Basic TypeScript
TypeScript Project Setup
One of the benefits of using TypeScript is its flexibility when configuring projects. This allows us to customize the compiler's behavior and optimize the development process. The key to this configuration is the tsconfig.json
file, which defines the compiler options and other project settings.
The tsconfig.json
File
The tsconfig.json
file is the TypeScript configuration file that defines how the code is compiled. You can generate this file automatically using the following command:
bash
This image shows a screenshot using tsconfig.json
Basic Structure of tsconfig.json
A basic tsconfig.json
file has the following structure:
json
Common Options in compilerOptions
Below, we will look at some of the most commonly used options in the tsconfig.json
file.
target
The target
option defines to which ECMAScript version the TypeScript code should be transpiled. Some common values are:
"es5"
: Compatible with most browsers."es6"
: Allows the use of ECMAScript 6 features, such aslet
andconst
.
json
module
The module
option specifies the module system to be used. Some common values are:
"commonjs"
: Module system used in Node.js."esnext"
: Uses the native ECMAScript module system.
json
strict
The strict
option enables TypeScript's strict mode, which includes several safety configurations, such as noImplicitAny
and strictNullChecks
. This helps detect more errors during development time.
json
esModuleInterop
This option facilitates interoperability between ECMAScript and CommonJS modules, which is useful when working with JavaScript libraries that use different module systems.
json
Including and Excluding Files
TypeScript allows including and excluding files in the compilation using the include
and exclude
options. This gives us control over which files and folders will be processed by the compiler.
Inclusion Example
json
Exclusion Example
json
Configuration for Development and Production
TypeScript allows configuring different options for development and production. A common strategy is to use different configuration files or override some options for each environment.
Development Configuration
json
Production Configuration
json
Conclusion
In this chapter, we have learned how to configure TypeScript projects using the tsconfig.json
file. This configuration allows us to customize the compiler's behavior, optimizing our workflow for different environments, such as development and production.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
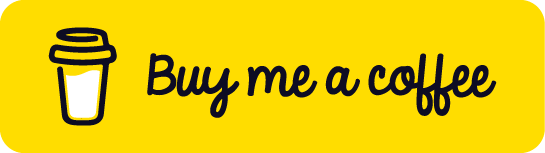
Chat with Chuck
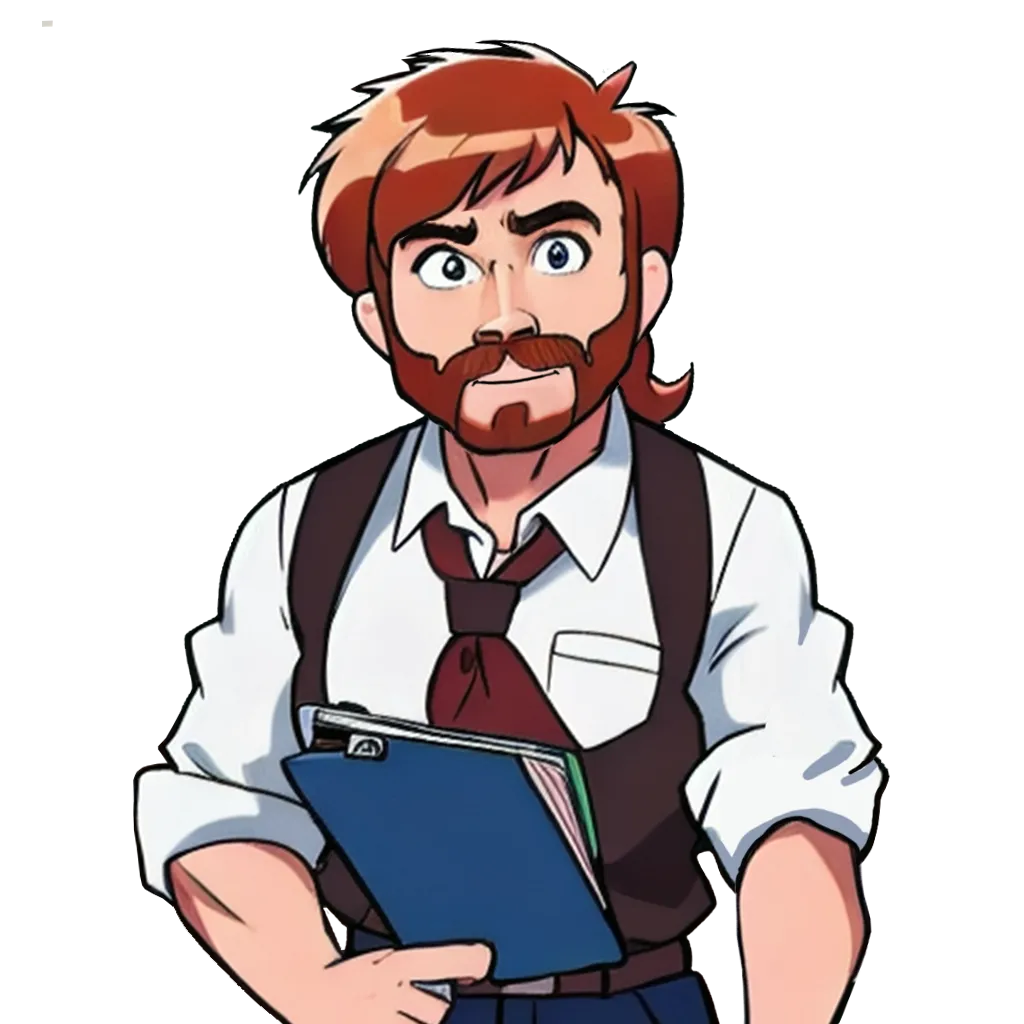
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps