Basic TypeScript
Migration from JavaScript to TypeScript
Migrating a JavaScript project to TypeScript is an excellent way to take advantage of static typing, error checking during development, and autocompletion. In this chapter, we will explore strategies and key steps for migrating an existing JavaScript project to TypeScript without disrupting the workflow.
This image shows a cartoon about migrating from JavaScript to TypeScript
Benefits of Migrating to TypeScript
Before diving into the migration process, it is important to understand the benefits of using TypeScript:
- Type Checking: TypeScript allows you to catch errors before the code is run, significantly reducing errors in production.
- Autocompletion: Enhances productivity by providing autocompletion and suggestions based on defined types.
- Compatibility: TypeScript is fully compatible with JavaScript, allowing for gradual migration.
Migration Strategies
There are several strategies for migrating a project to TypeScript, depending on the size and complexity of the project. Here are some of the most common:
Progressive Migration
Progressive migration is one of the safest and most recommended strategies for large projects. It involves converting one or several files to TypeScript at a time, allowing JavaScript and TypeScript to coexist in the project.
Complete Migration
For smaller projects or when time permits, a complete migration can be chosen, where all files are converted to TypeScript at once.
Step 1: Project Configuration
The first step in any migration process is to configure TypeScript in the existing project. Install TypeScript and create a tsconfig.json
file.
Installing TypeScript
bash
Generating tsconfig.json
bash
The tsconfig.json
file should be configured to include JavaScript files during the migration.
json
Step 2: Rename Files to .ts
The next step is to rename .js
files to .ts
. This tells TypeScript that these files now need to be processed and checked by the compiler.
bash
At this stage, JavaScript files will continue to work, but TypeScript will start type-checking them. The .ts
files will receive full type-checking.
Step 3: Gradually Add Typing
Once the files have been renamed to .ts
, you can start adding types progressively. TypeScript is flexible, so not everything needs to be typed right away.
Variable Typing
typescript
Function Typing
typescript
Step 4: Install Type Definitions
Many JavaScript libraries do not include type definitions by default. To leverage typing for external libraries, install type definitions from the DefinitelyTyped repository.
Example of Type Installation
bash
Step 5: Review and Refactoring
During migration, it is important to review and refactor the code to ensure you fully leverage TypeScript's advantages. Some common practices include:
- Avoiding
any
: Use more specific types instead ofany
to maintain type safety. - Adding Interfaces: Use interfaces to clearly define object structures.
- Refactoring Functions: Leverage TypeScript features to refactor functions and improve readability.
Refactoring Example
Before TypeScript:
javascript
After TypeScript:
typescript
Step 6: Update the Build Process
Finally, ensure that your project's build process is configured to compile TypeScript. If you use tools like Webpack, you'll need to configure specific loaders for TypeScript.
Webpack Configuration
bash
javascript
Conclusion
Migrating a JavaScript project to TypeScript is a process that can be done gradually, allowing both languages to coexist while adopting static typing. In this chapter, we explored the key steps to carry out a successful migration, from initial setup to refactoring and adjusting the build process.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
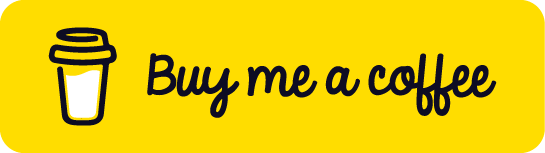
Chat with Chuck
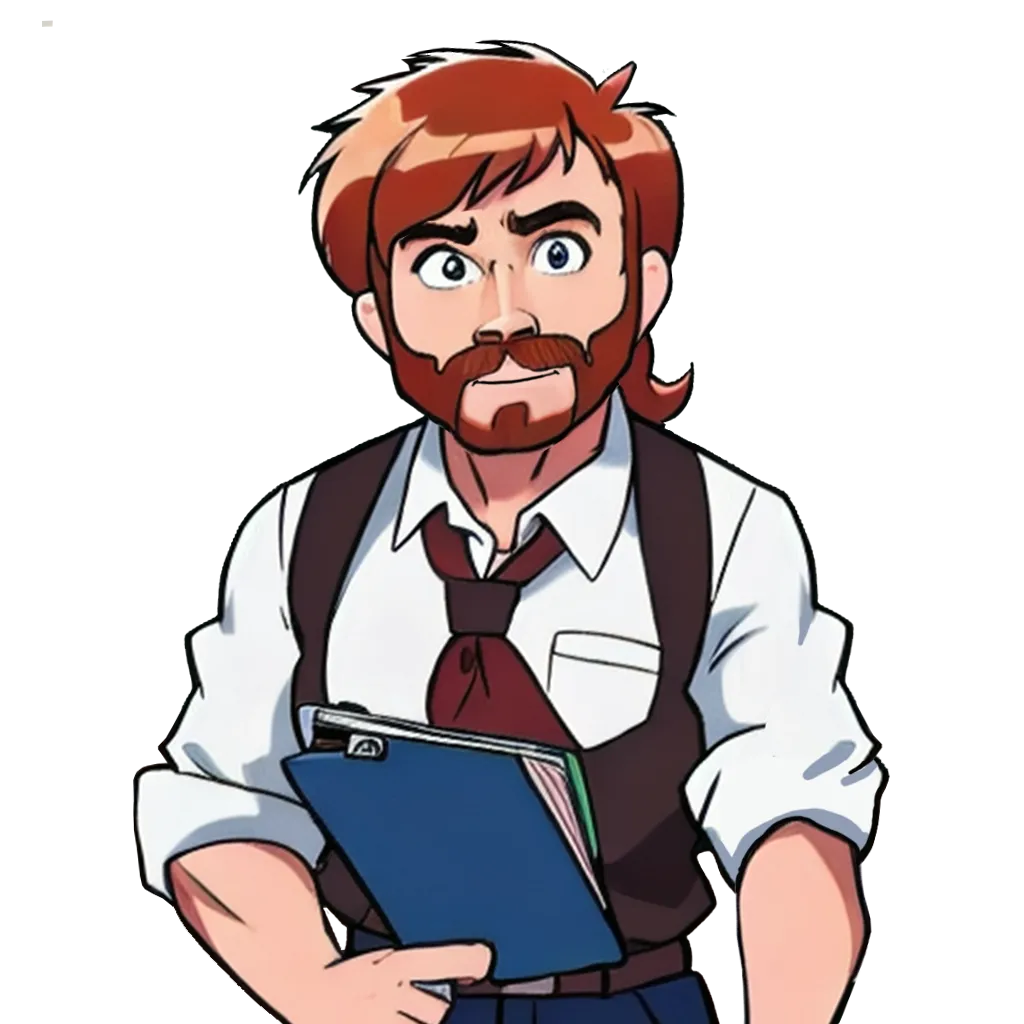
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps