Basic TypeScript
Error Handling in TypeScript
Proper error handling is essential for building robust and secure applications. TypeScript, being a superset of JavaScript, inherits JavaScript's error handling capabilities but adds stricter typing that helps us manage errors in a more controlled way.
this image shows a cover for error handling
Types of Errors
In TypeScript, we can handle different types of errors, including:
- Compile-time Errors: These errors are detected by the TypeScript compiler and prevent incorrect code from being executed. For example, typing errors.
- Runtime Errors: These errors occur during code execution and are handled using
try
andcatch
blocks.
Compile-time Error Example
typescript
Runtime Error Handling
Runtime errors can be caught using try
, catch
, and finally
blocks. This allows us to handle errors in a more controlled way, ensuring that our program can recover or properly handle exceptions.
try
and catch
Example
typescript
finally
Block
The finally
block always executes, regardless of whether an error occurred. This is useful when we need to execute code that must run no matter what, like releasing resources or closing connections.
typescript
Error Typing
One of the advantages of TypeScript is that we can define types for the errors we want to handle, making the code more predictable and secure.
Custom Error Typing
We can create our own error types to handle them more specifically in our applications.
typescript
Asynchronous Errors
When working with asynchronous operations, such as API calls, it is also important to handle errors properly. Promises and asynchronous functions provide mechanisms to catch errors.
Error Handling with Promises
typescript
Error Handling with async
and await
Asynchronous functions with async
and await
allow us to write more readable code and handle errors similarly to synchronous functions.
typescript
Conclusion
In this chapter, we have learned how to handle errors in TypeScript, both at compile-time and runtime. We have also seen how to type custom errors and handle asynchronous errors, allowing us to build more robust and secure applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
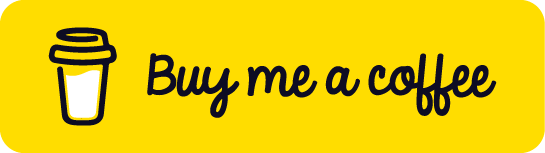
Chat with Chuck
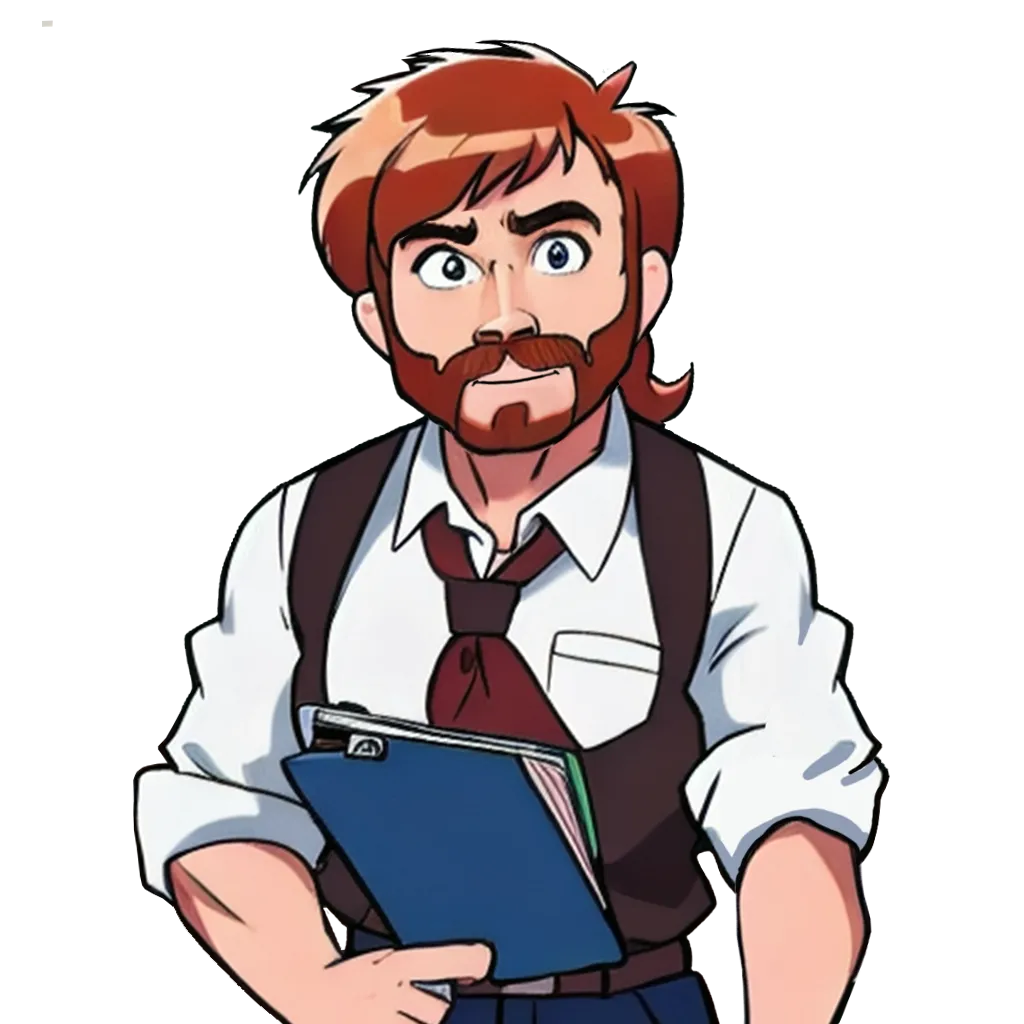
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps