Basic TypeScript
Advanced Typing in TypeScript
TypeScript provides a wide range of advanced tools for working with types. Among them, we find conditional types, mapped types, and other mechanisms that allow for more flexible and safer code. In this chapter, we'll explore how to use these advanced typing features.
Union and Intersection Types
This image shows an illustration of differences between union and intersection
Union Types
Union types allow a variable to have more than one type. This is useful when a variable can take different forms or values.
typescript
Intersection Types
Intersection types combine multiple types into one. An object using an intersection type must have all the properties of the combined types.
typescript
Conditional Types
Conditional types allow creating types that depend on a condition. The basic syntax of a conditional type is T extends U ? X : Y
.
Conditional Types Example
typescript
Mapped Types
Mapped types allow transforming an existing type into another, applying changes to each of its properties. This is useful for creating modified versions of complex types.
Mapped Type Example
typescript
Indexed Types
Indexed types allow accessing the types of an object's properties. This is useful when you want to reuse a type in different places.
Indexed Type Example
typescript
Conclusion
In this chapter, we have explored some of the advanced typing features in TypeScript, including union and intersection types, conditional, mapped, and indexed types. These tools enable writing more sophisticated and secure code, especially in large and complex projects.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
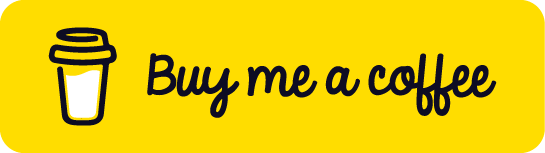
Chat with Chuck
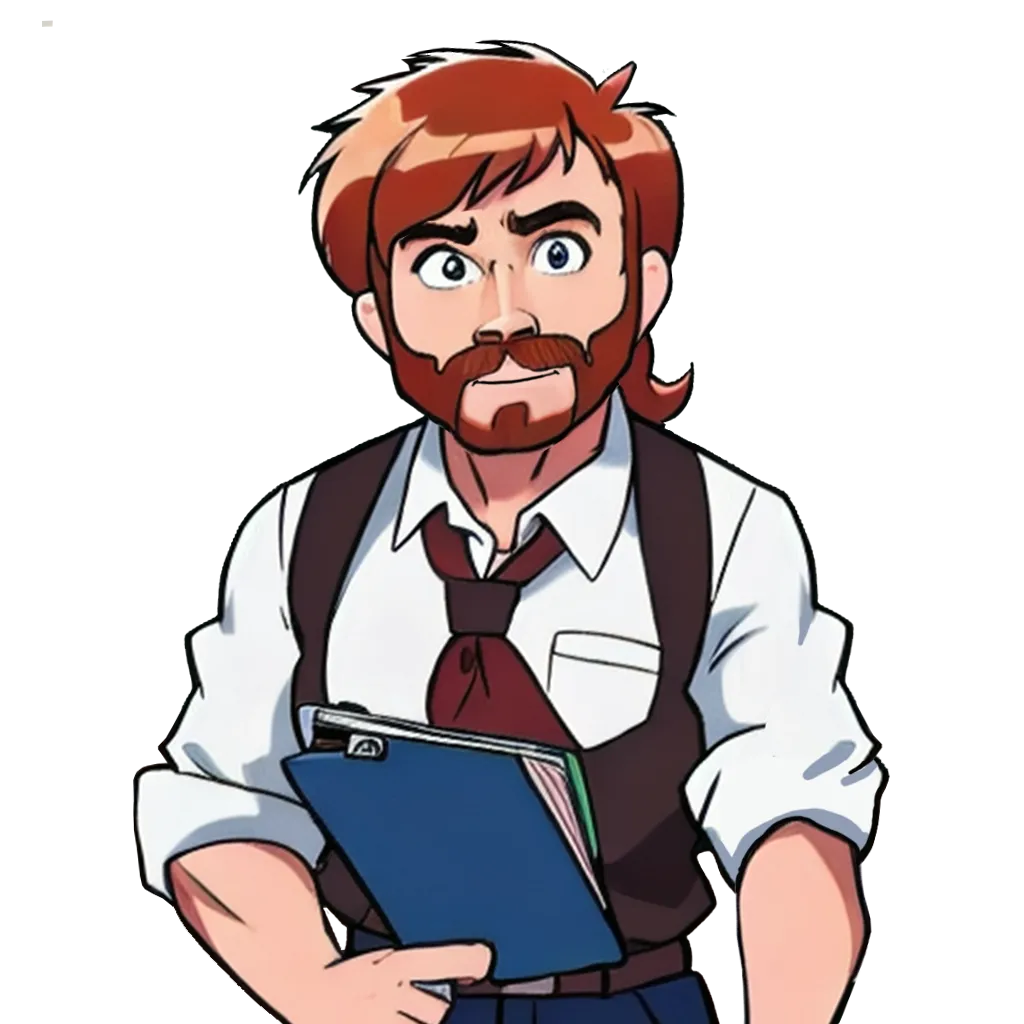
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps