Basic TypeScript
Data Types in TypeScript
One of the most powerful aspects of TypeScript is its type system, which allows you to define the data types that variables, functions, and other code elements must handle. Below, we will explore the basic and advanced data types that you can utilize to make your code more robust and predictable.
Primitive Types
TypeScript includes all the primitive data types found in JavaScript. These are:
- number: for numbers, both integers and decimals.
- string: for text.
- boolean: for boolean values (true or false).
- null and undefined: to represent the absence of value.
Here is an example of how to declare variables with primitive types:
typescript
Esta imagen muestra distintos data types
Complex Types
In addition to primitive types, TypeScript offers various complex data types that allow for more sophisticated data structures:
- Arrays: Arrays in TypeScript can be defined with homogeneous types.
- Tuples: Tuples are arrays with a fixed number of elements, each having a specific type.
- Enums: Enums allow you to define a set of named constants.
Arrays
To declare an array in TypeScript, you can use the type[]
or Array<type>
notation:
typescript
Tuples
Tuples allow you to declare an array with a specific number and types of values:
typescript
Enums
Enums in TypeScript are used to declare a set of clearly-named constants:
typescript
Custom Types: Alias and Union
TypeScript allows you to create custom types using aliases and union types. This facilitates code readability and maintenance.
Type Aliases
Type aliases let you create a name for a complex type, simplifying its use in various parts of the code:
typescript
Union Types
Union types allow a variable to have more than one type:
typescript
Conclusion
In this chapter, we have explored the data types in TypeScript, from primitive types to complex types like arrays, tuples, and enums, as well as the creation of aliases and union types. These concepts are essential to leverage TypeScript's type system and write clearer and safer code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
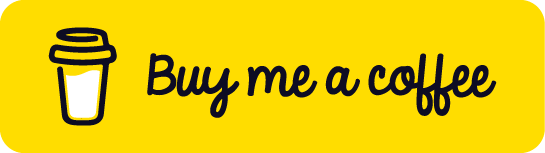
Chat with Chuck
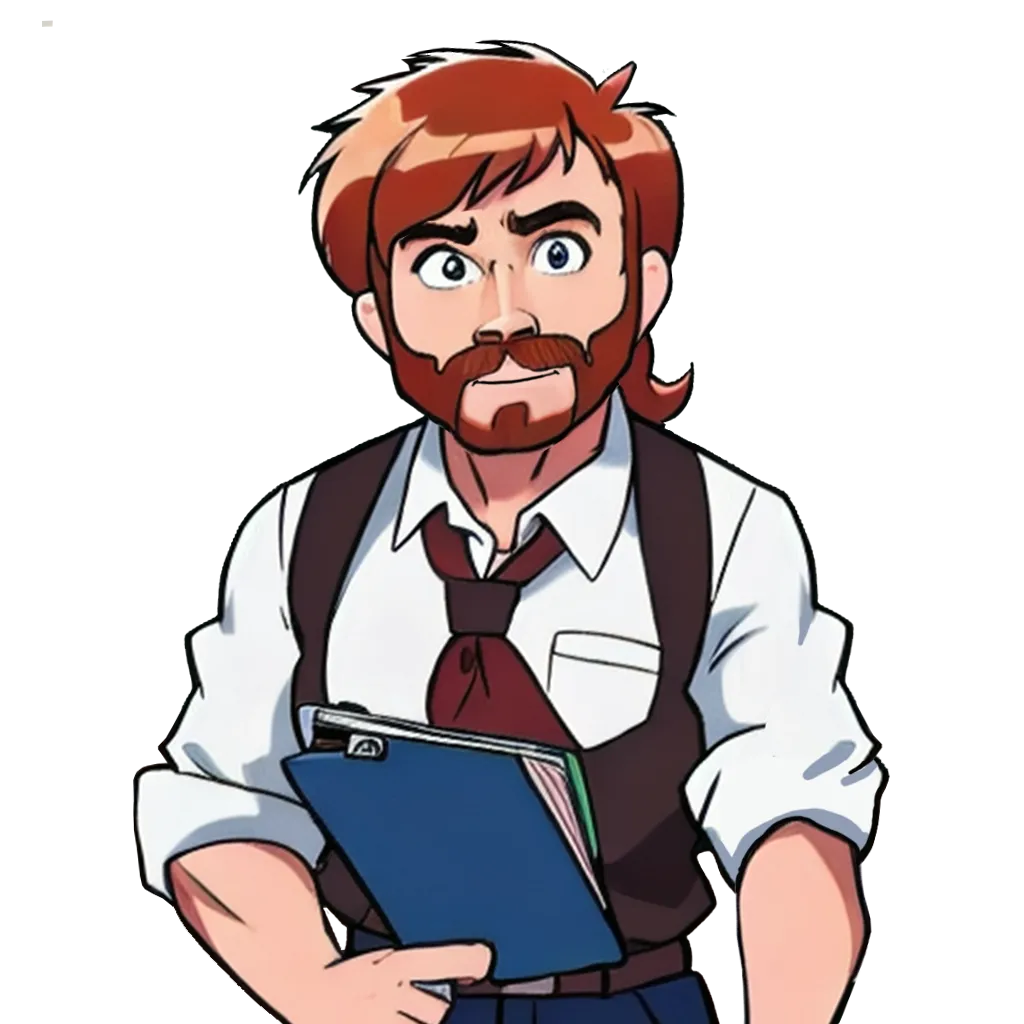
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps