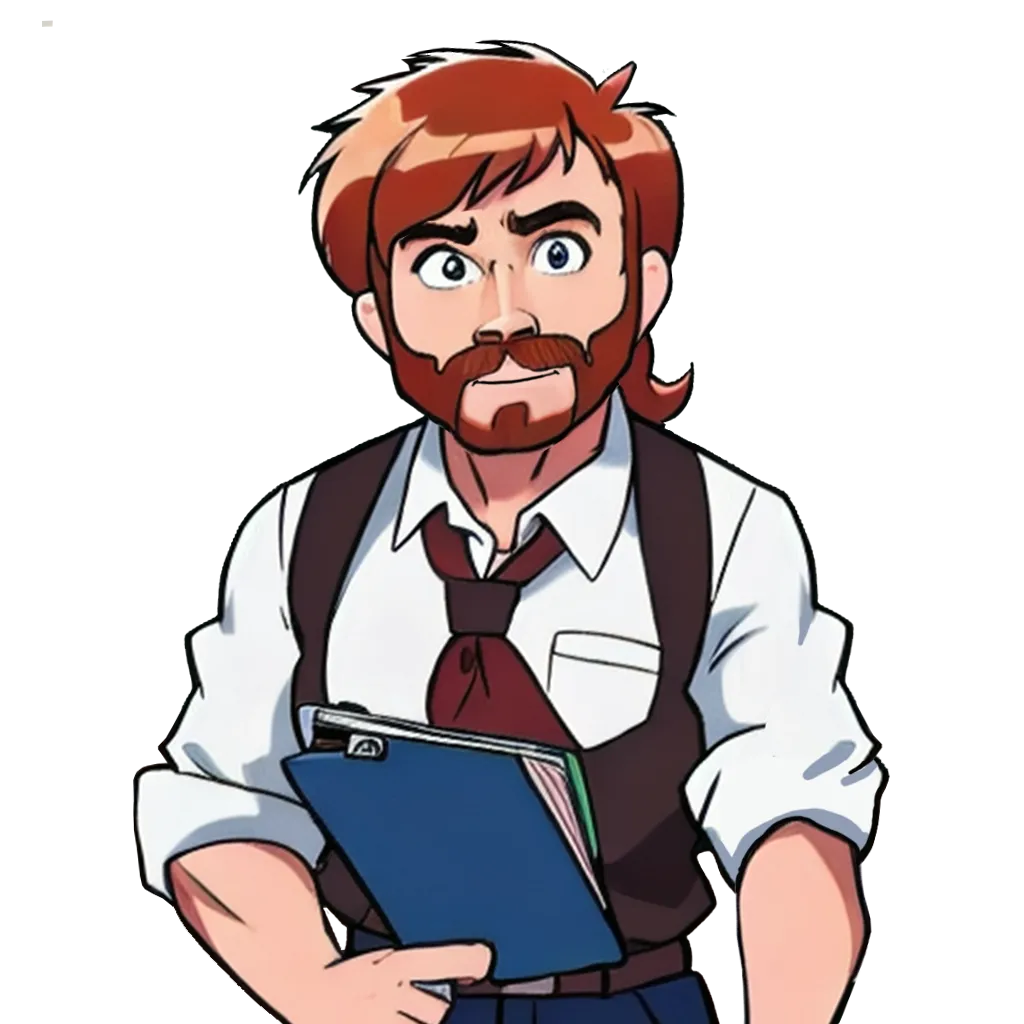
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React