Intermediate React
Suspense Implementation for Data Fetching
Handling asynchronous data in React is crucial for creating modern and dynamic applications. React introduces the Suspense component to help manage the data loading process efficiently and provide a better user experience during the wait. In this chapter, we will learn how to use Suspense in the context of data fetching and look at some practical cases.
suspense data fetching
What is Suspense in React?
Suspense is a React component that allows displaying a waiting interface while loading asynchronous resources, such as data from an API. This functionality improves the user experience by preventing incomplete or unwanted elements from being displayed during the loading time.
Basic Implementation of Suspense
To use Suspense in data loading, we need an "asynchronous resource." Although Suspense does not directly support promises, data handling libraries or tools like react-query
or Relay
can be used. We can also simulate the behavior of Suspense using a basic resource in this example.
Example of Suspense with a Loading Component
javascript
Suspense for Data Loading with Libraries
Libraries like react-query
or Relay
can work with Suspense to simplify the loading and management of asynchronous data. Using react-query
, for example, we can combine data loading hooks with Suspense to improve user experience and performance.
Example with Suspense and React Query
In this example, we use Suspense
and react-query
to handle data loading and display a message while awaiting the response:
javascript
Creating Asynchronous Resources with Suspense
For situations where data management libraries are not used, a custom resource function can be created to simulate the behavior of Suspense in data loading.
Example of a Custom Asynchronous Resource
Here we create a resource that simulates data loading and wrap it in Suspense:
javascript
Good Practices with Suspense
- Use Suspense at High-Level Components: Place Suspense at high-level components to avoid multiple loading messages simultaneously on the same page.
- Combine with Data Management Libraries: Use libraries like
react-query
orRelay
that natively support Suspense to simplify implementation. - Provide Informative Fallbacks: The waiting messages in
fallback
should be informative and appealing to improve the user experience during loading.
Conclusion
Suspense in React allows effective data loading management, displaying waiting messages and improving the user experience. In this chapter, we explored how to use Suspense for data fetching, including basic and advanced examples.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
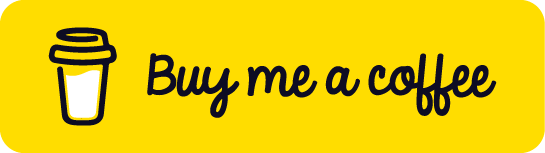
Chat with Chuck
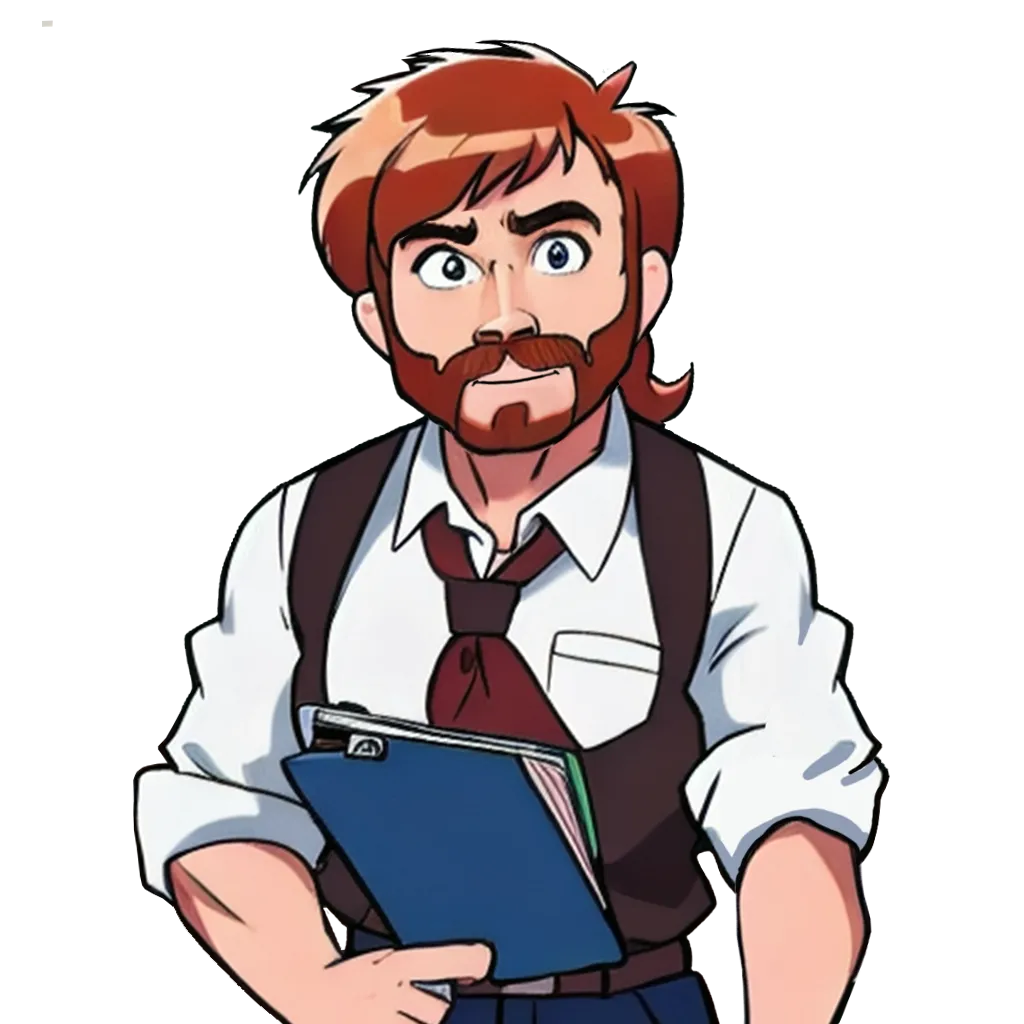
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React