Basic TypeScript
Webpack and TypeScript Configuration
Webpack is one of the most popular tools for bundling modern JavaScript applications. It allows you to bundle all the modules and files of your project into a single optimized output file. In this chapter, we will learn how to configure Webpack for TypeScript projects, which will help us manage our dependencies and optimize code for production.
This image shows a webpack logo
Installing Webpack and TypeScript
First, we need to install Webpack and TypeScript along with some additional tools to integrate both. Run the following command to install the necessary dependencies:
bash
Installing Webpack
webpack
: The core of Webpack.webpack-cli
: The command line interface for Webpack.ts-loader
: A Webpack loader that allows processing TypeScript files.
Basic Webpack Configuration
To configure Webpack in a TypeScript project, we need to create a webpack.config.js
file that defines how Webpack should behave when processing our files.
Basic webpack.config.js
File
javascript
File Explanation
- entry: Defines the main file that Webpack should process, in this case
index.ts
. - output: Defines the output file (
bundle.js
) and the folder where it will be saved (dist
). - resolve: Allows Webpack to resolve extensions like
.ts
and.js
. - module.rules: Here, the
ts-loader
is configured to process all files with the.ts
extension.
TypeScript Configuration with Webpack
Next, we need a tsconfig.json
file to define how TypeScript should compile our code.
tsconfig.json
File
json
Compiling with Webpack
Once you have configured webpack.config.js
and tsconfig.json
, you can run the following command to compile the project using Webpack:
bash
Production Optimization
Webpack offers several tools to optimize code in production environments, such as minification and dead code elimination.
Configuration for Production
In Webpack, you can define different configurations for development and production. A common way to do this is to use the production
mode and add optimizations like code minification.
javascript
Integrating Plugins in Webpack
Besides loaders, Webpack also allows the use of plugins to extend its functionality. Some useful plugins for TypeScript projects include CleanWebpackPlugin
to clean the output folder before each build, and HtmlWebpackPlugin
to automatically generate an HTML file that includes the bundle.
Example with Plugins
bash
javascript
Conclusion
In this chapter, we learned how to set up Webpack in a TypeScript project, from basic installation to production optimization. We also saw how to use plugins to extend Webpack's functionality. These tools allow us to better manage our projects and optimize the code to be more efficient in production environments.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
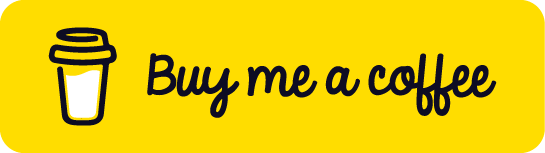
Chat with Chuck
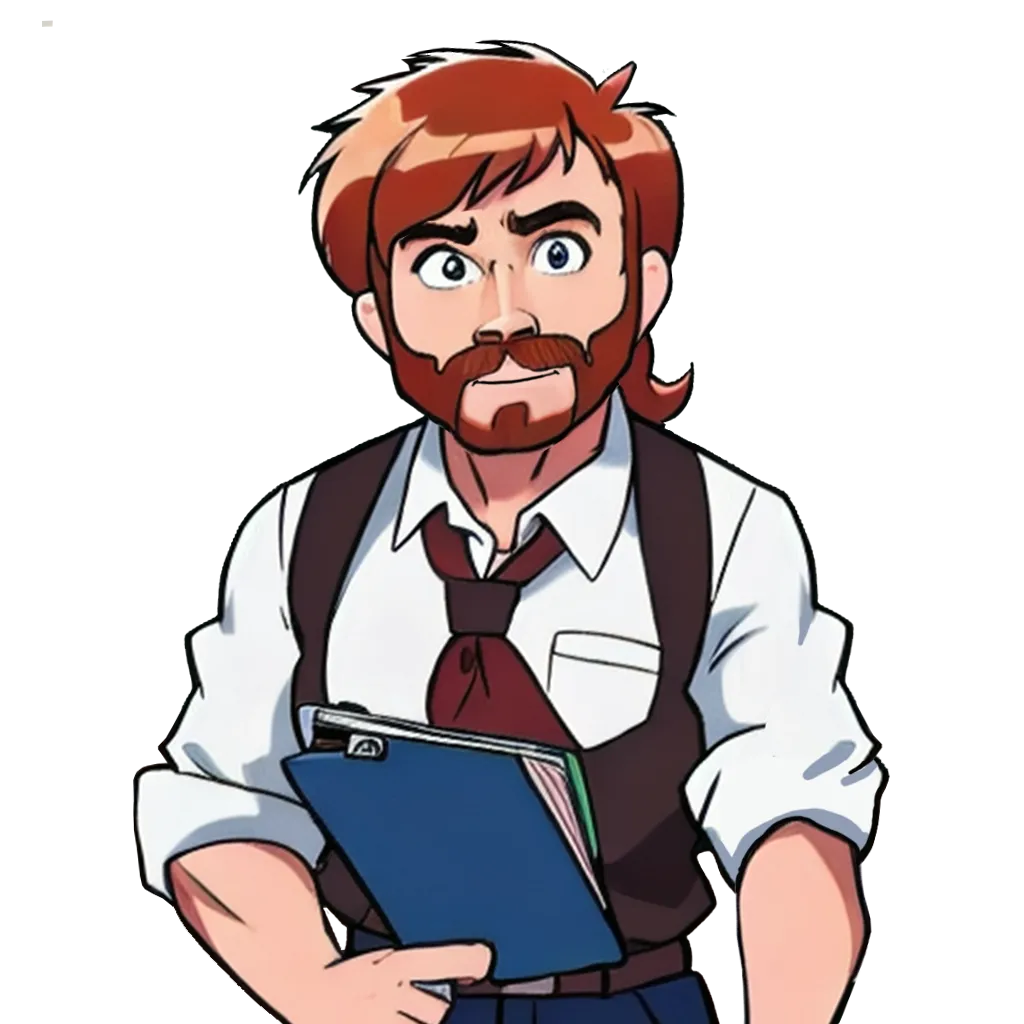
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps