Basic TypeScript
Decorators in TypeScript
Decorators in TypeScript are a powerful feature that allows you to modify the behavior of classes, methods, properties, and parameters in a flexible and reusable way. This feature is inspired by languages like Python and is widely used in frameworks like Angular.
This image shows a logo of TypeScript decorators
Introduction to Decorators
A decorator is a function that applies to a class, method, property, or parameter and can modify its behavior. To use decorators, you need to enable this feature in the tsconfig.json
file:
json
Syntax of a Decorator
A decorator is simply a function that receives the element it is decorating as an argument. Let's look at a basic example:
typescript
Types of Decorators
Class Decorator
A class decorator applies to the definition of a class and can modify its behavior. The decorator receives the class constructor as an argument.
typescript
Method Decorator
Method decorators apply to class methods. They can modify the behavior of methods, as we saw in the previous example.
Property Decorator
A property decorator is used to modify the behavior of a property. This decorator cannot access the property's value; it can only modify its configuration.
typescript
Parameter Decorator
A parameter decorator applies to a method's parameters and can be used to inspect or modify the parameter values.
typescript
Decorators in Frameworks
Decorators are widely used in frameworks like Angular, where they are used to define components, services, and other parts of the application. For example, in Angular, the @Component
decorator is used to define a component:
typescript
Conclusion
In this chapter, we have learned what decorators are in TypeScript and how to use them to modify the behavior of classes, methods, properties, and parameters. Decorators are a powerful tool widely used in frameworks like Angular to structure complex applications in a clean and efficient manner.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
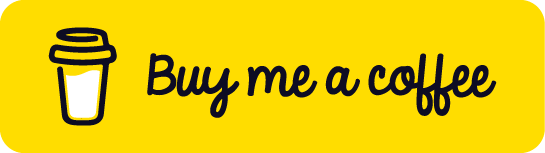
Chat with Chuck
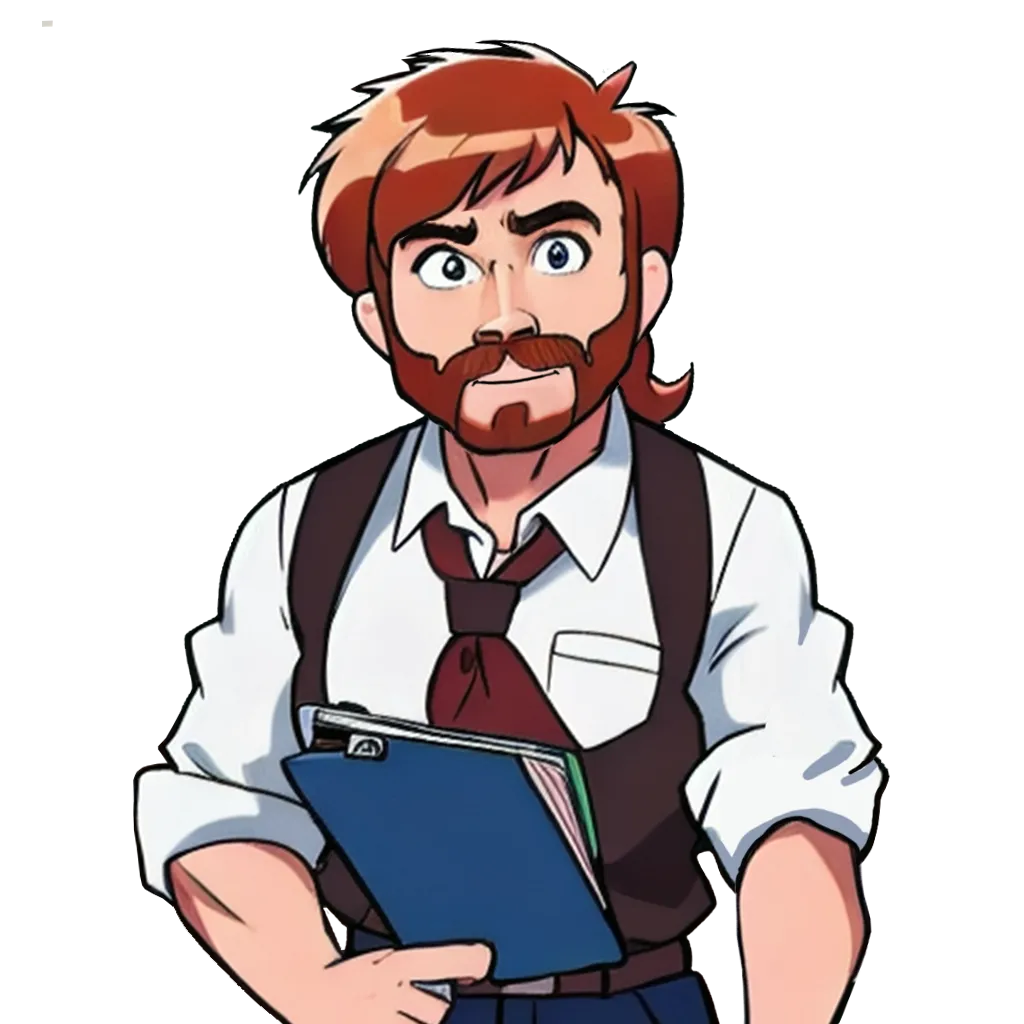
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps