Intermediate React
Data Caching and Persistence Strategies in React
Data caching and persistence strategies are essential in modern applications to optimize performance and reduce the number of data requests. In React, there are various techniques and libraries that enable efficient data caching and persistence. In this chapter, we will explore how to implement caching and persistence strategies in React applications.
Introduction to Data Caching and Persistence
Cache is the temporary storage of data so that it can be reused without making repeated requests. Data persistence ensures that data remains available between user sessions, improving the experience by avoiding reloading the same data on each visit.
Cache Management Libraries
- React Query: Simplifies data fetching and caching in React applications.
- localStorage and sessionStorage: Store data in the browser persistently or temporarily.
- IndexedDB: A browser storage database for large volumes of data.
Implementing Cache with React Query
react query
React Query facilitates automatic data caching and revalidation, which is ideal for applications that require real-time data synchronization.
Basic Usage Example of React Query for Caching
In this example, we use useQuery
to fetch data from an API and automatically cache it:
javascript
Data Persistence in localStorage
localStorage allows persistent data storage in the browser, ideal for data that needs to be maintained across user sessions.
Example of Storing Data in localStorage
Here we create a custom hook useLocalStorage
to save and retrieve data from localStorage:
javascript
Implementing Cache Revalidation Strategies
Cache revalidation allows data to be updated when a certain time has passed, ensuring cached data is always up-to-date without making unnecessary requests.
Automatic Revalidation Example with React Query
React Query allows setting up automatic revalidation at specific time intervals:
javascript
Using IndexedDB for Large Volumes of Data
IndexedDB is a browser database that allows for structured storage of large volumes of data. It is useful for applications that handle large collections and need local storage without affecting performance.
Example of Using IndexedDB
Here we demonstrate how to open a connection and store data in IndexedDB:
javascript
Best Practices for Data Caching and Persistence
- Set Revalidation Intervals: Define appropriate time intervals to revalidate data, especially if it changes frequently.
- Persist Critical Data: Use localStorage or IndexedDB for important data that must be available between sessions.
- Minimize Cached Data Size: Avoid caching unnecessary large data and consider clearing the cache at certain times to free up memory.
Conclusion
Implementing data caching and persistence strategies in React greatly enhances efficiency and user experience in web applications. In this chapter, we explored how to use React Query, localStorage, and IndexedDB to cache and persist data in React.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
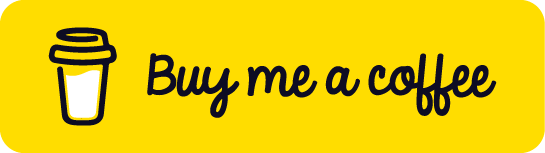
Chat with Chuck
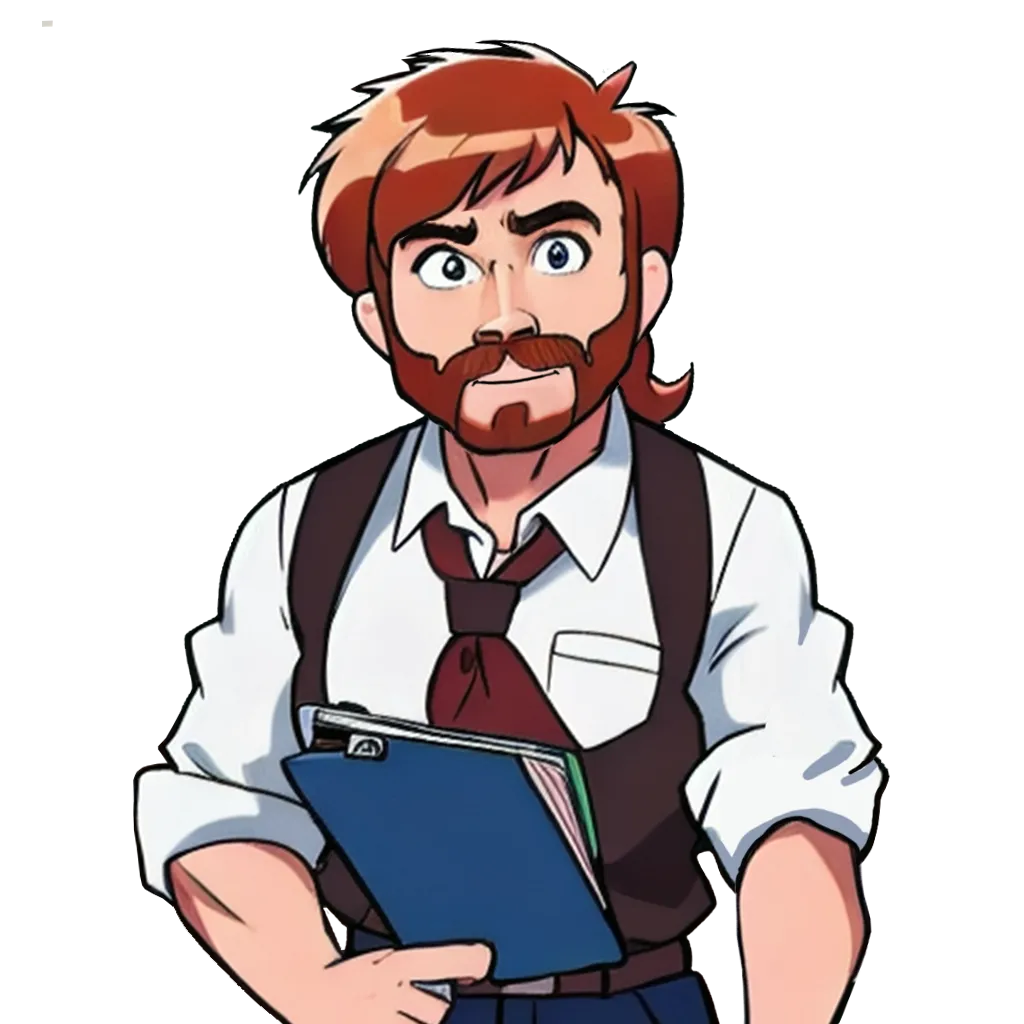
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React