Basic TypeScript
Functions in TypeScript
Functions are one of the fundamental pillars in any programming language, and TypeScript adds typing to make functions more secure and predictable. In this chapter, we will learn how to type parameters, return values, and how to handle optional functions and default parameters.
Typing Parameters and Return
In TypeScript, you can specify the type of parameters a function receives, as well as the type of value it returns. This ensures that the function is used correctly and that its behavior is predictable.
Example of Typing Parameters and Return
typescript
If you try to pass values that are not numbers, TypeScript will generate an error:
typescript
Optional Parameters
Just like with optional properties in objects, TypeScript allows function parameters to be optional. This is achieved by adding the symbol ?
after the parameter name.
Example of Optional Parameters
typescript
Default Parameters
TypeScript also allows you to define default values for parameters. If a value is not provided, the parameter will take the default value.
Example of Default Parameters
typescript
Anonymous and Arrow Functions
TypeScript supports anonymous functions and arrow functions, which are a more concise way to write functions. Typing can also be applied to these functions.
Example of Arrow Function
typescript
Conclusion
In this chapter, we have explored how to use functions in TypeScript, from defining types for parameters and return values to using optional and default parameters. Additionally, we saw how to work with arrow functions, which are a concise and modern way to write functions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
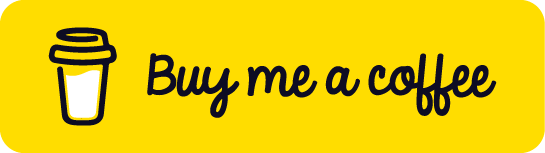
Chat with Chuck
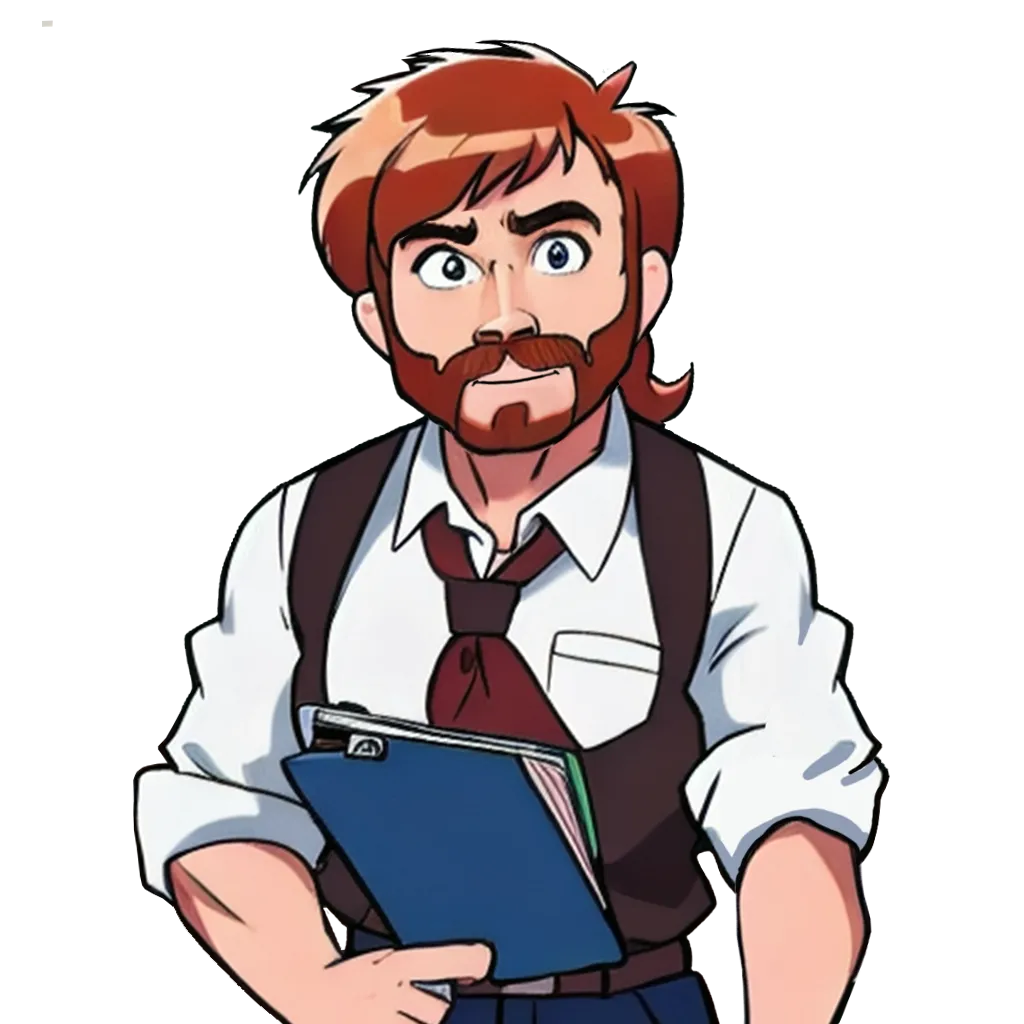
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps