Basic TypeScript
Interfaces and Types in TypeScript
In TypeScript, interfaces and types are powerful tools that allow you to define clear and precise data structures. These structures help make the code more maintainable and easier to understand, as they explicitly define what types of values can be expected by functions, classes, and other elements.
Defining Interfaces
An interface in TypeScript is a contract that defines the shape that an object should have. It does not specify implementations, just the structure. Let's see a basic example:
typescript
Extending Interfaces
TypeScript allows interfaces to be extended, which means an interface can inherit properties from another and add its own. This is useful when you want to create more complex structures based on simpler ones.
typescript
Defining Types
In addition to interfaces, TypeScript allows you to define custom types using the type
keyword. Types can be used to create more flexible structures or aliases for complex types.
typescript
Type Aliases
Type aliases are useful to simplify complex or repetitive types, making the code clearer and easier to read.
typescript
Interfaces vs Types
While interfaces and types are similar in many respects, there are some key differences:
- Interfaces: They are more appropriate for describing objects and can be extended.
- Types: They are more flexible since they can describe more complex structures, such as union types and type aliases.
This image shows a comparison between interfaces and types
In most cases, you can choose which to use based on your needs. TypeScript allows you to mix interfaces and types within the same project.
Conclusion
In this chapter, we have learned to use interfaces and types in TypeScript to define more clear and precise data structures. Interfaces are ideal for describing the shape of objects, while types give us additional flexibility with aliases and union types.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
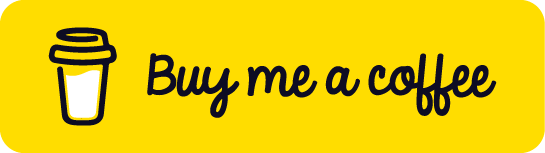
Chat with Chuck
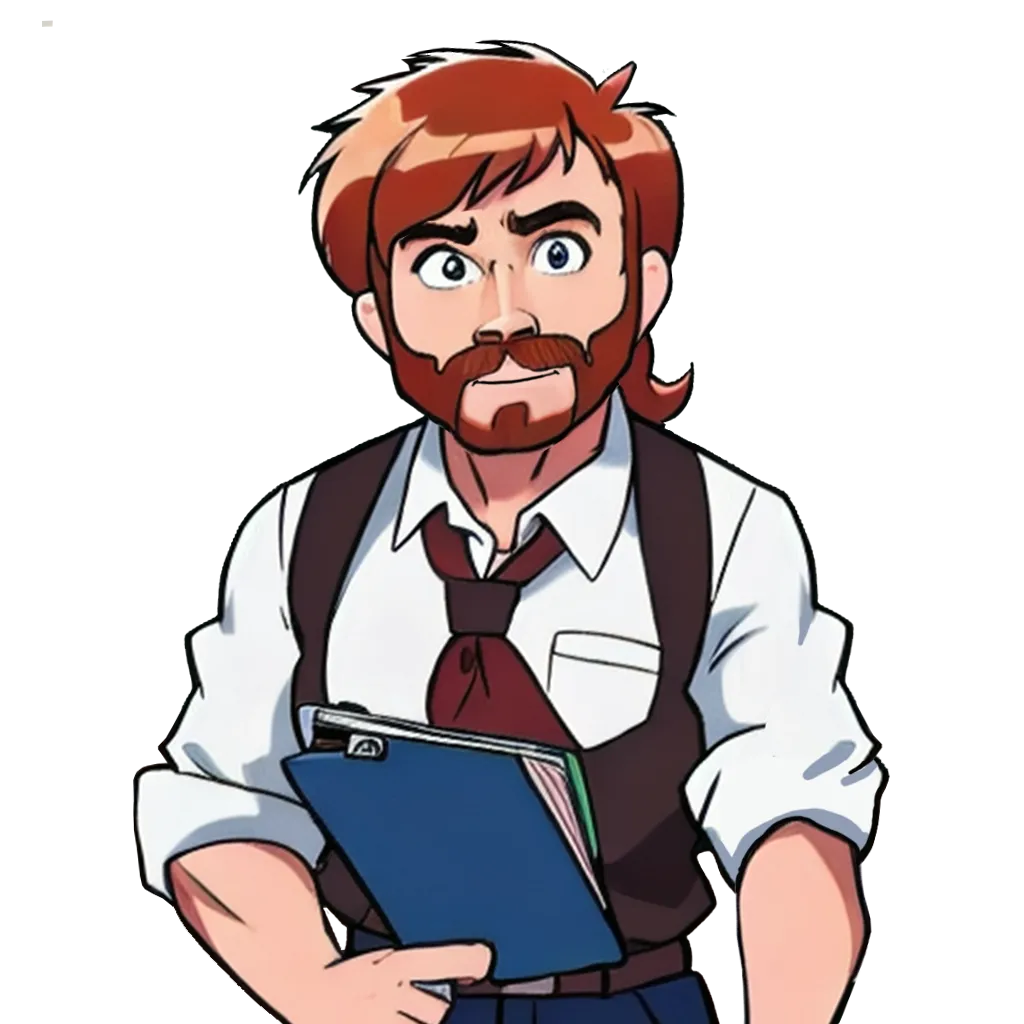
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps