Intermediate React
Integration with Animation Libraries
Animations play an important role in enhancing the user experience of web applications. In React, integrating animations can be effectively done using libraries like Framer Motion and React Spring. In this chapter, we will explore how to use these libraries to add attractive and smooth animations to our applications.
some animation libraries
Choosing Animation Libraries
There are many libraries to add animations in React, each with its own advantages. Below, we review two of the most popular libraries:
- Framer Motion: Offers a simple and powerful API for implementing declarative animations.
- React Spring: Based on realistic physics, it allows creating fluid and interactive animations.
Basic Animations with Framer Motion
Framer Motion is a library that simplifies creating animations with properties like initial
, animate
, and exit
. These properties allow defining the initial and final state of an animation.
Basic Example with Framer Motion
In this example, we use Framer Motion to animate a div
element that changes opacity and position when it appears:
javascript
Conditional Animations with React Spring
React Spring is a physics-based library that allows creating realistic animations using springs. In React Spring, animations can be defined using the useSpring
hook and configured according to conditions.
Example with React Spring for Show/Hide
In this example, we apply conditional animations to an element that is shown or hidden using a button:
javascript
Creating Complex Animations with Sequences in Framer Motion
Framer Motion allows creating animation sequences for complex components using variants
. This approach facilitates controlling multiple animations that occur in order.
Example of Animation Sequence
This example shows how to apply an animation sequence using Framer Motion variants:
javascript
Controlling Animations with useTransition in React Spring
The useTransition
hook in React Spring allows animating elements that enter and exit the screen, useful for dynamic lists and mounting effects.
Example with useTransition for Dynamic Lists
Here we show how to use useTransition
to animate list items when they are added or removed:
javascript
Best Practices for Animations in React
- Minimize the Use of Complex Animations: Complex animations can affect performance. Use them only when they enhance the user experience.
- Prefer Declarative Libraries: Framer Motion and React Spring make it easy to create declarative and optimized animations.
- Use Natural Transitions: Opt for smooth and natural transitions to enhance the perception and aesthetics of the interface.
Conclusion
Integrating animations in React using libraries like Framer Motion and React Spring allows creating attractive and dynamic interfaces. In this chapter, we explored how to use both libraries to add motion effects, from simple animations to complex sequences.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
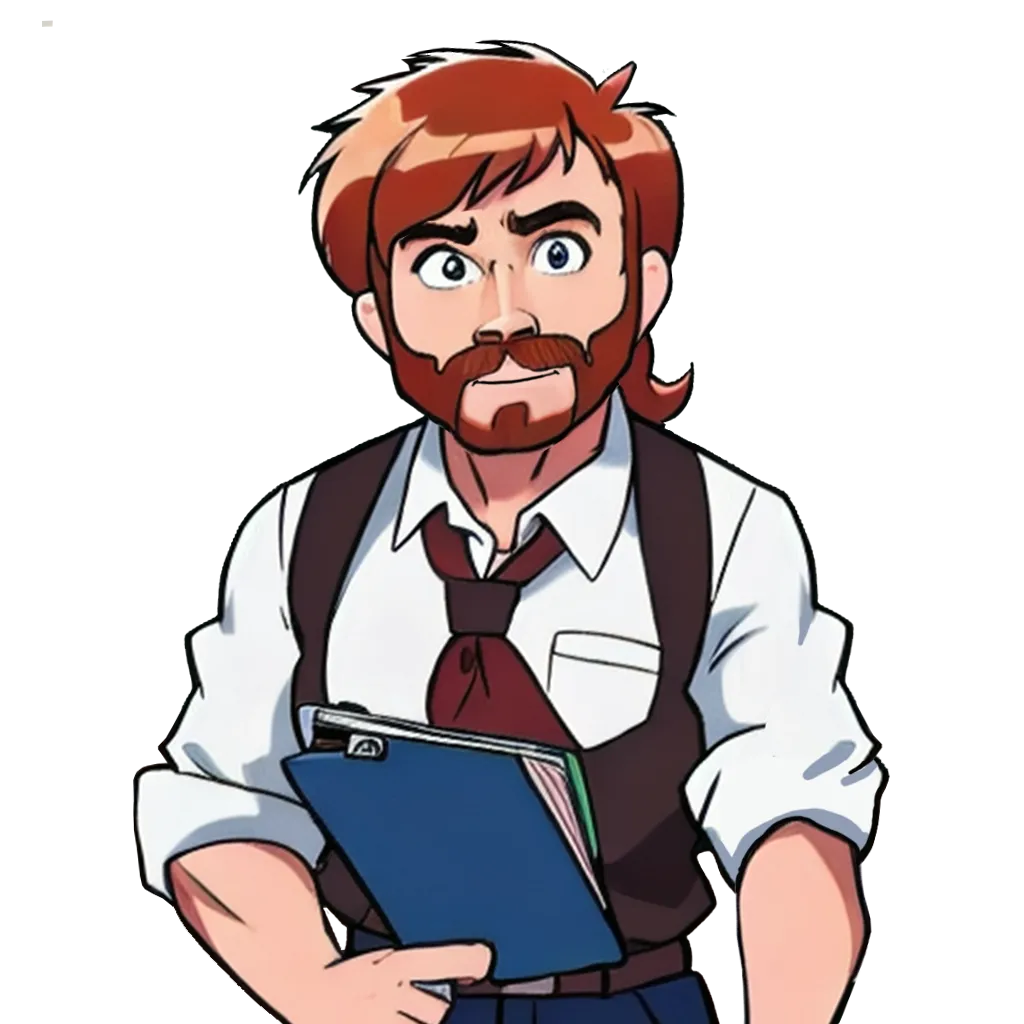