Intermediate React
React.memo and useMemo for Performance Improvement
As React applications grow, performance optimization becomes a necessity to avoid unnecessary renders and improve user experience. In this chapter, we will explore how to use React.memo and the useMemo hook to optimize components and expensive calculations in React applications.
react memo vs usememo
What is React.memo?
React.memo is a function that memoizes functional components. This means React will only re-render the component if the properties (props) change. It is especially useful in components that receive large amounts of data or those that render frequently.
Example of React.memo in Functional Components
In this example, we use React.memo
to optimize a list component that displays items:
javascript
Limitations of React.memo
Although React.memo
optimizes performance by avoiding unnecessary renders, there are cases where it may not be useful:
- Referential props: If the properties change in reference but not in content (like a recreated array), the component will re-render.
- Comparison costs: In some cases, the comparison of the properties may be as costly as the rendering itself.
Optimizing Expensive Calculations with useMemo
The useMemo
hook allows you to memoize the result of expensive calculations, executing the function only when a dependency changes. This is useful in cases where a calculation relies on props or states that change infrequently.
Basic Example of useMemo
In the following example, we use useMemo
to memoize the result of an intensive calculation function:
javascript
Combined Cases: React.memo and useMemo
In complex components that receive many props, React.memo
and useMemo
can be used in combination to optimize both the component rendering and internal calculations. This combination is particularly useful in applications that handle large volumes of data or lists.
Example of React.memo with useMemo
Here we show how React.memo
and useMemo
can work together in a component that calculates complex values for each item in a list:
javascript
Best Practices When Using React.memo and useMemo
- Use in Heavy Components: Apply
React.memo
to large components or those that receive many props. - Be Careful with Dependencies in useMemo: Ensure that
useMemo
dependencies are correctly defined to avoid reference errors. - Measure the Impact: Use tools like React Profiler to identify if any performance gains are significant.
Conclusion
react memo vs usememo.png
React.memo
and useMemo
are powerful tools for optimizing component performance and calculations in React. In this chapter, we explored how and when to use these optimizers to avoid unnecessary renders and calculations. In the next chapter, we will dive into the use of Suspense for data fetching and handling asynchronous data loading.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
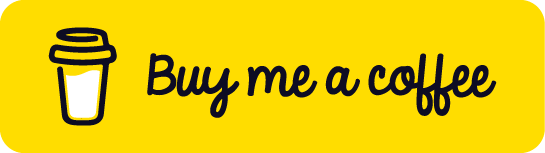
Chat with Chuck
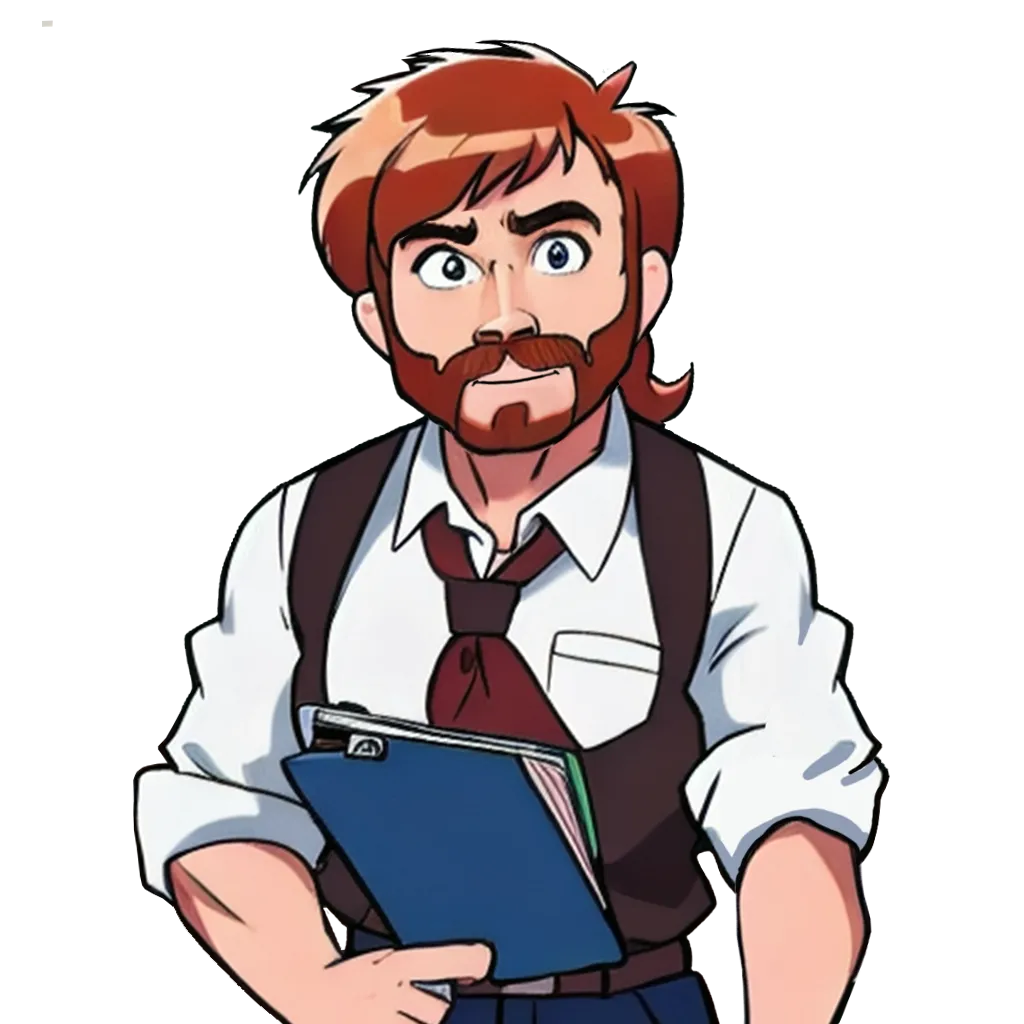
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React