Basic TypeScript
Introduction to TypeScript
TypeScript is a superset of JavaScript that adds optional static typing to the language. It was created by Microsoft with the aim of making large-scale JavaScript application development easier and less error-prone. Like JavaScript, TypeScript runs on any browser or platform that supports JavaScript.
This image shows the TypeScript logo
TypeScript allows developers to catch errors at development time, before the code is executed. This facilitates writing safer and more maintainable code, which is especially important in large projects and development teams.
This image shows a comparison between untyped JavaScript code and typed TypeScript code, highlighting the differences in error detection
Advantages of TypeScript
Some of the main advantages of using TypeScript include:
- Static Typing: Allows defining data types for variables, function parameters, and return values, which helps prevent type errors.
- IDE Enhancements: Text editors like Visual Studio Code offer autocompletion, refactoring, and real-time error detection, thanks to TypeScript.
- JavaScript Compatibility: TypeScript is 100% compatible with JavaScript, meaning any
.js
file is valid TypeScript code. - Compile-Time Error Checking: By compiling TypeScript code to JavaScript, errors can be detected before execution.
typescript
Installing TypeScript
To start using TypeScript, it needs to be installed. One of the most common ways is to use Node.js package manager, npm. Here is how to install TypeScript globally:
bash
Once TypeScript is installed, you can verify the installed version using the following command:
bash
First Program in TypeScript
Let's see a basic example of a TypeScript program. This program will print a greeting to the console:
typescript
To compile this program, the TypeScript compiler, tsc
, is used, which converts the .ts
file into a JavaScript file that can be run in the browser or Node.js:
bash
[Placeholder for image: This image shows the process of compiling a TypeScript file to JavaScript using the tsc
command.]
Conclusion
In this chapter, we have explored what TypeScript is, its advantages, how to install it, and created our first basic program. In the next chapters, we will delve into data types in TypeScript and how to make the most of its capabilities to write more robust and maintainable code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
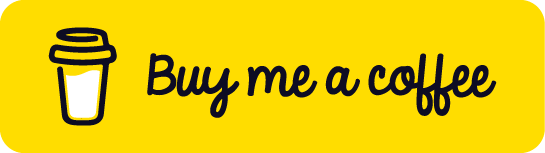
Chat with Chuck
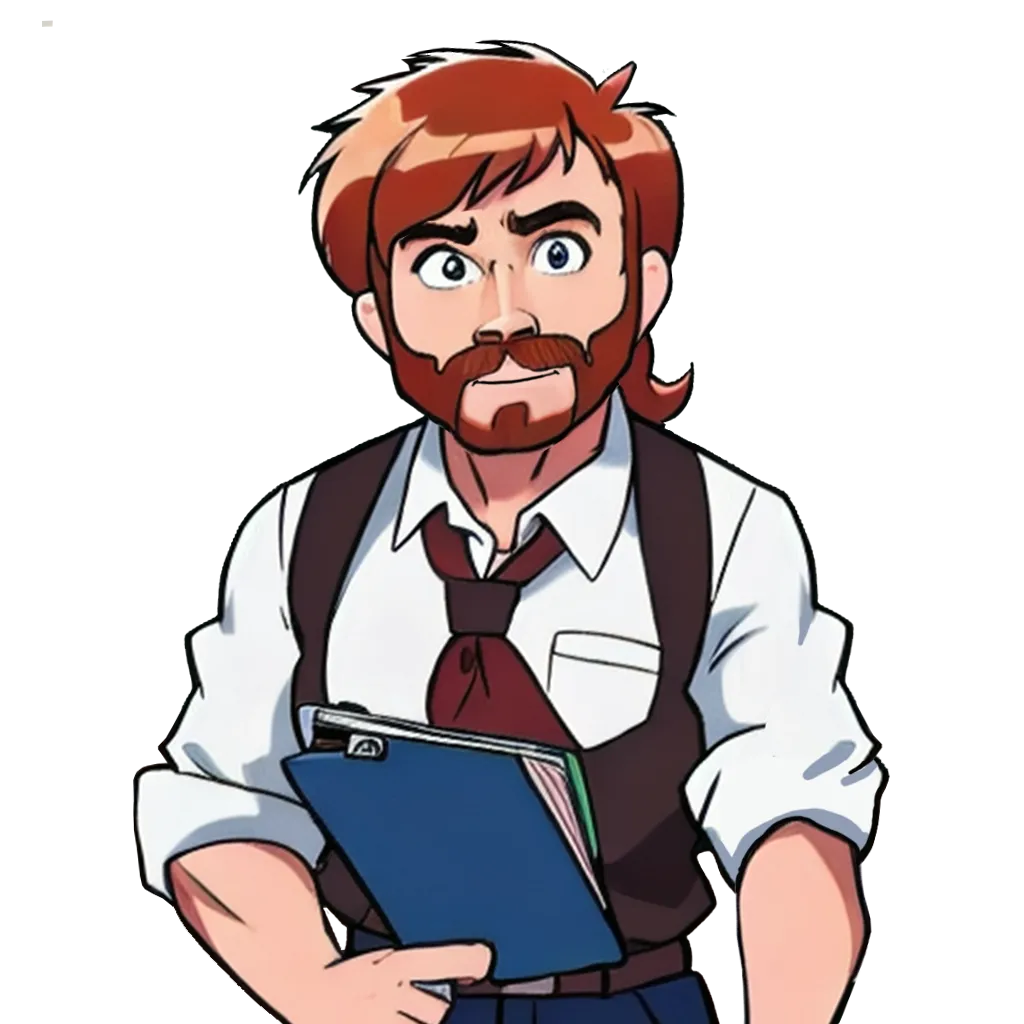
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps