Basic TypeScript
TypeScript in Node.js
Node.js is a popular platform for developing backend applications and web services. Using TypeScript in Node.js enhances the development experience by adding static typing, autocompletion, and error checking during development time. In this chapter, we will learn how to set up a Node.js project with TypeScript and how to use TypeScript to develop secure and scalable backend applications.
This image shows a node js plus typescript logo
Setting Up a Node.js Project with TypeScript
To start, you need to initialize a Node.js project with support for TypeScript. First, create a folder for the project and run the following command to initialize npm
:
bash
Installing TypeScript and Node Types
Then, install TypeScript and the type definitions for Node.js using npm:
bash
Setting Up the tsconfig.json
File
After installing TypeScript, you need to set up the tsconfig.json
file to define how TypeScript code should be compiled in Node.js. Run the following command to generate the tsconfig.json
file:
bash
Next, edit the tsconfig.json
file to ensure it is correctly configured for Node.js:
json
Creating a Server with TypeScript
Once the project is set up, we can create a basic server using TypeScript and Node.js APIs.
Example of an HTTP Server with TypeScript
Create a file named index.ts
in the src
folder and write the following code to create a basic HTTP server:
typescript
Compiling and Running the Server
To compile the TypeScript code, execute the following command:
bash
Then, you can run the server using Node.js:
bash
Using Express with TypeScript
Express is one of the most widely used frameworks for building web applications and APIs in Node.js. With TypeScript, we can correctly type the controllers and middlewares of Express, which helps us avoid common errors.
Installing Express and Express Types
First, install Express and the corresponding type definitions:
bash
Example of an Express Server with TypeScript
Next, create a file server.ts
in the src
folder and write the following code to create an Express server with TypeScript:
typescript
Typing Request Parameters in Express
With TypeScript, we can type the parameters of a request to ensure we are handling the data correctly. Here is an example of how to do it with a route that receives dynamic parameters:
typescript
Typing Middlewares in Express
Middlewares in Express can benefit from TypeScript typing, making it easier to create reusable and secure middlewares.
Example of Middleware with TypeScript
typescript
Conclusion
In this chapter, we learned how to configure and develop Node.js applications with TypeScript. We saw how to set up a basic HTTP server, how to integrate Express with TypeScript, and how to leverage static typing in controllers and middlewares. TypeScript in Node.js provides a safer and more productive development experience.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
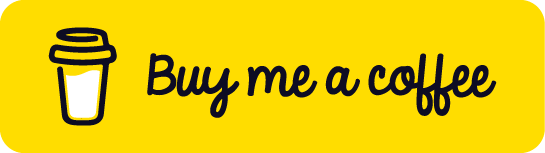
Chat with Chuck
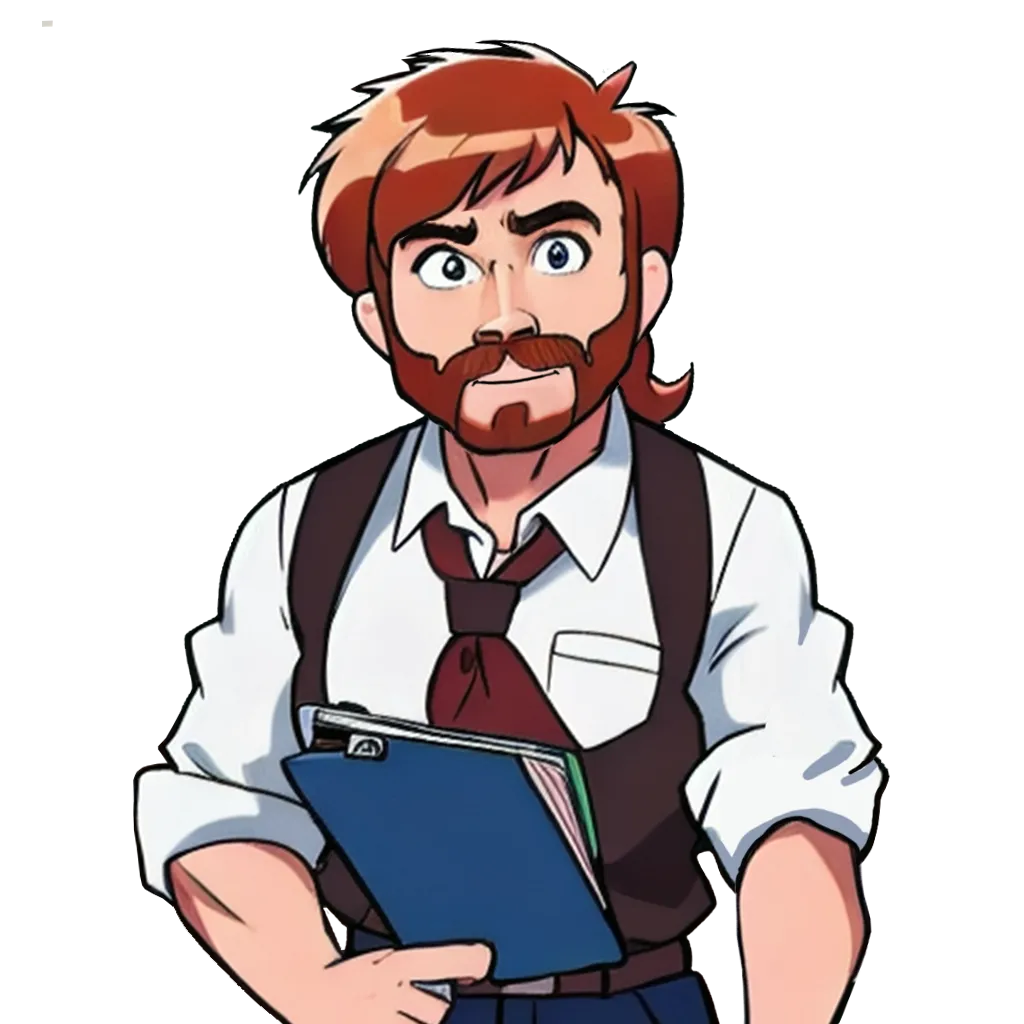
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps