Intermediate React
Error Handling in Components with Error Boundaries
In React applications, error handling is crucial to prevent failures in individual components from impacting the user's overall experience. In this chapter, we will learn how to use Error Boundaries to capture and handle errors in React components, ensuring a smooth user experience even when unexpected errors occur.
What are Error Boundaries?
Error Boundaries are components that act as "error boundaries" in the application. They allow capturing JavaScript errors in the lifecycle of child components, without affecting other components or the main application tree. Error Boundaries are useful for handling exceptions in specific components and displaying an alternative interface or custom error messages.
Creating a Basic Error Boundary
To create an Error Boundary, define a class component that implements the componentDidCatch
method and the getDerivedStateFromError
method. This component captures errors and can update its state to display an alternative interface in case of failures.
Example of an Error Boundary
javascript
Using an Error Boundary in the Application
To use an Error Boundary, wrap the components you want to protect:
javascript
Enhancing User Experience with Custom Error Messages
Error Boundaries allow displaying custom error messages to enhance the user experience. This is especially useful for applications that require clear communication in case of failure.
Example of Error Boundary with Custom Message
We can modify the Error Boundary to display a dynamic error message or even action buttons for the user to attempt to recover functionality:
javascript
Custom ErrorBoundary
Error Boundaries and Asynchronous Errors
Error Boundaries only capture JavaScript errors in the component lifecycle and rendering. They do not capture asynchronous errors in promises or async/await
functions. To handle asynchronous errors, you can implement custom error handlers or use try/catch
in asynchronous methods.
Example of Handling Asynchronous Errors
If a component uses asynchronous functions to fetch data, it is recommended to wrap the logic in try/catch
blocks:
javascript
Best Practices for Using Error Boundaries
- Use Error Boundaries in Critical Components: Apply Error Boundaries in key components or those more likely to generate errors, such as data visualization components.
- Provide Recovery Options: Offer options like retry buttons or redirects to enhance the user experience in case of an error.
- Log Errors: When using
componentDidCatch
, log the errors to a monitoring system for tracking and improvement.
Conclusion
Error Boundaries in React are essential for capturing and handling errors efficiently, maintaining application stability. In this chapter, we explored how to implement Error Boundaries and handle asynchronous errors to create more robust applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
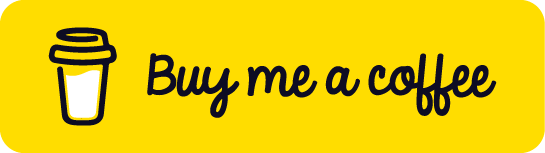
Chat with Chuck
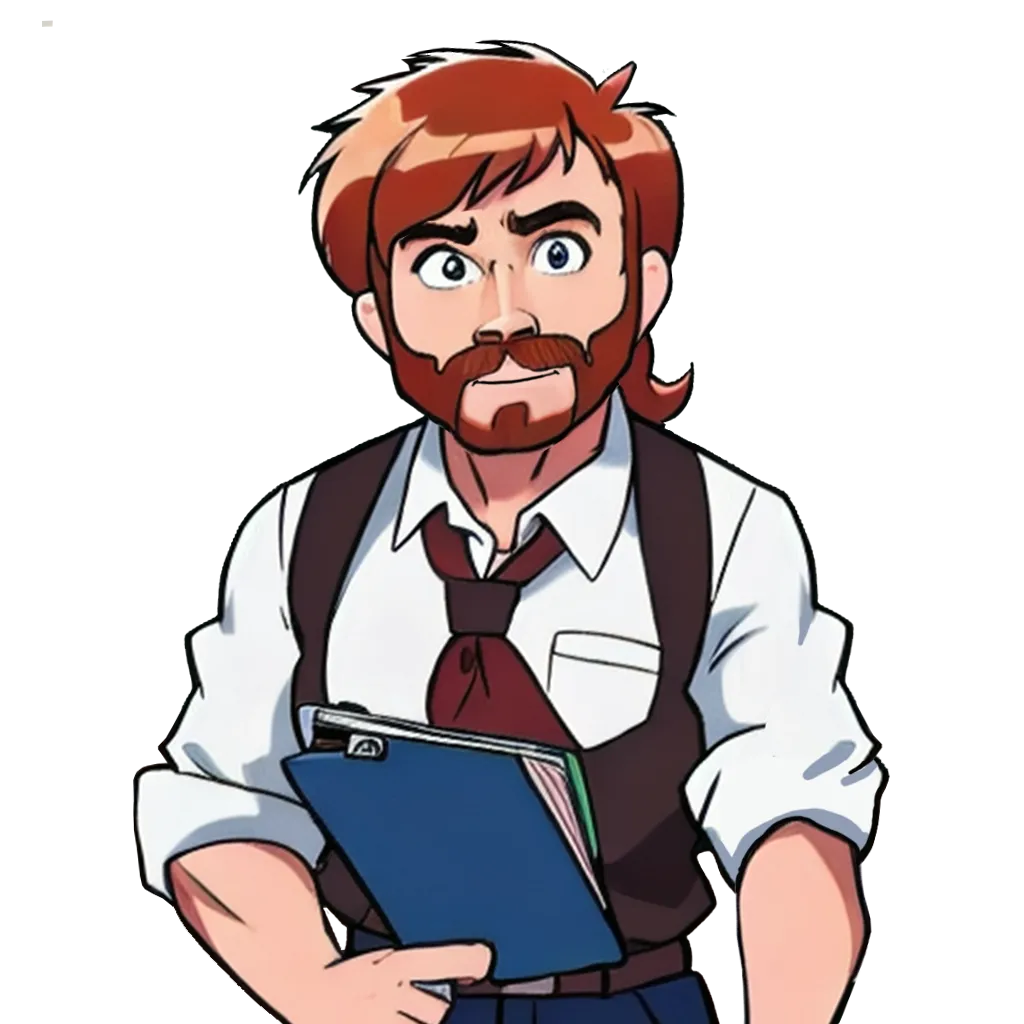
- Advanced State Management with Context API and useReducer
- Performance Optimization in React
- Lazy Loading and Code Splitting
- React Profiler and Performance Analysis
- Context API y State Management Escalable
- Render Props and Higher-Order Components (HOC)
- Error Handling in Components with Error Boundaries
- Refs and Direct DOM Manipulation
- React.memo and useMemo for Performance Improvement
- Suspense Implementation for Data Fetching
- Complex Component Communication
- Advanced Conditional Rendering
- Integration with Animation Libraries
- Advanced Custom Hooks Patterns
- Data Handling and RESTful APIs in React
- Data Caching and Persistence Strategies in React
- Accessibility Management in Interactive Components
- Advanced Performance Optimization in React
- Testing Components with Mocking and Integration Tests
- Best Practices in React Component Architecture
- Creation of Reusable React Components
- Conclusions and Next Steps in Advanced React