Intermediate React
Creation of Reusable React Components
Creating reusable components is a key skill for developing scalable and efficient React applications. Reusable components help reduce duplicate code, improve interface consistency, and make project maintenance easier. In this chapter, we will explore practices and patterns for designing and developing components that can be reused in different contexts and projects.
Principles of Reusable Component Design
To create reusable components, it is important to follow certain principles:
- Generalization: Components should be flexible and adapt to different scenarios.
- Decoupling: A component should avoid depending on specific contexts to facilitate its use elsewhere.
- Configurability: Allow component users to configure its properties and style.
Using Props for Component Configuration
Props allow you to configure and customize a component, making it adaptable to different situations. Through props, components can accept varied information without changing their structure.
Example of Reusable Button with Props
In this example, we design a reusable button that accepts props to configure its text and style:
javascript
Implementing Composition in Components
Composition is a technique that allows the construction of complex components from smaller, specialized components. This helps create modular and highly reusable user interfaces.
Example of Composition with a Card Component
In this example, we create a Card
component that accepts children components and becomes a reusable container for different content:
javascript
Using Children and Render Props for Flexibility
The use of children
and Render Props allows components to accept dynamic structures, offering flexibility to component users without needing to modify their internal code.
Example of Render Props in a Modal Component
In this example, a Modal
component uses Render Props to customize its content according to user needs:
javascript
Designing Controlled and Uncontrolled Components
Controlled components rely on an external state, whereas uncontrolled components maintain their own internal state. The choice depends on the level of control and flexibility needed.
Example of Controlled and Uncontrolled Component
Here, we create a controlled input and an uncontrolled one to illustrate their differences:
javascript
Best Practices for Reusable Components
- Allow Configuration through Props: Design components to accept configurations through props rather than relying on internal data.
- Use Render Props for Flexibility: Implement Render Props to allow component users to customize their content.
- Define Styles in Self-contained Components: Keep styles within the component to enhance its modularity and avoid external dependencies.
Conclusion
Creating reusable components is essential for building scalable and flexible applications in React. In this chapter, we explored techniques such as using props, composition, Render Props, and differentiating between controlled and uncontrolled components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
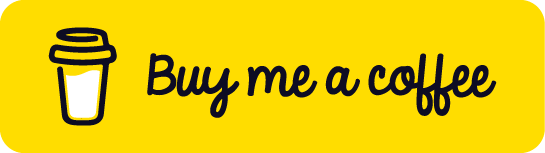
Chat with Chuck
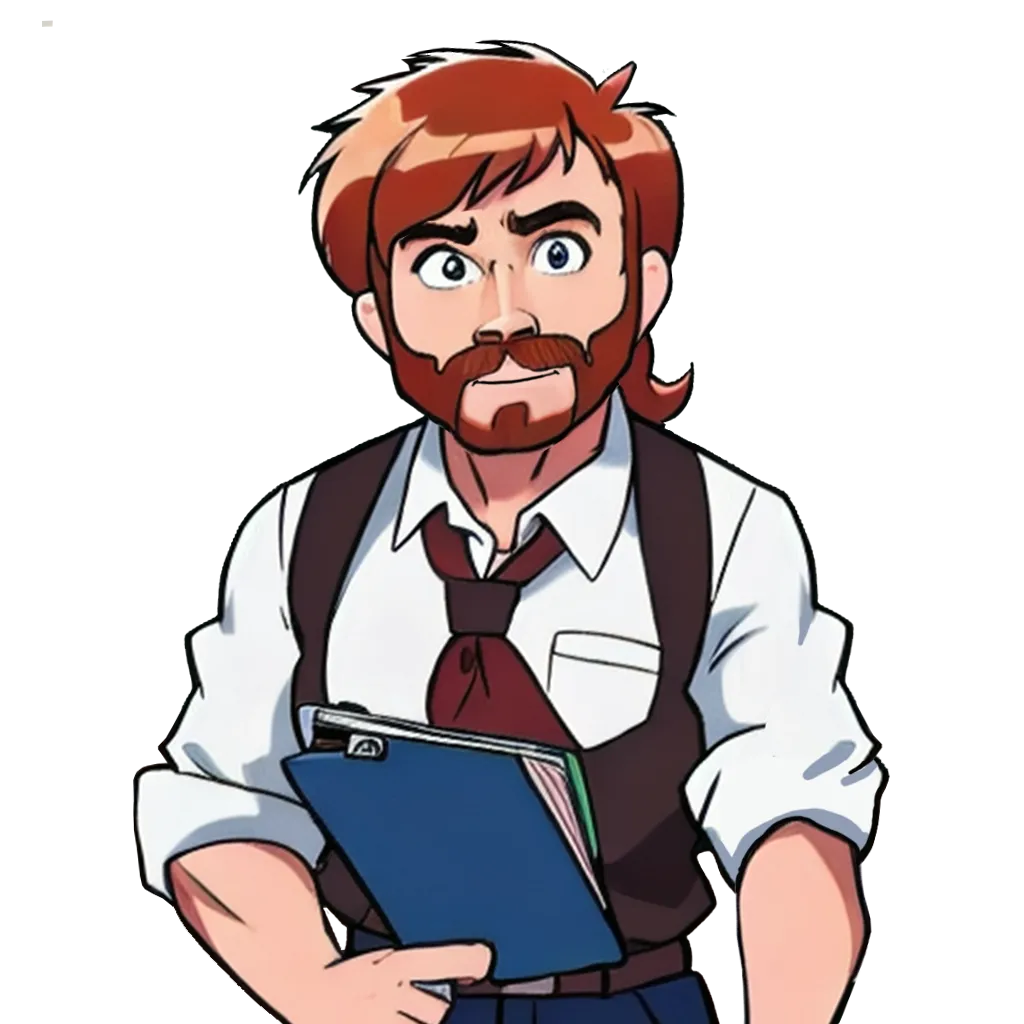
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React