Basic TypeScript
Strict and Optional Typing in TypeScript
Strict typing is a key feature in TypeScript that ensures compliance with the typing rules defined by the developer, helping to prevent errors. In this chapter, we will delve into how strict and optional typing works in TypeScript, and how they can improve code robustness.
Strict Typing
Strict typing mode is a configuration option that you can enable in the tsconfig.json
file. When enabled, it enforces stricter rules regarding the use of types. For example, it does not allow the use of null
or undefined
as values for types that do not expect them, nor the use of uninitialized variables.
To enable strict typing, the following option must be added or modified in the tsconfig.json
file:
json
Strict typing also includes other configurations, such as:
- noImplicitAny: Prevents the use of implicit
any
, requiring the explicit declaration of a variable's type. - strictNullChecks: Does not allow assigning
null
orundefined
to variables that do not expect them.
typescript
Optional Variables
In TypeScript, it is possible to define function parameters or object properties as optional. This means that it is not mandatory to provide a value for these variables. To do so, the ?
symbol is used after the parameter or property name.
Optional Parameters
When a parameter is optional, the value may or may not be provided when the function is called. If not provided, its value will be undefined
.
typescript
Optional Properties
Object properties can also be optional, allowing some values to be absent when creating instances of a type.
typescript
Type Assertions
Type assertions allow the developer to explicitly indicate to TypeScript that a variable has a specific type. This is useful when working with values whose type may be ambiguous or cannot be correctly inferred.
Type Assertion Syntax
There are two ways to make a type assertion in TypeScript:
-
Using the
as
keyword:typescript"Here, someValue is of type any, but we are asserting that it is a string, so we can access the length property." -
Using angle bracket syntax:
typescript"This example uses the same type assertion as the previous one, but with angle bracket syntax. Both forms are equivalent."
Conclusion
Strict and optional typing in TypeScript is essential for writing safer and clearer code. Through strict type rules and the use of optional parameters and type assertions, you can avoid common errors and have greater control over the data you handle.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
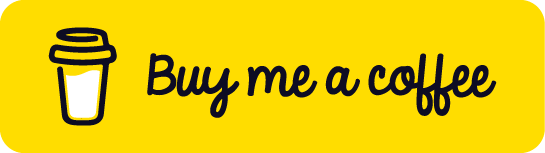
Chat with Chuck
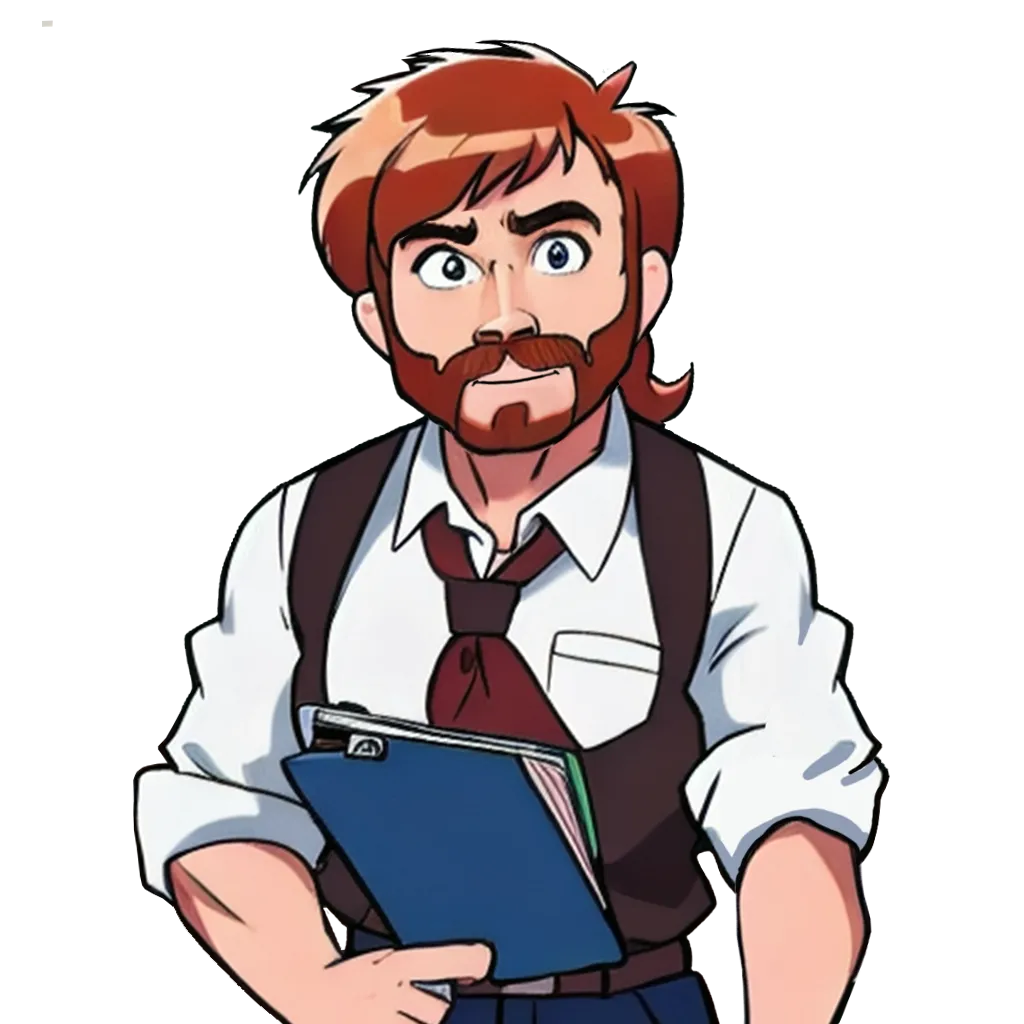
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps