Intermediate React
Advanced Performance Optimization in React
Optimizing performance in React is essential for applications that require efficiency and a smooth user experience. As applications grow, the need for advanced optimization techniques becomes more important. In this chapter, we will learn advanced strategies to optimize performance in React applications.
Identifying Performance Issues with React Profiler
React Profiler is a tool that allows measuring and analyzing the performance of components. It helps us identify components that render unnecessarily or take too long to update.
Example of Using React Profiler
To use React Profiler, we wrap the components to be analyzed:
javascript
react profiler
Avoiding Unnecessary Renders with React.memo and useMemo
React.memo and useMemo are techniques that help prevent unnecessary renders. React.memo
memoizes components, while useMemo
memoizes the result of expensive calculations.
Example with React.memo
This example uses React.memo
to memoize a list component:
javascript
Controlling Renders with useCallback
useCallback memoizes functions and prevents them from being recreated on every render. It is useful for optimizing components that depend on functions passed as props.
Example of Using useCallback
In this example, useCallback
is used to prevent recreating the increment function on every render:
javascript
Code Splitting with React.lazy and Suspense
Code splitting allows loading components in a deferred manner, improving the initial load time of the application. React.lazy
and Suspense
facilitate the deferred loading of components.
Example of Deferred Loading with React.lazy
Here, we use React.lazy
to load a component only when it is needed:
javascript
Optimizing Large Lists with Virtualization
For large lists, virtualization improves performance by rendering only the elements visible on the screen. Libraries like react-window help implement virtualization in large lists.
Example of Virtualization with react-window
Here, we use react-window
to optimize a large list:
javascript
Best Practices for Performance Optimization
- Avoid Unnecessary Renders: Use
React.memo
,useMemo
, anduseCallback
to reduce repetitive renders. - Efficient Code Splitting: Implement
React.lazy
andSuspense
to improve initial load and only load what is necessary. - Consider Virtualization in Lists: Apply virtualization in large lists to improve performance in applications that display large volumes of data.
Conclusion
Performance optimization is crucial to ensure that React applications work efficiently and quickly, even in complex situations. In this chapter, we explored advanced techniques such as React Profiler, memoization, and virtualization.
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React
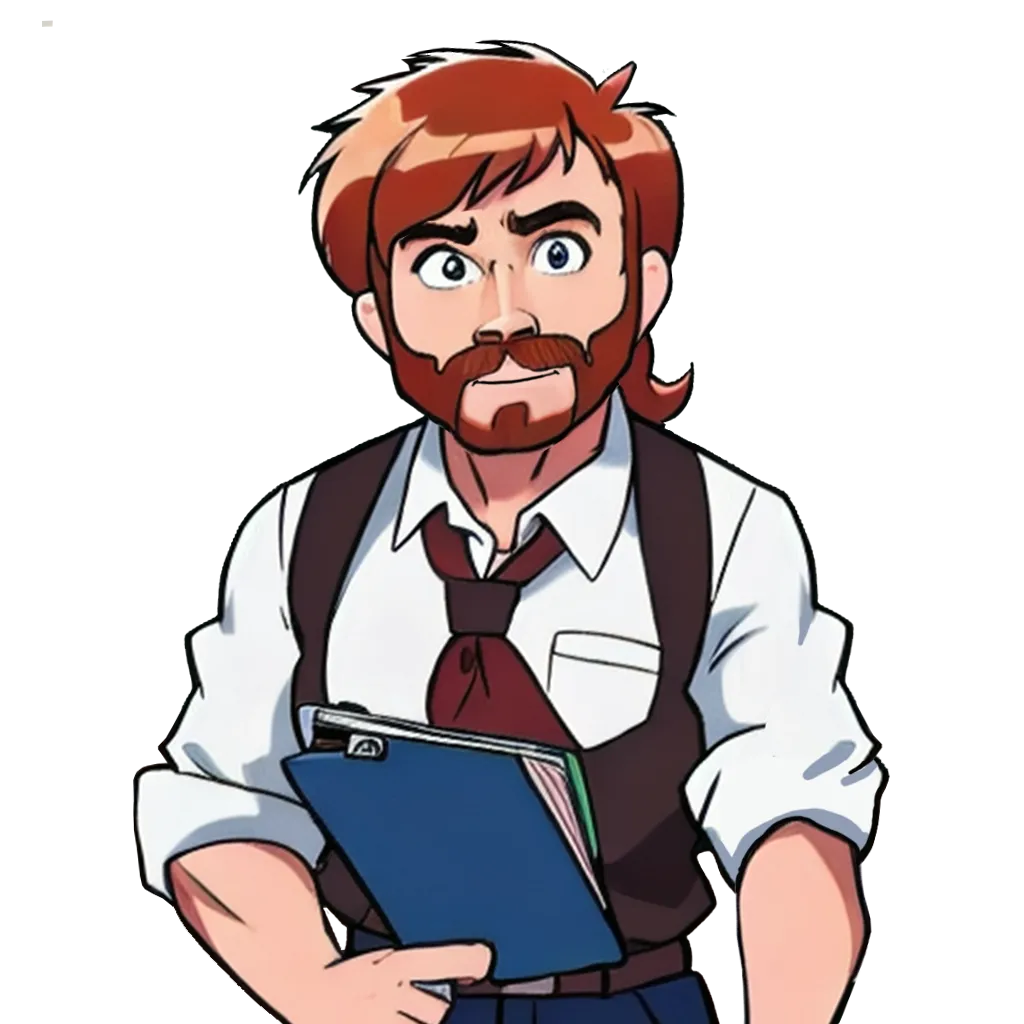