Basic TypeScript
Conclusions and Next Steps
Throughout this course, we have explored the capabilities of TypeScript in depth, from the basics to advanced practices that help improve code quality and maintainability. TypeScript not only enriches development with static typing, but also offers tools to prevent errors, improve productivity, and ensure application scalability.
Summary of What We've Learned
Advantages of Using TypeScript
TypeScript provides several key advantages over JavaScript, such as:
- Static typing: Reduces runtime errors by detecting issues during compilation.
- Enhanced autocomplete and tools: Improves productivity through code suggestions and error checking as you write.
- Full compatibility with JavaScript: Allows for a gradual migration of existing JavaScript projects.
- Support for modern ECMAScript features: Facilitates the use of the latest ECMAScript features while ensuring backward compatibility.
Patterns and Best Practices
We've learned to implement best practices and common patterns in TypeScript, such as:
- Use of interfaces and types: Clear definition of data structures to avoid common mistakes.
- Immutability and strict typing: Enhances code safety and predictability.
- Design patterns like Singleton, Factory, and Decorator: To better structure the code and promote reuse.
Using TypeScript in React and Node.js
We also saw how to integrate TypeScript with popular frameworks in the JavaScript ecosystem, such as React and Node.js, allowing for the creation of more robust and maintainable frontend and backend applications.
Next Steps
TypeScript is a constantly evolving tool. Now that you master the fundamentals and advanced practices, there are several ways you can continue enhancing your skills and deepening your use of TypeScript:
Contribute to Open Source Projects
Contributing to open source projects is an excellent way to learn more about TypeScript and collaborate with other developers. Many open source projects use TypeScript and are always looking for contributions.
Explore Advanced Frameworks
After getting familiar with TypeScript, you can explore advanced frameworks that make full use of TypeScript, such as:
- NestJS: A framework for building scalable backend applications.
- Next.js: A framework for React that facilitates the development of web applications with server-side rendering.
Enhance Project Configuration
As you work with TypeScript in larger projects, you can investigate:
- Advanced
tsconfig.json
settings: Adjust compilation options to optimize performance. - Incremental compilation and build optimization: Improve compilation speed in large projects.
Learn More About Testing
While we have touched on testing in this course, you can delve deeper into:
- Integration and unit tests: Write more comprehensive tests using frameworks like Jest or Mocha.
- Type tests: Ensure that the type definitions in your libraries and APIs are accurate.
Final Reflection
TypeScript is not just a typing tool but an ecosystem that helps you create safer, scalable, and easier-to-maintain software. With an active and constantly growing community, TypeScript will continue to be an integral part of modern JavaScript development.
We encourage you to keep experimenting and improving your skills with TypeScript. Every project you build will be an opportunity to hone your knowledge and discover new ways to write cleaner and more efficient code.
What's Next?
Now that you have completed this course, here are some resources and areas you might explore to continue advancing:
- Official TypeScript documentation: The official documentation is always a great reference to delve into advanced features.
- Community and forums: Join online communities, such as Stack Overflow, where you can ask questions and share your experience with other developers.
- Conferences and workshops: Participate in conferences and workshops where practical cases are explored, and the latest TypeScript developments are presented.
Congratulations on completing the course, and we hope you continue using TypeScript in all your future projects!
You can also deepen in the following topics:
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
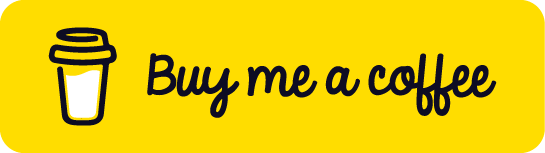
Chat with Chuck
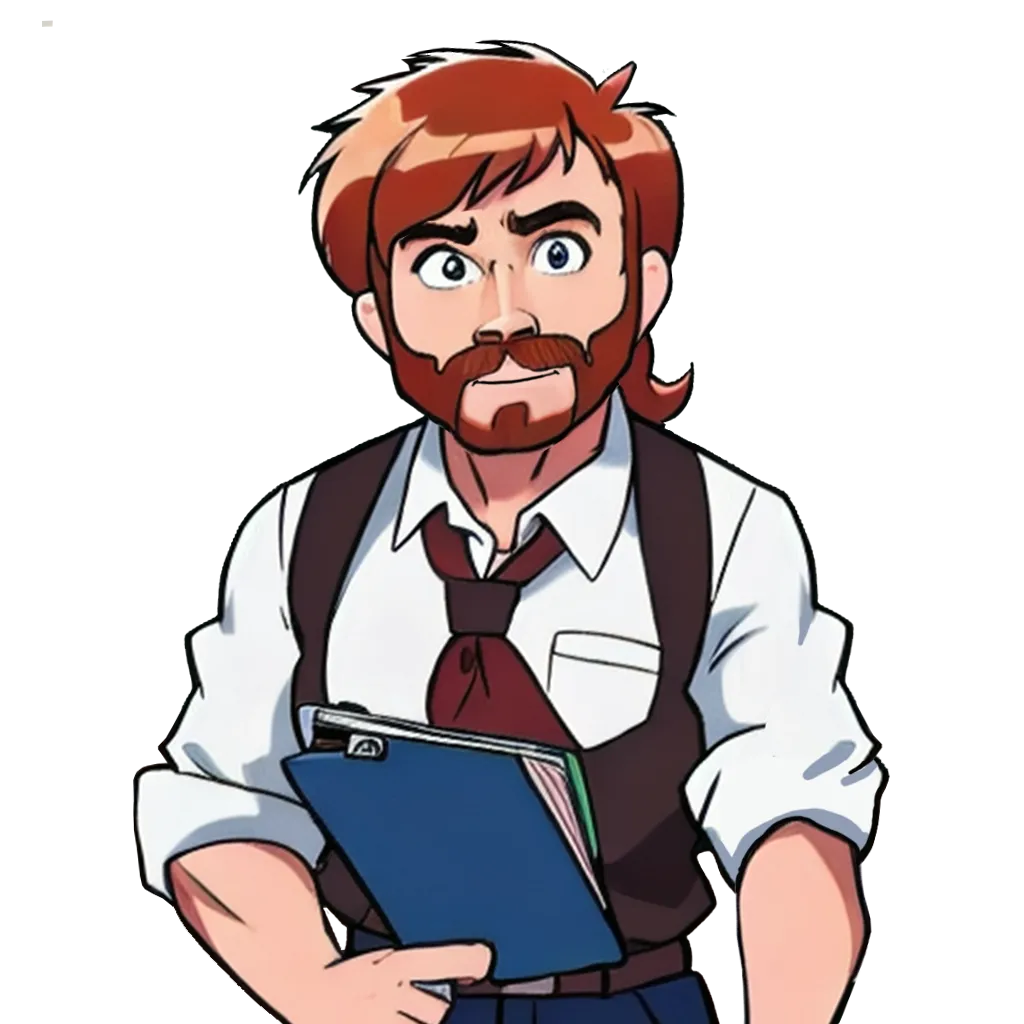
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps