Basic TypeScript
Modularization and Dependency Management in TypeScript
In large projects, it is crucial to divide code into smaller modules and manage them efficiently. In this chapter, we will learn how to modularize a TypeScript project, manage dependencies, and work with external modules using npm and other dependency managers.
Modularization in TypeScript
TypeScript uses the same module system as ECMAScript 6 (ES6). Modules allow you to divide code into different files and organize it in a clearer and more maintainable way.
Module Export and Import
To create a module, simply export the functions, classes, or variables you want to share from a file. Then, import those elements into other files where they are needed.
This image shows a diagram with module import
Exported Module Example
typescript
Imported Module Example
typescript
Dependency Management with npm
npm is the most popular package manager for JavaScript and TypeScript projects. It allows you to easily install, update, and manage external dependencies.
Installing Dependencies
To install a library or external dependency, use the npm install
command followed by the library’s name.
bash
Using External Dependencies in TypeScript
Once the dependency is installed, we can use it in our project. If the dependency does not include type definitions, we can also install the type definitions separately.
bash
Example of Using an External Dependency
typescript
Dependency Versioning
npm allows you to control the versions of the dependencies installed in the project. This is managed through the package.json
file, which holds specific versions of each package.
json
Managing Dependencies in Large Projects
In large projects, managing dependencies can become complex. Some recommended practices include:
- Keep dependencies updated: Make sure to regularly update dependencies to include security fixes and new features.
- Use a lock file:
package-lock.json
oryarn.lock
files ensure specific versions of dependencies are installed, avoiding differences across environments.
Updating Dependencies
To update dependencies to their latest compatible versions, you can use the following command:
bash
Auditing Dependencies
npm also includes an audit tool that checks dependencies for security vulnerabilities.
bash
Using Other Dependency Managers
While npm is the most popular dependency manager, there are other alternatives like Yarn or pnpm that offer performance improvements or additional features.
Installing and Using Yarn
Yarn is an alternative dependency manager focused on speed and consistency between environments. You can install Yarn using npm:
bash
Installing Dependencies with Yarn
bash
Conclusion
In this chapter, we have learned how to modularize TypeScript projects and manage dependencies using npm and other tools. Efficient modularization and dependency management are essential for maintaining large and scalable projects.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
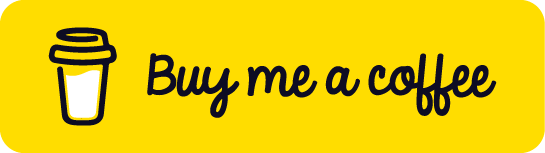
Chat with Chuck
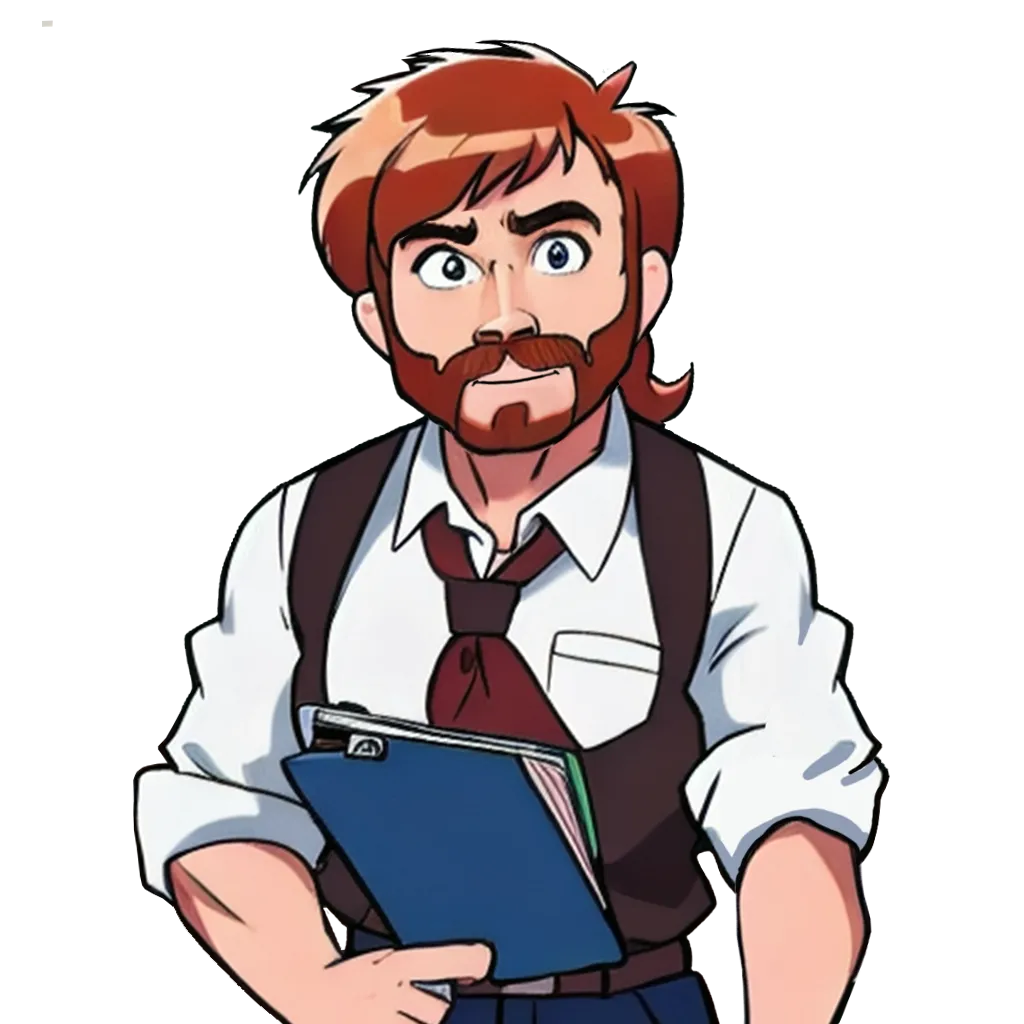
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps