Basic TypeScript
TypeScript in React
TypeScript has become a powerful tool for developing React applications. Using TypeScript with React not only enhances the development experience by adding static typing and autocompletion but also helps prevent errors before they occur. In this chapter, we will learn how to set up a React project with TypeScript and how to correctly type components, props, and more.
This image shows a logo of TypeScript plus React
Setting Up a React Project with TypeScript
To start, we can create a new React project with TypeScript support using create-react-app
. The following command generates a React project template configured for TypeScript:
bash
This command generates a project in the my-app
folder with the necessary configuration files to work with TypeScript in React.
Project Structure
Once the project is generated, you will see a folder structure that includes a tsconfig.json
file and several .tsx
files. The .tsx
files are similar to .jsx
files in JavaScript but allow the use of TypeScript with JSX.
Typing Functional Components
In React, functional components are the preferred way to create components. TypeScript allows typing the props these components receive, ensuring that the data passed to the components is correct.
Example of Typed Functional Component
typescript
Using the Component
typescript
Typing Optional Props
TypeScript also allows defining optional props in components. This is useful when some props are not necessary in all situations.
Example of Optional Props
typescript
Typing States with useState
The useState
hook is widely used in React to handle component state. In TypeScript, we can type both the state and the function that updates it.
Example of Typed useState
typescript
Typing Refs with useRef
The useRef
hook allows direct access to DOM elements in functional components. We can also type useRef
in TypeScript to ensure we always reference the correct type of element.
Example of Typed useRef
typescript
Typing Components with Children
In React, many components accept child elements (children) as part of their props. TypeScript allows typing these children using the property React.ReactNode
.
Example of Component with Children
typescript
Using Context API with TypeScript
React Context API is used to share data between components without the need to manually pass props. We can type the context to ensure that the data we share is always of the expected type.
Example of Typed Context API
typescript
Conclusion
In this chapter, we have learned to use TypeScript with React, including how to type functional components, props, states, and contexts. TypeScript provides us with additional safety when working with React applications, helping to avoid errors and improve productivity.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
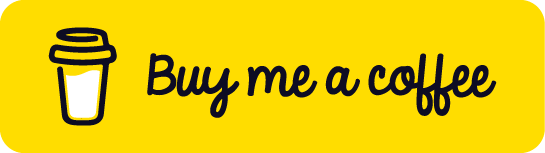
Chat with Chuck
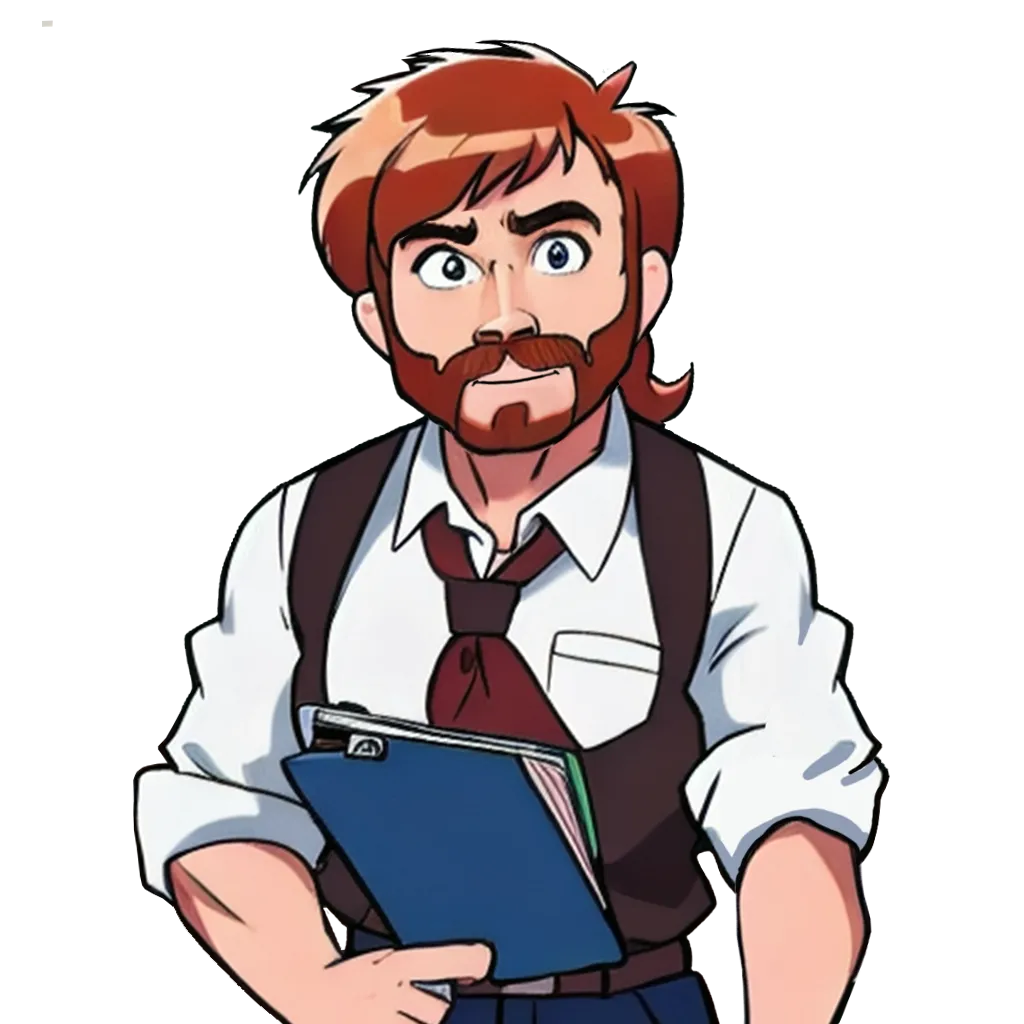
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps